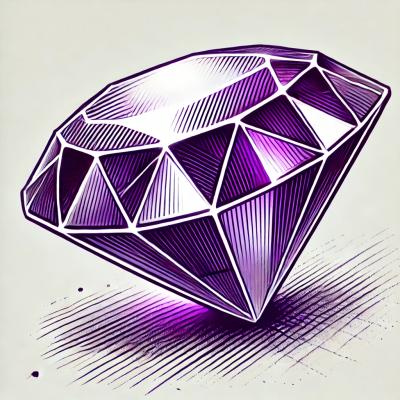
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
The 'when' npm package is a robust library for working with asynchronous programming in JavaScript, particularly using promises. It provides utilities for creating, managing, and composing promises, making it easier to handle asynchronous operations and their potential complexities.
Creating Promises
This feature allows the creation of new promises. The code sample demonstrates how to create a simple promise that resolves with 'Hello, World!' after 1 second.
const when = require('when');
const promise = when.promise(function(resolve, reject) {
setTimeout(() => resolve('Hello, World!'), 1000);
});
promise.then(response => console.log(response));
Chaining Promises
This feature demonstrates chaining multiple promises. It shows how to perform a series of tasks sequentially, where each task starts only after the previous one has completed.
const when = require('when');
const cleanRoom = () => when.promise(resolve => resolve('Room cleaned'));
const removeTrash = () => when.promise(resolve => resolve('Trash removed'));
const winIcecream = () => when.promise(resolve => resolve('Won ice cream'));
cleanRoom()
.then(result => {
console.log(result);
return removeTrash();
})
.then(result => {
console.log(result);
return winIcecream();
})
.then(result => console.log(result));
Handling Errors
This feature involves error handling in promises. The code sample shows how to catch and handle errors that occur during the execution of promises.
const when = require('when');
const failTask = () => when.promise((resolve, reject) => reject('Failed task'));
failTask()
.then(result => console.log('Success:', result))
.catch(error => console.log('Error:', error));
Bluebird is a full-featured promise library with a focus on innovative features and performance. It is similar to 'when' but often cited for its superior performance and additional features like cancellation, progress tracking, and more detailed stack traces.
Q is one of the earliest promise libraries that influenced many others. It offers a similar API to 'when' but is generally considered to be less performant in modern applications. It provides a straightforward approach to handling asynchronous operations with promises.
The 'promise' package provides a minimalist implementation similar to the ES6 Promise specification. It is more lightweight compared to 'when' but lacks some of the more advanced features and utilities provided by 'when'.
A lightweight CommonJS Promises/A and when()
implementation. It also provides several other useful Promise-related concepts, such as joining and chaining, and has a robust unit test suite.
It's just over 1k when compiled with Google Closure (w/advanced optimizations) and gzipped.
when.js was derived from the async core of wire.js.
exports
to avoid a problem with QUnit. See #54 for more info.when.resolve(value)
creates a resolved promise for value
. See API docs.deferred.resolve
and deferred.reject
now return a promise for the fulfilled or rejected value.deferred.resolve(promise)
- when promise
resolves or rejects, so will deferred
.git clone https://github.com/cujojs/when
or git submodule add https://github.com/cujojs/when
Configure your loader with a package:
packages: [
{ name: 'when', location: 'path/to/when/', main: 'when' },
// ... other packages ...
]
define(['when', ...], function(when, ...) { ... });
or require(['when', ...], function(when, ...) { ... });
git clone https://github.com/cujojs/when
or git submodule add https://github.com/cujojs/when
<script src="path/to/when/when.js"></script>
when
will be available as window.when
npm install when
var when = require('when');
ringo-admin install cujojs/when
var when = require('when');
Install buster.js
npm install -g buster
Run unit tests in Node:
buster test -e node
Run unit tests in Browsers (and Node):
buster server
- this will print a urllocalhost:1111/capture
buster test
or buster test -e browser
Much of this code was inspired by @unscriptable's tiny promises, the async innards of wire.js, and some gists here, here, here, and here
Some of the code has been influenced by the great work in Q, Dojo's Deferred, and uber.js.
1.4.4
exports
to avoid a problem with QUnit. See #54 for more info.FAQs
A lightweight Promises/A+ and when() implementation, plus other async goodies.
The npm package when receives a total of 556,295 weekly downloads. As such, when popularity was classified as popular.
We found that when demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.