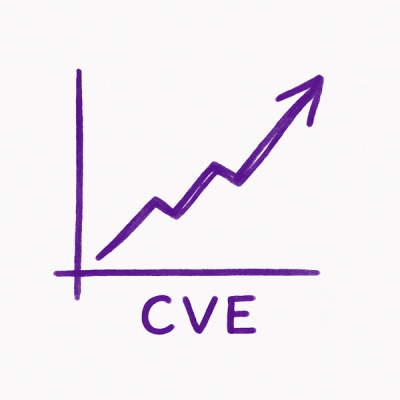
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
webpack-remove-empty-scripts
Advanced tools
Webpack plugin removes empty JavaScript files generated when using styles.
The webpack-remove-empty-scripts package is a plugin for Webpack that helps to remove empty scripts generated during the build process. This can be particularly useful when using tools like MiniCssExtractPlugin, which can sometimes generate empty JavaScript files when only CSS is being extracted.
Remove Empty Scripts
This feature allows you to remove empty JavaScript files that are generated during the Webpack build process. By including the RemoveEmptyScriptsPlugin in your Webpack configuration, you can ensure that any empty scripts are automatically removed, keeping your build output clean.
const RemoveEmptyScriptsPlugin = require('webpack-remove-empty-scripts');
module.exports = {
plugins: [
new RemoveEmptyScriptsPlugin(),
],
};
The clean-webpack-plugin is used to remove/clean your build folder(s) before building. While it doesn't specifically target empty scripts, it helps in keeping the build directory clean by removing old files. This can indirectly help in managing unnecessary files but does not specifically address the issue of empty scripts.
The webpack-shell-plugin-next allows you to run shell commands before or after Webpack builds. You could use this to run a script that removes empty files, but it requires additional setup and scripting compared to the more focused webpack-remove-empty-scripts plugin.
A Webpack plugin to remove empty JavaScript files generated when using style only entries.
By default, Webpack creates a JavaScript file for every entry specified in the entry
option - even when the entry is a style file (like SCSS or CSS).
Example:
module.exports = {
entry: {
styles: './styles.scss',
},
}
Output:
dist/styles.css
dist/styles.js // <= unwanted empty JS file
When using mini-css-extract-plugin
, CSS is correctly extracted into a separate file, but Webpack still emits an empty JavaScript file for each style-only entry - the known issue.
This plugin detects and removes those redundant .js
files automatically, keeping your output clean.
Note
This plugin is compatible with
Webpack 5
. ForWebpack 4
use webpack-fix-style-only-entries.
npm install webpack-remove-empty-scripts --save-dev
See the full documentation on GitHub.
FAQs
Webpack plugin removes empty JavaScript files generated when using styles.
The npm package webpack-remove-empty-scripts receives a total of 147,272 weekly downloads. As such, webpack-remove-empty-scripts popularity was classified as popular.
We found that webpack-remove-empty-scripts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.