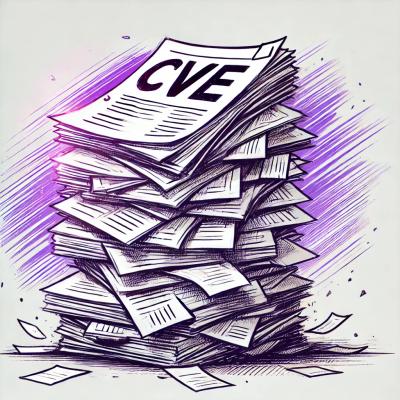
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
waii-sdk-js
Advanced tools
Typescript / Javascript SDK for the waii api. SQL generation and much more.
Welcome to the SDK documentation for WAII (World's most powerful SQL/AI API). This documentation provides detailed information on how to use the SDK to interact with the WAII system. The SDK allows enables developers to generate, explain, describe, modify and run SQL queries from text input, as well as manage a semantic layer, handle database connections, perform semantic search, and access the history of generated queries.
To get started with the WAII API, you first need to initialize the system. You can get your API key, by reaching out to us here: https://www.waii.ai
// Import the WAII module
import WAII from 'waii-sdk-js';
// Initialize the WAII system with the URL and API key
WAII.initialize('https://tweakit.waii.ai/api/', 'your_api_key');
If it's the first time you're using the system you have to add a database connection. This can be done ahead of time / outside the sdk (using the UI for instance).
let result = await WAII.Database.modifyConnections(
{
updated: [{
key: null, // will be generated by the system
account_name: 'your account',
database: 'your database name',
warehouse: 'your warehouse name',
role: 'desired user role',
username: 'your username',
password: 'your password',
db_type: 'snowflake',
}]
}
);
If you already have database connections configured you can activate them by key. Like so:
WAII.Database.activateConnection(<connection key>);
It can take little time depending on the number of tables in the system.
And now you are ready to generate queries:
let result = await WAII.Query.generate({
ask: 'show me the revenue month over month for the last three years by store location'
});
The Query module provides functions to generate, run, and manage SQL queries.
This function allows you to generate or refine/update a query based on the provided request parameters.
async function generate(params: QueryGenerationRequest, signal?: AbortSignal): Promise<GeneratedQuery>;
params
(required): An object containing the query generation request parameters.
search_context
(optional): An array of SearchContext
objects that specify the database, schema, and table names to consider during query generation. The system will automatically pick the needed objects, if this field is ommitted all the objects in the database will be considered (that's the common case).tweak_history
(optional): An array of Tweak
objects that provide additional information for query generation. This is to interactively update a query. The Tweaks are the previous asks and response queries and these will be refined with the new ask.ask
(optional): A string containing the english language statement for the query generation. Can we a question or a description of the requested result.uuid
(optional): A unique identifier for the query.dialect
(optional): The dialect of the query: Snowflake, or PostgreSQL.parent_uuid
(optional): The parent query's unique identifier. This is for interactive sessions and refers to the UUID from the previous interaction.signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GeneratedQuery
object containing the details of the generated SQL query.
The GeneratedQuery
object represents the details of a generated query and contains the following fields:
uuid
(optional): A string representing the unique identifier of the generated query. This can be later used for liking the query. Please refer to documentation of "Liking a Query".
liked
(optional): A boolean value indicating whether the query has been liked/favorited by the user. This is always false when the query is generated, but can be set via the "like" API.
tables
(optional): An array of TableName
objects representing the tables used in the generated query (this is a subset of the search_context). Each TableName
object may contain the following fields:
table_name
(required): The name of the table.schema_name
(optional): The name of the schema (if applicable).database_name
(optional): The name of the database (if applicable).semantic_context
(optional): An array of SemanticStatement
objects representing the semantic context of the generated query. Each SemanticStatement
object may contain the following fields:
id
(optional): A string representing the unique identifier of the semantic statement.scope
(required): A string representing the scope of the semantic statement. This is either a database, schema, table or column name. The semantic statement will be considered whenever that object is involved in a query.statement
(required): A string representing the semantic statement itself (e.g.: Describes the price of an item, final price is computed as 'price - discount'). This helps understand why a query was built a certain way.labels
(optional): An array of strings representing the labels associated with the semantic statement.query
(optional): A string representing the generated SQL query.
detailed_steps
(optional): An array of strings providing detailed steps or actions performed by the generated SQL query. This is the textual "explain plan".
what_changed
(optional): A string representing what changed in the query. This will be set when a generated query is refined with additional asks.
compilation_errors
(optional): An array of CompilationError
objects representing any compilation errors that occurred during query generation. Each CompilationError
object may contain the following fields:
message
(required): A string representing the compilation error message.line
(optional): An array of numbers representing the line numbers where the compilation error occurred.is_new
(optional): A boolean value indicating whether the generated query is new.
timestamp_ms
(optional): A number representing the timestamp (in milliseconds) when the query was generated.
Please note that some fields in the GeneratedQuery
object may be optional, and their presence depends on the details of the specific generated query.
This function allows you to run a given query.
async function run(params: RunQueryRequest, signal?: AbortSignal): Promise<GetQueryResultResponse>;
params
(required): An object containing the run query request parameters.
query
(required): The query string to be executed.session_id
(optional): The session ID for the query execution. We will use the same connection for specific sessionIds, thus it's possible to use stateful statements as well (e.g: use schema, create temproary table, etc)signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GetQueryResultResponse
object containing the query result details.
The GetQueryResultResponse
object represents the result of a query execution and contains the following fields:
rows
(optional): An array of objects representing the rows of the query result. Each object corresponds to a row, and its properties represent the column values.
more_rows
(optional): A number indicating the number of additional rows available for the query result. If the query result exceeds the maximum returned rows, this field indicates the number of remaining rows that can be fetched.
column_definitions
(optional): An array of Column
objects representing the column definitions of the query result. Each Column
object may contain the following fields:
name
(required): A string representing the name of the column.
type
(required): A string representing the data type of the column.
comment
(optional): A string representing any comment or description associated with the column.
sample_values
(optional): An array of ColumnSampleValues
objects representing sample values for the column. Each ColumnSampleValues
object may contain the following fields:
values
(optional): An array of objects representing sample values for the column, along with their respective counts. Each object may have value
(string) and count
(number) properties.query_uuid
(optional): A string representing the unique identifier of the query associated with the result.
Please note that some fields in the GetQueryResultResponse
object may be optional, and their presence depends on the specific result of the executed query.
This function allows you to like or favorite a specific query. This helps finding queries in the query history, but also tells the system when queries are correct to learn from them.
async function like(params: LikeQueryRequest, signal?: AbortSignal): Promise<LikeQueryResponse>;
params
(required): An object containing the like query request parameters.
query_uuid
(required): The unique identifier of the query to be liked or disliked.liked
(required): A boolean value indicating whether to like (true) or dislike (false) the query.signal
(optional): An AbortSignal object for aborting the request.
LikeQueryResponse
object confirming the success of the operation. The LikeQueryResponse
object has no fields, and is just a placeholder. If the query doesn't fail, the state is updated to mark the requested query as a favorite.This function allows you to submit a query for processing. This is the same as "Running a Query" except that this function is async. It returns an id that can be used later to retrieve a query response.
async function submit(params: RunQueryRequest, signal?: AbortSignal): Promise<RunQueryResponse>;
params
(required): An object containing the submit query request parameters.
query
(required): The query string to be submitted.session_id
(optional): The session ID for the query execution.signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a RunQueryResponse
object containing the query ID.
The RunQueryResponse
object represents the response of the "run query" operation and contains the following field:
query_id
(optional): A string representing the unique identifier of the executed query. This id is to be used in the "Get Query Results" call.This function allows you to get the results of a previously submitted query.
async function getResults(params: GetQueryResultRequest, signal?: AbortSignal): Promise<GetQueryResultResponse>;
params
(required): An object containing the get query results request parameters.
query_id
(required): The unique identifier of the query.signal
(optional): An AbortSignal object for aborting the request.
GetQueryResultResponse
object containing the query result data. For a description of this object please refer to the documentation of "Running a query".This function allows you to cancel a running query.
async function cancel(params: CancelQueryRequest, signal?: AbortSignal): Promise<CancelQueryResponse>;
params
(required): An object containing the cancel query request parameters.
query_id
(required): The unique identifier of the query to be canceled. This is the response of the "Submit Query" api.signal
(optional): An AbortSignal object for aborting the request.
CancelQueryResponse
object confirming the successful cancellation.This function allows you to describe a query, providing a summary and detailed steps.
async function describe(params: DescribeQueryRequest, signal?: AbortSignal): Promise<DescribeQueryResponse>;
params
(required): An object containing the describe query request parameters.
search_context
(optional): An array of SearchContext
objects that specify the database, schema, and table names related to the query. This is optional, when provided the query processing engine will only consider objects in the search context. If ommitted, all objects in the database will be considered.current_schema
(optional): The current schema name for the query. An optional hint to the system that the query is using a particular schema.query
(optional): The query string to be described.signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a `DescribeQueryResponse object containing the query description details. The DescribeQueryResponse object contains the following fields:
summary
(optional): A string representing a summary of the query's purpose or objective.
detailed_steps
(optional): An array of strings providing detailed steps or actions performed by the query.
tables
(optional): An array of TableName objects representing the tables involved in the query. Each TableName object may contain the following fields:
table_name
(required): The name of the table.schema_name
(optional): The name of the schema (if applicable).database_name
(optional): The name of the database (if applicable).Please note that some fields in the DescribeQueryResponse object may be optional, and their presence depends on the information available for the query or the provided search_context.
The Semantic Context module provides functions to modify and retrieve the semantic context.
This function allows you to modify the semantic context by adding or deleting semantic statements.
async function modifySemanticContext(params: ModifySemanticContextRequest, signal?: AbortSignal): Promise<ModifySemanticContextResponse>;
params
(required): An object containing the modify semantic context request parameters.
updated
(optional): An array of SemanticStatement
objects representing the updated semantic statements.deleted
(optional): An array of strings representing the IDs of the semantic statements to be deleted.validate_before_save
(optional): A boolean value indicating whether to validate the changes before saving.user_id
(optional): The ID of the user performing the modification.signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a ModifySemanticContextResponse
object containing the updated and deleted semantic statements.
The ModifySemanticContextResponse
object represents the response of the "modify semantic context" operation and contains the following fields:
updated
(optional): An array of SemanticStatement
objects representing the semantic statements that have been updated as a result of the operation. Each SemanticStatement
object may contain the following fields:
id
(optional): A string representing the unique identifier of the semantic statement.scope
(required): A string representing the scope of the semantic statement.statement
(required): A string representing the semantic statement itself.labels
(optional): An array of strings representing the labels associated with the semantic statement.deleted
(optional): An array of strings representing the IDs of the semantic statements that have been deleted as a result of the operation.
Please note that the updated
and deleted
fields in the ModifySemanticContextResponse
object may be optional, depending on the changes made during the "modify semantic context" operation.
This function allows you to retrieve the current semantic context.
async function getSemanticContext(params: GetSemanticContextRequest, signal?: AbortSignal): Promise<GetSemanticContextResponse>;
params
(optional): An object containing the get semantic context request parameters.
signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GetSemanticContextResponse
object containing the semantic statements.
The GetSemanticContextResponse
object represents the response of the "get semantic context" operation and contains the following field:
semantic_context
(optional): An array of SemanticStatement
objects representing the semantic statements retrieved from the server. Each SemanticStatement
object may contain the following fields:
id
(optional): A string representing the unique identifier of the semantic statement.scope
(required): A string representing the scope of the semantic statement.statement
(required): A string representing the semantic statement itself.labels
(optional): An array of strings representing the labels associated with the semantic statement.Please note that the semantic_context
field in the GetSemanticContextResponse
object may be optional.
The Database module provides functions to manage database connections and access schema and table information.
This function allows you to modify existing database connections or add new ones.
async function modifyConnections(params: ModifyDBConnectionRequest, signal?: AbortSignal): Promise<ModifyDBConnectionResponse>;
params
(required): An object containing the modify database connection request parameters.
updated
(optional): An array of DBConnection
objects representing the updated database connections.removed
(optional): An array of strings representing the keys of the database connections to be removed.validate_before_save
(optional): A boolean value indicating whether to validate the changes before saving.user_id
(optional): The ID of the user performing the modification.signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a ModifyDBConnectionResponse
object containing the updated connectors and diagnostics.
The ModifyDBConnectionResponse
object represents the response of the "modify database connection" operation and contains the following fields:
connectors
(optional): An array of DBConnection
objects representing the updated database connectors as a result of the operation. Each DBConnection
object may contain the following fields:
key
(required): A string representing the unique key or identifier of the database connection.db_type
(required): A string representing the type of the database (e.g., MySQL, PostgreSQL).description
(optional): A string representing a description or additional information about the database connection.account_name
(optional): A string representing the account name associated with the database connection.username
(optional): A string representing the username used to connect to the database.password
(optional): A string representing the password used to authenticate the database connection.database
(optional): A string representing the name of the specific database to connect to.warehouse
(optional): A string representing the warehouse for the database connection.role
(optional): A string representing the role associated with the database connection.path
(optional): A string representing the path to the database.parameters
(optional): An object containing additional parameters related to the database connection.This function allows you to retrieve the list of current database connections.
async function getConnections(params: GetDBConnectionRequest = {}, signal?: AbortSignal): Promise<GetDBConnectionResponse>;
params
(optional): An object containing the get database connection request parameters.
signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GetDBConnectionResponse
object containing the list of database connections and diagnostics.
The GetDBConnectionResponse
object represents the response of the "get database connections" operation and contains the following fields:
connectors
(optional): An array of DBConnection
objects representing the list of database connectors retrieved from the server. Each DBConnection
object may contain the following fields:
key
(required): A string representing the unique key or identifier of the database connection.db_type
(required): A string representing the type of the database (e.g., MySQL, PostgreSQL).description
(optional): A string representing a description or additional information about the database connection.account_name
(optional): A string representing the account name associated with the database connection.username
(optional): A string representing the username used to connect to the database.password
(optional): A string representing the password used to authenticate the database connection.database
(optional): A string representing the name of the specific database to connect to.warehouse
(optional): A string representing the warehouse for the database connection.role
(optional): A string representing the role associated with the database connection.path
(optional): A string representing the path to the database.parameters
(optional): An object containing additional parameters related to the database connection.This function allows you to activate a specific database connection for subsequent API calls.
function activateConnection(key: string): void;
key
(required): The key of the database connection to activate.This function allows you to fetch information about catalogs, schemas, and tables.
async function getCatalogs(params: GetCatalogRequest = {}, signal?: AbortSignal): Promise<GetCatalogResponse>;
params
(optional): An object containing the get catalogs request parameters.
signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GetCatalogResponse
object containing the list of catalogs and associated schema and table information.
The GetCatalogResponse
object represents the response of the "get catalogs" operation and contains the following fields:
catalogs
(optional): An array of Catalog
objects representing the list of catalogs retrieved from the server. Each Catalog
object may contain the following fields:
name
(required): A string representing the name of the catalog.
schemas
(optional): An array of Schema
objects representing the list of schemas associated with the catalog. Each Schema
object may contain the following fields:
name
(required): A SchemaName
object representing the name of the schema.tables
(optional): An array of Table
objects representing the list of tables in the schema. Each Table
object may contain the following fields:
name
(required): A TableName
object representing the name of the table.columns
(optional): An array of Column
objects representing the list of columns in the table. Each Column
object may contain the following fields:
name
(required): A string representing the name of the column.type
(required): A string representing the data type of the column.comment
(optional): A string representing any comment or description associated with the column.sample_values
(optional): An array of ColumnSampleValues
objects representing sample values for the column. Each ColumnSampleValues
object may contain the following fields:
values
(optional): An array of objects representing sample values for the column, along with their respective counts. Each object may have value
(string) and count
(number) properties.refs
(optional): An array of TableReference
objects representing the references to other tables. Each TableReference
object may contain the following fields:
src_table
(optional): An array of TableName
objects representing the source tables.src_cols
(optional): An array of strings representing the source columns.ref_table
(optional): An array of TableName
objects representing the referenced tables.ref_cols
(optional): An array of strings representing the referenced columns.description
(optional): A SchemaDescription
object representing the description of the schema.
The History module provides functions to access the history of generated queries.
This function allows you to retrieve the history of generated queries.
async function list(params: GetGeneratedQueryHistoryRequest = {}, signal?: AbortSignal): Promise<GetGeneratedQueryHistoryResponse>;
params
(optional): An object containing the get generated query history request parameters.
signal
(optional): An AbortSignal object for aborting the request.
A Promise resolving to a GetGeneratedQueryHistoryResponse
object containing the list of generated query history entries.
The GetGeneratedQueryHistoryResponse
object represents the responses of past "generated query" operations and contains the following field:
history
(optional): An array of GeneratedQueryHistoryEntry
objects representing the list of generated query history entries retrieved from the server. Each GeneratedQueryHistoryEntry
object may contain the following fields:
query
(optional): A GeneratedQuery
object representing the details of the generated query. The GeneratedQuery
object may contain the following fields:
uuid
(optional): A string representing the unique identifier of the generated query.liked
(optional): A boolean value indicating whether the query has been liked by the user.tables
(optional): An array of TableName
objects representing the tables involved in the generated query. Each TableName
object may contain the following fields:
table_name
(required): The name of the table.schema_name
(optional): The name of the schema (if applicable).database_name
(optional): The name of the database (if applicable).semantic_context
(optional): An array of SemanticStatement
objects representing the semantic context of the generated query. Each SemanticStatement
object may contain the following fields:
id
(optional): A string representing the unique identifier of the semantic statement.scope
(required): A string representing the scope of the semantic statement.statement
(required): A string representing the semantic statement itself.labels
(optional): An array of strings representing the labels associated with the semantic statement.query
(optional): A string representing the generated query.detailed_steps
(optional): An array of strings providing detailed steps or actions performed by the generated query.what_changed
(optional): A string representing what changed in the query.compilation_errors
(optional): An array of CompilationError
objects representing any compilation errors that occurred during query generation. Each CompilationError
object may contain the following fields:
message
(required): A string representing the compilation error message.line
(optional): An array of numbers representing the line numbers where the compilation error occurred.is_new
(optional): A boolean value indicating whether the generated query is new.timestamp_ms
(optional): A number representing the timestamp (in milliseconds) when the query was generated.request
(optional): A QueryGenerationRequest
object representing the request used for generating the query. The QueryGenerationRequest
object may contain the following fields:
search_context
(optional): An array of SearchContext
objects that specify the database, schema, and table names related to the query. Each SearchContext
object may contain the following fields:
db_name
(optional): The name of the database.schema_name
(optional): The name of the schema.table_name
(optional): The name of the table.tweak_history
(optional): An array of Tweak
objects representing the history of query tweaks applied during query generation. Each Tweak
object may contain the following fields:
sql
(optional): A string representing the SQL tweak applied.ask
(optional): A string representing the ask tweak applied.ask
(optional): A string representing the "ask" query input.uuid
(optional): A string representing the unique identifier of the generated query.dialect
(optional): A string representing the dialect used for the query.parent_uuid
(optional): A string representing the unique identifier of the parent query, if applicable.That's all folks! You have completed the API documentation for the WAII API. If you have any questions or need further assistance, please refer to the contact information provided on the webpage www.waii.ai . Happy coding!
FAQs
Typescript / Javascript SDK for the waii api. SQL generation and much more.
The npm package waii-sdk-js receives a total of 87 weekly downloads. As such, waii-sdk-js popularity was classified as not popular.
We found that waii-sdk-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.