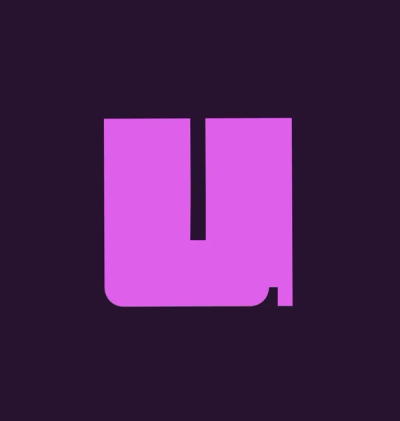
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Prop type definitions for Vue.js. Compatible with both Vue 1.x and 2.x
vue-types
is a collection of configurable prop type definitions for Vue.js components, inspired by React.PropTypes
.
While basic prop type definition in Vue is simple and convenient, detailed prop validation can become verbose on complex components.
This is the case for vue-types
.
Instead of:
export default {
props: {
id: {
type: Number,
default: 10
},
name: {
type: String,
required: true
},
age: {
type: Number,
validator(value) {
return Number.isInteger(value)
}
},
nationality: String
},
methods: {
// ...
}
};
You may write:
import VueTypes from 'vue-types';
export default {
props: {
id: VueTypes.number.def(10),
name: VueTypes.string.isRequired,
age: VueTypes.integer,
// No need for `default` or `required` key, so keep it simple
nationality: String
},
methods: {
// ...
}
}
npm install vue-types --save
Most native types come with a default value, a .def()
method to reassign the default value for the current prop and a isRequired
flag to set the required: true
key
const numProp = vueTypes.number
// numProp === { type: Number, default : 0}
const numPropCustom = vueTypes.number.def(10)
// numPropCustom === { type: Number, default : 10}
const numPropRequired = vueTypes.number.isRequired
// numPropCustom === { type: Number, required : true}
const numPropRequiredCustom = vueTypes.number.def(10).isRequired
// numPropRequiredCustom === { type: Number, default: 10, required : true}
VueTypes.any
Validates any type of value and has no default value.
VueTypes.array
Validates that a prop is an array primitive.
Array
VueTypes.bool
Validates boolean props.
true
VueTypes.func
Validates that a prop is a function.
VueTypes.number
Validates that a prop is a number.
0
VueTypes.integer
Validates that a prop is an integer (uses Number.isInteger
).
0
VueTypes.object
VueTypes.object
Validates that a prop is an object.
Object
VueTypes.string
VueTypes.string
Validates that a prop is a string.
''
Custom types have not default value, a .def()
method to assign a default value for the current prop and a isRequired
flag to set the required: true
key
const oneOfPropDefault = vueTypes.oneOf([0, 1]).def(1)
// oneOfPropCustom.default === 1
const oneOfPropRequired = vueTypes.oneOf([0, 1]).isRequired
// oneOfPropCustom.required === true
const oneOfPropRequiredCustom = vueTypes.oneOf([0, 1]).def(1).isRequired
// oneOfPropRequiredCustom.default === 1
// oneOfPropRequiredCustom.required === true
VueTypes.instanceOf()
class Person {
// ...
}
export default {
props: {
user: VueTypes.instanceOf(Person)
}
}
Validates that a prop is an instance of a JavaScript constructor. This uses JavaScript's instanceof
operator.
VueTypes.oneOf()
VueTypes.oneOf(arrayOfValues)
Validates that a prop is one of the provided values.
export default {
props: {
genre: VueTypes.oneOf(['action', 'thriller'])
}
}
VueTypes.oneOfType()
Validates that a prop is an object that could be one of many types. Accepts both simple and vue-types
types.
export default {
props: {
theProp: VueTypes.oneOfType([
String,
VueTypes.integer,
VueTypes.instanceOf(Person)
])
}
}
VueTypes.arrayOf()
Validates that a prop is an array of a certain type.
export default {
props: {
theProp: VueTypes.arrayOf(String)
}
}
//accepts: ['my', 'string']
//rejects: ['my', 1]
VueTypes.objectOf()
Validates that a prop is an object with values of a certain type.
export default {
props: {
userData: VueTypes.objectOf(String)
}
}
//accepts: userData = {name: 'John', surname: 'Doe'}
//rejects: userData = {name: 'John', surname: 'Doe', age: 30}
VueTypes.shape()
Validates that a prop is an object taking on a particular shape. Accepts both simple and vue-types
types. You can set shape's types as required
but (obviously) you cannot use .def()
export default {
props: {
userData: VueTypes.shape({
name: String,
age: VueTypes.integer,
id: VueTypes.integer.isRequired
})
}
}
//accepts: userData = {name: 'John', age: 30, id: 1}
//rejects: userData = {name: 'John', age: 'wrong data', id: 1}
//rejects: userData = {name: 'John', age: 'wrong data'} --> missing required `id` key
Copyright (c) 2016 Marco Solazzi
FAQs
Prop types utility for Vue
The npm package vue-types receives a total of 66,625 weekly downloads. As such, vue-types popularity was classified as popular.
We found that vue-types demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.