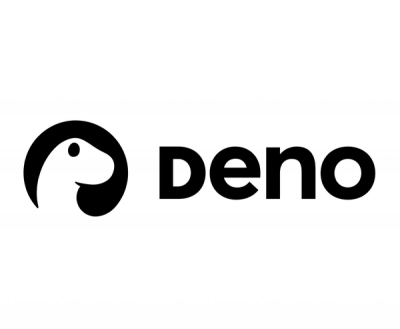
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
vue-cryptojs
Advanced tools
A small wrapper for integrating crypto-js into Vue3 and Vue2
npm install vue-cryptojs
And in your entry file:
// Vue3
import { createApp } from 'vue'
import VueCryptojs from 'vue-cryptojs'
const app = createApp(...)
app.use(VueCryptojs)
app.mount('#app')
// Vue2
import Vue from 'vue'
import VueCryptojs from 'vue-cryptojs'
Vue.use(VueCryptojs)
This wrapper bind CryptoJS
to Vue
or this
if you're using single file component.
Simple AES text encrypt/decrypt example:
const encryptedText = this.$CryptoJS.AES.encrypt("Hi There!", "Secret Passphrase").toString()
const decryptedText = this.$CryptoJS.AES.decrypt(encryptedText, "Secret Passphrase").toString(this.CryptoJS.enc.Utf8)
For Vue3 we suggest to use inject
on Composition API:
<script>
import { inject } from 'vue'
export default {
setup() {
const cryoptojs = inject('cryptojs')
return {
cryoptojs
}
}
}
</script>
Please kindly check full documention of crypto-js
FAQs
A small wrapper for integrating crypto-js into VueJS
The npm package vue-cryptojs receives a total of 2,511 weekly downloads. As such, vue-cryptojs popularity was classified as popular.
We found that vue-cryptojs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.