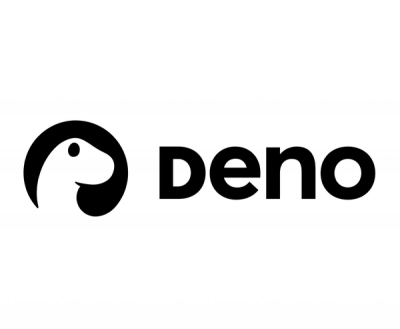
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
wabt.js is a port of WABT to the Web, allowing you to manipulate WebAssembly modules using a JavaScript API.
$> npm install wabt
var wabt = require("wabt");
var wasm = ...; // a buffer holding the contents of a wasm file
var myModule = wabt.readWasm(wasm, { readDebugNames: true });
myModule.applyNames();
var wast = myModule.toText({ foldExprs: false, inlineExport: false });
console.log(wast);
The buildbot also publishes nightly versions once a day if there have been changes. The latest nightly can be installed through
$> npm install wabt@nightly
or you can use one of the previous versions instead if necessary.
parseWat(filename: string
, buffer: string | Uint8Array
): WasmModule
Parses a wst source to a module.
readWasm(buffer: Uint8Array
, options: ReadWasmOptions
): WasmModule
Reads a wasm binaryen to a module.
WasmModule
A class representing a WebAssembly module.
void
void
void
void
ToTextOptions
): string
ToBinaryOptions
): ToBinaryResult
void
ReadWasmOptions
Options modifying the behavior of readWasm
.
boolean
ToTextOptions
Options modifying the behavior of WasmModule#toText
.
boolean
boolean
ToBinaryOptions
Options modifying the behavior of WasmModule#toBinary
.
boolean
boolean
boolean
boolean
ToBinaryResult
Result object of WasmModule#toBinary
.
buffer: Uint8Array
The wasm binary buffer.
log: string
Generated log output.
FAQs
JavaScript version of WABT, The WebAssembly Binary Toolkit.
We found that wabt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.