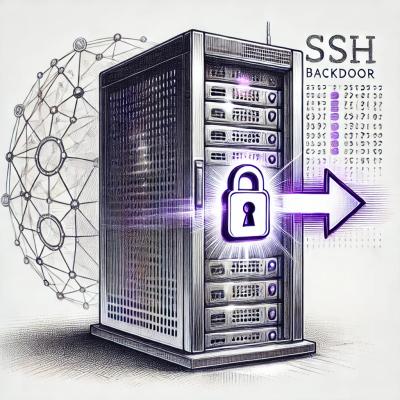
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
vue-easter-egg-trigger
Advanced tools
This packages makes it nice and easy to add Easter Egg triggers to your Vue site.
The vue-easter-egg-trigger
component makes it nice and easy to add Easter Egg triggers to your Vue site. Also available for Vue 3 at vue3-easter-egg-trigger.
pnpm add vue-easter-egg-trigger
npm i vue-easter-egg-trigger
import Vue from 'vue';
import EasterEggTrigger from 'vue-easter-egg-trigger';
Vue.use(EasterEggTrigger);
import { EasterEggTrigger } from 'vue-easter-egg-trigger';
See it in action on the Demo Page
Name | Type | Default | Description |
---|---|---|---|
delay | Integer | 500 | Determines the timeout before the event lister resets. |
import Vue from 'vue';
import EasterEggTrigger from 'vue-easter-egg-trigger';
Vue.use(EasterEggTrigger, {
delay: 1000,
});
Name | Type | Default | Description |
---|---|---|---|
callback | Function | null | The callback function. |
destroyBus | Boolean | true | Determines if a bus $on event is destroyed automatically. |
name | String | easter-egg | Identifier & used for even bus callback. |
pattern | Array | ['ArrowUp', 'ArrowUp', 'ArrowDown', 'ArrowDown', 'ArrowLeft', 'ArrowRight', 'ArrowLeft', 'ArrowRight', 'b', 'a'] | The key/click combination a user does to trigger easter egg. The default combination is the konami code. |
target | String | div | Use this to target DOM elements, Id's, or Class Names. Used with click events. |
triggered | Function | null | Same functionality as the callback option. |
type | String | keydown | The type of action the trigger will be listening for. |
withBus | Boolean | false | Determines if a bus event is emitted. |
Name | Response | Description |
---|---|---|
callback | $event | The callback event handler. If you use $event it will return the easter egg options object. |
triggered | $event | Same functionality as the callback event handler. |
<EasterEggComponent
@callback="callbackEvent($event)"
/>
When using the component you will setup VueEasterEggTrigger
using the Component Registration.
Instead of using :callback
and :triggered
as an option you can use the @callback
and @triggered
event handlers.
The default key combination to trigger the easter egg is the Konami Code.
<template>
<EasterEggComponent
type="click"
@callback="callbackEvent('using-component')"
/>
</template>
<EasterEggComponent
:withBus"true"
type="keydown"
@callback="callbackEvent('using-component')"
/>
<script>
this.$bus.$on('easter-egg', () => {
// also do something...
});
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="keydown"
@callback="callbackEvent('using-component')"
/>
<script>
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="keydown"
@triggered="triggeredEvent('using-component')"
/>
<script>
export default {
methods: {
triggeredEvent(name) {
// do something ...
},
},
};
</script>
First you will need to set the type in the plugin options.
Available types of Mouse Events: click
, dblclick
, mouseup
, mousedown
.
When using dblclick
the pattern will only work with one double click. Ex. pattern: ['dblclick']
<EasterEggComponent
type="click"
@callback="callbackEvent('using-component')"
/>
<script>
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="click"
@callback="callbackEvent('using-component')"
/>
<script>
this.$bus.$on('easter-egg', () => {
// also do something...
});
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="click"
target="h1"
@callback="callbackEvent('using-component')"
/>
<script>
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="click"
target="#foo"
pattern="['click', 'click']"
@callback="callbackEvent('using-component')"
/>
<script>
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
<EasterEggComponent
type="click"
target=".foo"
pattern="['click', 'click', 'click']"
@callback="callbackEvent('using-component')"
/>
<script>
export default {
methods: {
callbackEvent(name) {
// do something ...
},
},
};
</script>
There are two instance methods available to use.
$easterEgg
$easterEggTrigger
When using an instance method you will setup VueEasterEggTrigger
using the Plugin Registration.
The default key combination to trigger the easter egg is the Konami Code.
this.$bus.$on('easter-egg', () => {
// do something...
});
this.$easterEgg({
withBus: true,
});
this.$bus.$on('easter-egg', () => {
// also do something...
});
this.$easterEgg({
name: 'easter-egg',
callback() {
// do something ...
},
withBus: true,
});
this.$easterEgg({
name: 'easter-egg',
callback() {
// do something ...
},
});
this.$easterEgg({
name: 'easter-egg',
triggered() {
// do something ...
},
});
First you will need to set the type in the plugin options.
Available types of Mouse Events: click
, dblclick
, mouseup
, mousedown
.
When using dblclick
the pattern will only work with one double click. Ex. pattern: ['dblclick']
import Vue from 'vue';
import EasterEggTrigger from 'vue-easter-egg-trigger';
Vue.use(EasterEggTrigger, {
type: 'click',
callback() {
// do something ...
},
});
this.$bus.$on('easter-egg', () => {
// also do something...
});
this.$easterEgg({
name: 'easter-egg',
pattern: ['click', 'click'],
callback() {
// do something...
},
withBus: true,
});
this.$easterEgg({
name: 'easter-egg',
pattern: ['click', 'click'],
target: 'h1',
callback() {
// do something ...
},
});
this.$easterEgg({
name: 'easter-egg',
pattern: ['click', 'click'],
target: '#foo',
callback() {
// do something ...
},
});
this.$easterEgg({
name: 'easter-egg',
pattern: ['click', 'click'],
target: '.foo',
callback() {
// do something ...
},
});
You can find a real world example on how to use the plugin in the HelloWorld.vue file.
Copyright (c) 2022 WebDevNerdStuff Licensed under the MIT license.
FAQs
This packages makes it nice and easy to add Easter Egg triggers to your Vue site.
The npm package vue-easter-egg-trigger receives a total of 3 weekly downloads. As such, vue-easter-egg-trigger popularity was classified as not popular.
We found that vue-easter-egg-trigger demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.