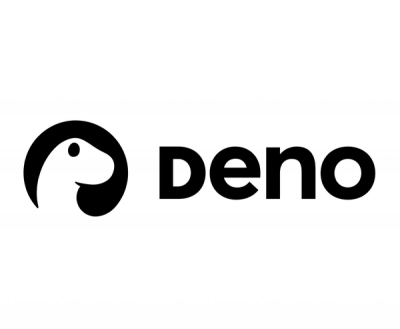
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Vue integration for the Laravel Echo library.
npm install vue-echo --save
First you'll need to register the plugin and optionally initialise the Echo instance.
import VueEcho from 'vue-echo';
Vue.use(VueEcho, {
broadcaster: 'pusher',
key: 'PUSHER KEY',
});
/**
* Alternatively you can pass an echo instance:
* ********************************************
* import Echo from 'laravel-echo';
*
* let EchoInstance = new Echo({
* broadcaster: 'pusher',
* key: 'PUSHER KEY',
* });
* Vue.use(VueEcho, EchoInstance);
*/
Once vue-echo is registered, every vue instance is able to to subscribe to channels and listen to events through the this.$echo
property on the connection you specified earlier.
You can subscribe a vue instance to a single standard channel if needed and define your events.
var vm = new Vue({
channel: 'blog'
echo: {
'BlogPostCreated': payload => {
console.log('blog post created', payload);
},
'BlogPostDeleted': payload => {
console.log('blog post deleted', payload);
}
}
})
Laravel echo allows you to subscribe to; normal, private and presence channels.
In the example above we subscribed to a standard channel.
If you would like to subscribe to private channel instead, prefix your channel name with private
:
var vm = new Vue({
channel: 'private:team.1'
echo: {
'BlogPostCreated': payload => {
console.log('blog post created', payload);
},
'BlogPostDeleted': payload => {
console.log('blog post deleted', payload);
}
}
})
If you would like to subscribe to presence channel instead, prefix your channel name with presence
:
var vm = new Vue({
channel: 'presence:team.1.chat'
echo: {
'NewMessage': payload => {
console.log('bNew message from team', payload);
}
}
})
FAQs
Vue integration for the Laravel Echo library.
The npm package vue-echo receives a total of 1,078 weekly downloads. As such, vue-echo popularity was classified as popular.
We found that vue-echo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.