
worker_map
A simple abstraction for Node.js worker_threads
, allowing you to create and share a Map (hash table)
between worker threads and the main process. This simplifies the process of managing shared data and communication between worker threads.
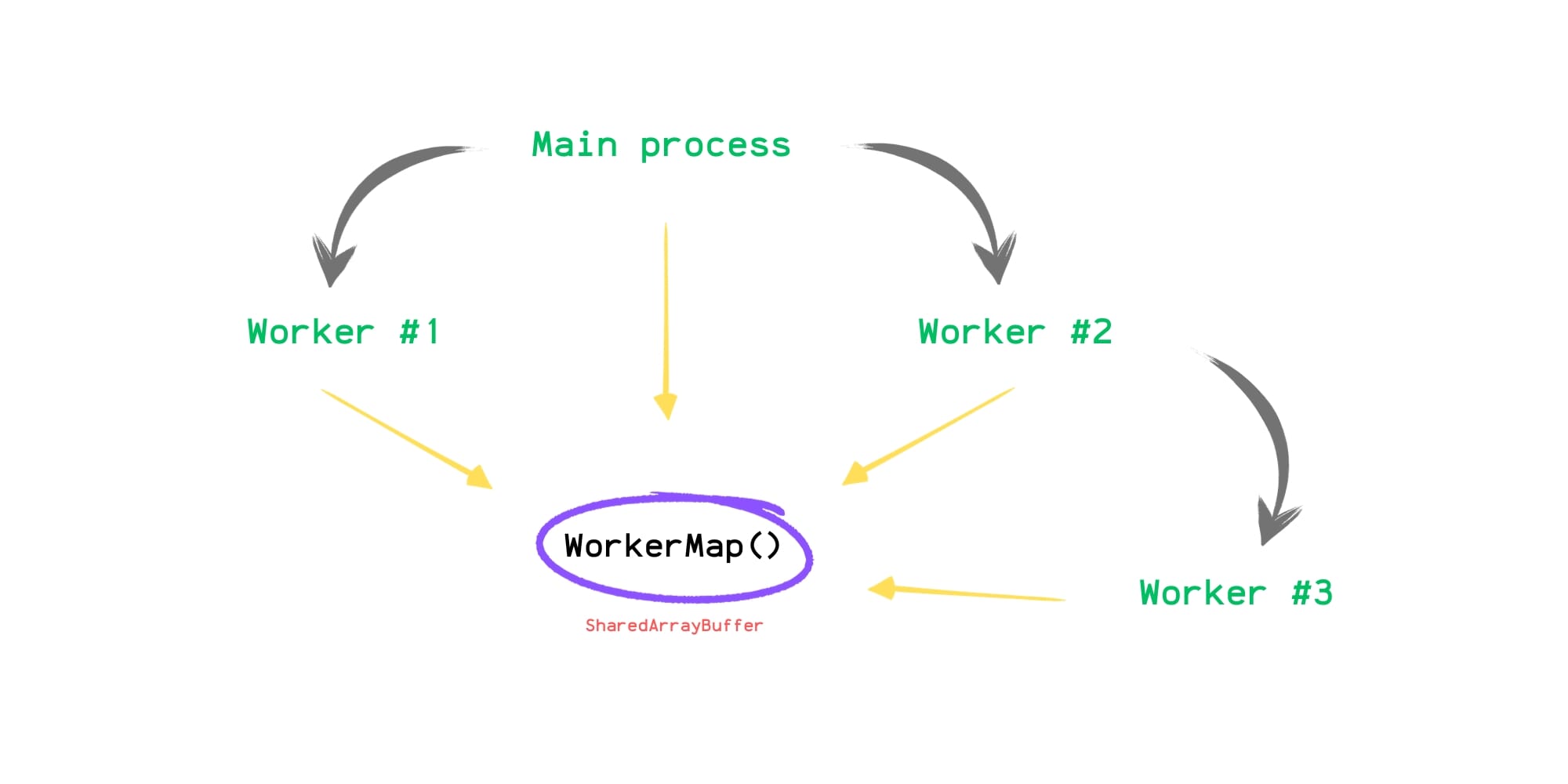
Under the hood, the library uses SharedArrayBuffer
to create shared memory, enabling seamless data sharing between threads. Additionally, it uses Atomics
mechanism to implement a mutex, ensuring thread safety and preventing race conditions during data access and manipulation.
Installation
npm i worker_map
Basic Example
First, let's create a simple hash map structure in main process, then create a worker thread and share the hash.
const { Worker } = require('worker_threads');
const { WorkerMap } = require('worker_map');
const map = new WorkerMap();
map.set('balance', 100);
new Worker('./worker.js', {
workerData: {
mapBuffer: map.toSharedBuffer(),
},
});
setTimeout(() => {
console.log(map.get('balance'));
}, 50);
Now, let's access the shared hash map structure in the worker thread.
const { WorkerMap } = require('worker_map');
const { workerData } = require('worker_threads');
const map = new WorkerMap(workerData.mapBuffer);
console.log(map.get('balance'));
map.set('balance', 200);
Instance methods
map.get(key):
const name = map.get('name');
map.set(key, value)
map.set('name', 'John');
map.delete(key):
map.delete('name'); // true
map.delete('something'); // false because doesn't exist
map.has(key)
map.has('name'); // true
map.has('country'); // false
map.size()
map.has('size'); // 1
map.keys()
map.keys(); // [ 'name' ]
map.toSharedBuffer()
const buffer = map.toSharedBuffer();
const sameMap = new WorkerMap(buffer);
map.toObject()
const mapObject = map.toObject();
mapObject.name; // 'John'
TODO
map.clear()
map.entries()
map.forEach()
Limitations
Please be aware of the following limitations when using our library:
- Functions: Function types are not supported.
- NaN Values: NaN values are not supported.