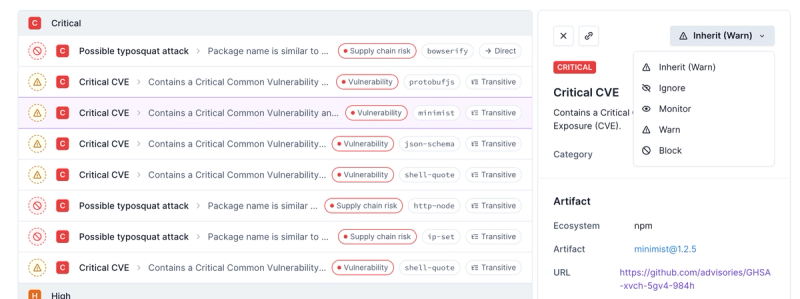
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
wasm-brotli
Advanced tools
Readme
WebAssembly compiled Brotli library.
npm install -S wasm-brotli
The awesome thing about
wasm-brotli
is that it does not need to compile or download any prebuilt binaries!
Because WebAssembly is supported on both Node.js and several browsers,
wasm-brotli
is super easy to use.
An example of compressing something and saving it to a file via Node.js.
import brotli from 'wasm-brotli';
import { writeFile } from 'fs';
import { promisify } from 'util';
const writeFileAsync = promisify(writeFile);
const content = Buffer.from('Hello, world!', 'utf8');
(async () => {
try {
const compressedContent = await brotli(content);
await writeFileAsync('./hello_world.txt.br', compressedContent);
} catch (err) {
console.error(err);
}
})();
An example of compressing something and downloading it from the browser.
import brotli from 'wasm-brotli';
const content = new TextEncoder('utf-8').encode('Hello, world!');
(async () => {
try {
const compressedContent = await brotli(content);
const file = new File([compressedContent], 'hello_world.txt.gz', { type: 'application/brotli' });
const link = document.createElement('a');
link.setAttribute('href', URL.createObjectURL(file));
link.setAttribute('download', file.name);
link.click();
} catch (err) {
console.error(err);
}
})();
data
<Uint8Array>
Compress data
using Brotli compression.
Want to see how fast this is? Go to the benchmark directory to see results, instructions on running your own benchmark, and more.
To build wasm-brotli
you will need to install Docker, and
pull rustlang/rust:nightly
. After that all that is needed is
to do the following:
npm install
npm run build
npm test
FAQs
🗜 WebAssembly compiled Brotli library
The npm package wasm-brotli receives a total of 8,501 weekly downloads. As such, wasm-brotli popularity was classified as popular.
We found that wasm-brotli demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.