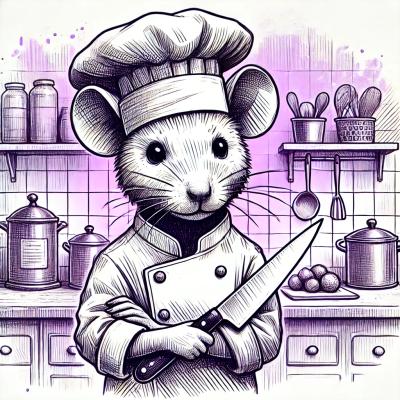
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
whois-history
Advanced tools
The client library for Whois History API for Node.js.
The minimum Node.js version is 8.
The library is distributed via npm
npm install whois-history
Full API documentation available here
const WhoisHistoryClient = require('whois-history').Client;
const Options = require('whois-history/include/client').Options;
let client = new WhoisHistoryClient(
'Your API Key'
);
// Check how many records available. It doesn't deduct credits.
client.preview('whoisxmlapi.com')
.then(function (data) {
console.log(data);
})
.catch(function (error) {
console.log(error);
});
// Get actual list of records.
client.purchase('whoisxmlapi.com')
.then(function (data) {
console.log(data);
})
.catch(function (error) {
console.log(error);
});
You can specify search options for these methods.
let date = new Date("2017-01-01")
let options = new Options()
options.sinceDate = date
options.createdDateFrom = date
options.createdDateTo = date
options.updatedDateFrom = date
options.updatedDateTo = date
options.expiredDateFrom = date
options.expiredDateTo = date
client.preview('whoisxmlapi.com', options)
.then(function (data) {
console.log(data);
})
.catch(function (error) {
console.log(error);
});
client.preview('whoisxmlapi.com', new Options(), function (err, res) {
if (err) {
console.log(err);
} else {
console.log(res);
}
});
FAQs
Whois History API client for Node.js
We found that whois-history demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.