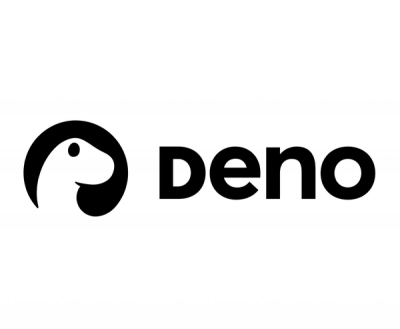
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Websocket Client & Server Library tracking the latest protocol drafts from the IETF.
The 'websocket' npm package provides a WebSocket server and client for Node.js, allowing for real-time, bidirectional communication between a client and server over a single, long-lived connection.
WebSocket Server
This code sets up a basic WebSocket server using the 'websocket' package. It listens for incoming WebSocket connections, accepts them, and allows for message exchange between the server and connected clients.
const WebSocketServer = require('websocket').server;
const http = require('http');
const server = http.createServer((request, response) => {
response.writeHead(404);
response.end();
});
server.listen(8080, () => {
console.log('Server is listening on port 8080');
});
const wsServer = new WebSocketServer({
httpServer: server
});
wsServer.on('request', (request) => {
const connection = request.accept(null, request.origin);
console.log('Connection accepted.');
connection.on('message', (message) => {
if (message.type === 'utf8') {
console.log('Received Message: ' + message.utf8Data);
connection.sendUTF('Hello from server!');
}
});
connection.on('close', (reasonCode, description) => {
console.log('Peer ' + connection.remoteAddress + ' disconnected.');
});
});
WebSocket Client
This code demonstrates how to create a WebSocket client using the 'websocket' package. The client connects to a WebSocket server, handles connection events, and sends random numbers to the server at regular intervals.
const WebSocketClient = require('websocket').client;
const client = new WebSocketClient();
client.on('connectFailed', (error) => {
console.log('Connect Error: ' + error.toString());
});
client.on('connect', (connection) => {
console.log('WebSocket Client Connected');
connection.on('error', (error) => {
console.log('Connection Error: ' + error.toString());
});
connection.on('close', () => {
console.log('Connection Closed');
});
connection.on('message', (message) => {
if (message.type === 'utf8') {
console.log('Received: ' + message.utf8Data);
}
});
function sendNumber() {
if (connection.connected) {
const number = Math.round(Math.random() * 0xFFFFFF);
connection.sendUTF(number.toString());
setTimeout(sendNumber, 1000);
}
}
sendNumber();
});
client.connect('ws://localhost:8080/', 'echo-protocol');
The 'ws' package is a popular WebSocket implementation for Node.js. It is known for its performance and simplicity. Compared to 'websocket', 'ws' is more lightweight and has a larger community, making it a preferred choice for many developers.
The 'socket.io' package provides a WebSocket-like API but with additional features such as fallback to HTTP long-polling, automatic reconnection, and rooms/namespaces support. It is more feature-rich compared to 'websocket' and is suitable for applications requiring more advanced real-time communication capabilities.
WARNING: This is an experimental library implementing the most recent draft of the WebSocket proposal.
Note about FireFox 6: Firefox 6 re-enables support for WebSockets by default. It uses a prefixed constructor name, MozWebSocket(), to avoid conflicting with already deployed scripts. It also implements draft-07, so if you want to target Firefox 6, you will need to checkout my draft-07 branch, not the latest one.
This code is currently unproven. It should be considered alpha quality, and is not recommended for production use, though it is used in production on worlize.com. Your mileage may vary.
This is a pure JavaScript implementation of the WebSocket Draft -09 for Node. There are some example client and server applications that implement various interoperability testing protocols in the "test" folder.
For a WebSocket -09 client written in Flash see my AS3WebScocket project.
There will not be a draft-08 implementation, as the -08 specification was only out for a week before being superseded by -09.
If you're looking for the version supporting draft-07 or draft-06, see the draft-07 or draft-06 branches. It will not be maintained, as I plan to track each subsequent draft of the protocol until it's finalized, and will ultimately be supporting only the final draft.
Tested against Node version 0.4.7. It may work in earlier versions but I haven't tried it. YMMV.
In your project root:
$ npm install websocket
Then in your code:
var WebSocketServer = require('websocket').server;
var WebSocketClient = require('websocket').client;
var WebSocketFrame = require('websocket').frame;
var WebSocketRouter = require('websocket').router;
Here's a short example showing a server that echos back anything sent to it, whether utf-8 or binary.
#!/usr/bin/env node
var WebSocketServer = require('websocket').server;
var http = require('http');
var server = http.createServer(function(request, response) {
console.log((new Date()) + " Received request for " + request.url);
response.writeHead(404);
response.end();
});
server.listen(8080, function() {
console.log((new Date()) + " Server is listening on port 8080");
});
wsServer = new WebSocketServer({
httpServer: server,
autoAcceptConnections: true
});
wsServer.on('connect', function(connection) {
console.log((new Date()) + " Connection accepted.");
connection.on('message', function(message) {
if (message.type === 'utf8') {
console.log("Received Message: " + message.utf8Data);
connection.sendUTF(message.utf8Data);
}
else if (message.type === 'binary') {
console.log("Received Binary Message of " + message.binaryData.length + " bytes");
connection.sendBytes(message.binaryData);
}
});
connection.on('close', function(connection) {
console.log((new Date()) + " Peer " + connection.remoteAddress + " disconnected.");
});
});
This is a simple example client that will print out any utf-8 messages it receives on the console, and periodically sends a random number.
#!/usr/bin/env node
var WebSocketClient = require('websocket').client;
var client = new WebSocketClient();
client.on('error', function(error) {
console.log("Connect Error: " + error.toString());
});
client.on('connect', function(connection) {
console.log("WebSocket client connected");
connection.on('error', function(error) {
console.log("Connection Error: " + error.toString());
});
connection.on('close', function() {
console.log("dumb-increment-protocol Connection Closed");
})
connection.on('message', function(message) {
console.log("Received: '" + message.utf8Data + "'");
});
function sendNumber() {
if (connection.connected) {
var number = Math.round(Math.random() * 0xFFFFFF);
connection.sendUTF(number);
setTimeout(sendNumber, 1000);
}
}
sendNumber();
});
client.connect("ws://localhost:8080/", ['echo-protocol']);
For an example of using the request router, see libwebsockets-test-server.js
in the test
folder.
For more complete documentation, see the Documentation Wiki.
FAQs
Websocket Client & Server Library implementing the WebSocket protocol as specified in RFC 6455.
The npm package websocket receives a total of 932,915 weekly downloads. As such, websocket popularity was classified as popular.
We found that websocket demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.