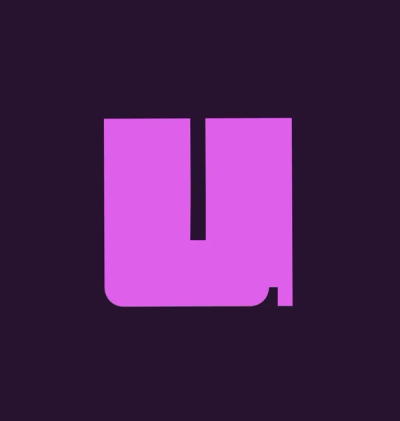
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
walt-compiler
Advanced tools
This is the main walt compiler package.
npm install --save walt-compiler
Compile and run Walt code in browser:
import compileWalt from 'walt-compiler';
const buffer = compileWalt(`
let counter: i32 = 0;
export function count(): i32 {
counter += 1;
return counter;
}
`);
WebAssembly.instantiate(buffer).then(result => {
console.log(`First invocation: ${result.instance.exports.count()}`);
console.log(`Second invocation: ${result.instance.exports.count()}`);
});
Compile and save a .wasm
file via Node.js:
const compileWalt = require('walt-compiler').default;
const fs = require('fs');
const buffer = compileWalt(`
let counter: i32 = 0;
export function count(): i32 {
counter += 1;
return counter;
}
`);
fs.writeFileSync('bin.wasm', new Uint8Array(buffer));
Main parse step of the compiler. Returns a bare bones Abstract Syntax Tree without type information. This tree cannot be directly compiled without semantic analysis, but can be transformed easily.
import { parser } from 'walt-compiler';
const ast = parser(`
type MyObjectType = { 'foo': i32, 'bar': f32 };
export function test(): f32 {
const obj: MyObjectType = 0;
obj['foo'] = 2;
obj['bar'] = 2.0;
return obj['foo'] + obj['bar'];
}
`);
console.log(ast); // { value: 'ROOT_NODE', Type: 'Program', range: [...], params: [...] .... }
Provides a human readable output of the AST nodes. Returns a string, does not log to console.
Semantic Analyzer, takes a bare AST and returns a transformed version of the AST with semantics applied(type indexes etc.,).
Intermediate code generator, takes an AST and returns a Program object.
Binary emitter, takes a Program and returns an OutputStream object. OutputStream.buffer()
generates a "real" binary buffer.
Used for debugging the buffer returned by emitter. Returns a string representation of the binary program.
FAQs
Alternative syntax for WebAssembly text format
We found that walt-compiler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.