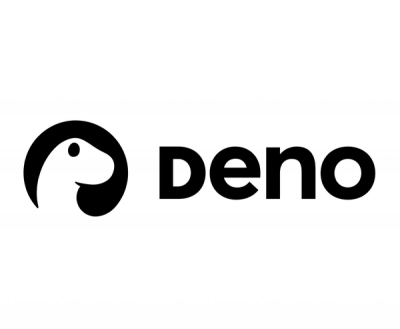
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
wc-context
Advanced tools
A context implementation for web components
✓ Small, fast and flexible
✓ No need to dedicated "provider" or "consumer" elements
✓ Ability to provide one or more contexts per element
✓ Integrates with lit-element and skatejs
✓ No Internet Explorer support
Warning: the public interface may change in future
The simplest way to use wc-context
is through the withContext
class mixin
Live examples:
import { withContext } from 'wc-context'
class Provider extends withContext(HTMLElement) {
static get providedContexts () {
return {
theme: { value: 'blue' }
}
}
toggleTheme () {
this.updateProvidedContext('theme', 'newtheme')
}
}
import { withContext } from 'wc-context'
class Consumer extends withContext(HTMLElement) {
static get observedContexts () {
return ['theme']
}
contextChangedCallback(name, oldValue, value) {
console.log(
`theme changed from "${oldValue}" to "${value}"`
);
// updates el accordingly
}
connectedCallback () {
super.connectedCallback()
this.innerHTML = `<div>Theme is ${this.context.theme}</div>`
}
}
Along side the generic class mixin, wc-context
provides specialized mixins that hooks into lit-element / skatejs reactivity system
// @polymer/lit-element
import { withContext } from 'wc-context/lit-element'
import { LitElement } from 'lit-element'
const Component = withContext(LitElement)
class Provider extends Component {
static get properties () {
return {
value: {type: String}
}
}
static get providedContexts () {
return {
theme: {property: 'value'}
}
}
toggleTheme () {
this.value = 'newtheme'
}
}
// skatejs
import Element from '@skatejs/element-lit-html'
import { withContext } from 'wc-context/skatejs'
const Component = withContext(Element)
class Provider extends Component {
static get props () {
return {
altTheme: String,
theme: String
}
}
static get providedContexts () {
return {
theme: {property: 'theme'}
altTheme: {property: 'altTheme'}
}
}
constructor () {
super()
this.theme = 'blue'
this.altTheme = 'yellow'
}
toggleTheme () {
this.theme = 'newtheme'
this.altTheme = 'newalttheme'
}
}
wc-context
also exports its low level functions that can be used to handle specific cases or create a new interface
import { registerProvidedContext, notifyContextChange } from 'wc-context/core'
// custom element that publishes an arbitrary context key and value
class ContextProvider extends HTMLElement {
connnectedCallback () {
const providedContexts = this.__providedContexts || (this.__providedContexts = {})
providedContexts[this.key] = this.value
registerProvidedContext(this, this.key, providedContexts)
}
set value (val) {
const providedContexts = this.__providedContexts || (this.__providedContexts = {})
providedContexts[this.key] = val
this.__value = val
notifyContextChange(this, this.key, val)
}
get value () {
return this.__value
}
}
customElements.define('context-provider', ContextProvider)
// later
import { html } from 'lit-html'
let theme = 'blue'
html`<context-provider .key="theme" .value=${theme}>
<div>
<my-theme-consumer></my-theme-consumer>
</div>
</context-provider>`
MIT Copyright © 2019 Luiz Américo
FAQs
Context for HTML custom elements / web components
The npm package wc-context receives a total of 1,257 weekly downloads. As such, wc-context popularity was classified as popular.
We found that wc-context demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.