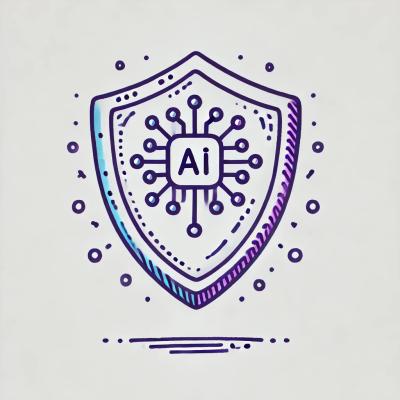
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
websocket-as-promised
Advanced tools
A WebSocket client library providing Promise-based API for connecting, disconnecting and messaging with server
A WebSocket client library with Promise-based API for browser and Node.js.
import WebSocketAsPromised from 'websocket-as-promised';
const wsp = new WebSocketAsPromised('ws://example.com');
await wsp.open();
wsp.send('message');
await wsp.close();
Promise
constructornpm i websocket-as-promised --save
import WebSocketAsPromised from 'websocket-as-promised';
const wsp = new WebSocketAsPromised('ws://example.com');
wsp.open()
.then(() => wsp.send('message'))
.then(() => wsp.close())
.catch(e => console.error(e));
Or with ES7 async / await:
import WebSocketAsPromised from 'websocket-as-promised';
const wsp = new WebSocketAsPromised('ws://example.com');
(async () => {
try {
await wsp.open();
wsp.send('message');
} catch(e) {
console.error(e);
} finally {
await wsp.close();
}
})();
As there is no built-in WebSocket client in Node.js, you should use a third-party WebSocket module.
Here you should use W3C compatible client - W3CWebSocket:
const WebSocketAsPromised = require('websocket-as-promised');
const W3CWebSocket = require('websocket').w3cwebsocket;
const wsp = new WebSocketAsPromised('ws://example.com', {
createWebSocket: url => new W3CWebSocket(url)
});
wsp.open()
.then(() => wsp.send('message'))
.then(() => wsp.close())
.catch(e => console.error(e));
Here it is important to define extractMessageData
option as event data are passed directly to onmessage
handler,
not as event.data
by spec:
const WebSocketAsPromised = require('websocket-as-promised');
const WebSocket = require('ws');
const wsp = new WebSocketAsPromised('ws://example.com', {
createWebSocket: url => new WebSocket(url),
extractMessageData: event => event, // <- this is important
});
wsp.open()
.then(() => wsp.send('message'))
.then(() => wsp.close())
.catch(e => console.error(e));
To send raw data use .send()
method:
wsp.send('foo');
To handle raw data from server use .onMessage
channel:
wsp.onMessage.addListener(data => console.log(data));
To send JSON you should define packMessage / unpackMessage
options:
const wsp = new WebSocketAsPromised(wsUrl, {
packMessage: data => JSON.stringify(data),
unpackMessage: data => JSON.parse(data)
});
To send data use .sendPacked()
method passing json as parameter:
wsp.sendPacked({foo: 'bar'});
To read unpacked data from received message you can subscribe to onUnpackedMessage
channel:
wsp.onUnpackedMessage.addListener(data => console.log(data.status));
Example of sending Uint8Array
:
const wsp = new WebSocketAsPromised(wsUrl, {
packMessage: data => (new Uint8Array(data)).buffer,
unpackMessage: data => new Uint8Array(data),
});
wsp.open()
.then(() => wsp.sendPacked([1, 2, 3]))
.then(() => wsp.close())
.catch(e => console.error(e));
websocket-as-promised provides simple request-response mechanism (JSON RPC).
Method .sendRequest()
sends message with unique requestId
and returns promise.
That promise get resolved when response message with the same requestId
comes.
For reading/setting requestId
from/to message there are two functions defined in options attachRequestId / extractRequestId
:
const wsp = new WebSocketAsPromised(wsUrl, {
packMessage: data => JSON.stringify(data),
unpackMessage: data => JSON.parse(data),
attachRequestId: (data, requestId) => Object.assign({id: requestId}, data), // attach requestId to message as `id` field
extractRequestId: data => data && data.id, // read requestId from message `id` field
});
wsp.open()
.then(() => wsp.sendRequest({foo: 'bar'})) // actually sends {foo: 'bar', id: 'xxx'}, because `attachRequestId` defined above
.then(response => console.log(response)); // waits server message with corresponding requestId: {id: 'xxx', ...}
By default requestId
value is auto-generated, but you can set it manually:
wsp.sendRequest({foo: 'bar'}, {requestId: 42});
Note: you should implement yourself attaching
requestId
on server side.
Object
Kind: global class
WebSocket
String
Boolean
Boolean
Boolean
Boolean
Channel
Channel
Channel
Channel
Channel
Channel
Channel
Promise.<Event>
Promise
Promise.<Event>
Constructor. Unlike original WebSocket it does not immediately open connection.
Please call open()
method to connect.
Param | Type | Description |
---|---|---|
url | String | WebSocket URL |
[options] | Options |
WebSocket
Returns original WebSocket instance created by options.createWebSocket
.
Kind: instance property of WebSocketAsPromised
String
Returns WebSocket url.
Kind: instance property of WebSocketAsPromised
Boolean
Is WebSocket connection in opening state.
Kind: instance property of WebSocketAsPromised
Boolean
Is WebSocket connection opened.
Kind: instance property of WebSocketAsPromised
Boolean
Is WebSocket connection in closing state.
Kind: instance property of WebSocketAsPromised
Boolean
Is WebSocket connection closed.
Kind: instance property of WebSocketAsPromised
Channel
Event channel triggered when connection is opened.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onOpen.addListener(() => console.log('Connection opened'));
Channel
Event channel triggered every time when message is sent to server.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onSend.addListener(data => console.log('Message sent', data));
Channel
Event channel triggered every time when message received from server.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onMessage.addListener(message => console.log(message));
Channel
Event channel triggered every time when received message is successfully unpacked. For example, if you are using JSON transport, the listener will receive already JSON parsed data.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onUnpackedMessage.addListener(data => console.log(data.foo));
Channel
Event channel triggered every time when response to some request comes. Received message considered a response if requestId is found in it.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onResponse.addListener(data => console.log(data));
Channel
Event channel triggered when connection closed.
Listener accepts single argument {code, reason}
.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onClose.addListener(event => console.log(`Connections closed: ${event.reason}`));
Channel
Event channel triggered when by Websocket 'error' event.
Kind: instance property of WebSocketAsPromised
See: https://vitalets.github.io/chnl/#channel
Example
wsp.onError.addListener(event => console.error(event));
Promise.<Event>
Opens WebSocket connection. If connection already opened, promise will be resolved with "open event".
Kind: instance method of WebSocketAsPromised
Promise
Performs request and waits for response.
Kind: instance method of WebSocketAsPromised
Param | Type | Default |
---|---|---|
data | * | |
[options] | Object | |
[options.requestId] | String | Number | <auto-generated> |
[options.timeout] | Number | 0 |
Packs data with options.packMessage
and sends to the server.
Kind: instance method of WebSocketAsPromised
Param | Type |
---|---|
data | * |
Sends data without packing.
Kind: instance method of WebSocketAsPromised
Param | Type |
---|---|
data | String | Blob | ArrayBuffer |
Promise.<Event>
Closes WebSocket connection. If connection already closed, promise will be resolved with "close event".
Kind: instance method of WebSocketAsPromised
Removes all listeners from WSP instance. Useful for cleanup.
Kind: instance method of WebSocketAsPromised
Object
Kind: global typedef
Defaults: please see options.js
Properties
Name | Type | Default | Description |
---|---|---|---|
[createWebSocket] | function | url => new WebSocket(url) | custom function for WebSocket construction. |
[packMessage] | function | noop | packs message for sending. For example, data => JSON.stringify(data) . |
[unpackMessage] | function | noop | unpacks received message. For example, data => JSON.parse(data) . |
[attachRequestId] | function | noop | injects request id into data. For example, (data, requestId) => Object.assign({requestId}, data) . |
[extractRequestId] | function | noop | extracts request id from received data. For example, data => data.requestId . |
[extractMessageData] | function | event => event.data | extracts data from event object. |
timeout | Number | 0 | timeout for opening connection and sending messages. |
connectionTimeout | Number | 0 | special timeout for opening connection, by default equals to timeout . |
Please see CHANGELOG.md.
MIT @ Vitaliy Potapov
1.0.0 (Nov 21, 2019)
FAQs
A WebSocket client library providing Promise-based API for connecting, disconnecting and messaging with server
The npm package websocket-as-promised receives a total of 1,025 weekly downloads. As such, websocket-as-promised popularity was classified as popular.
We found that websocket-as-promised demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.