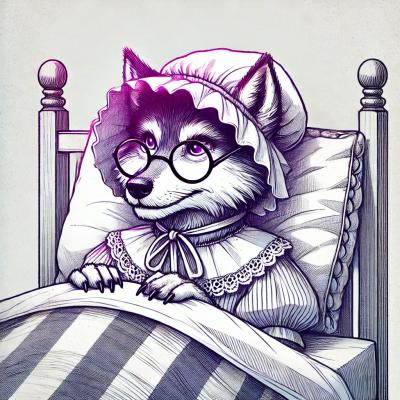
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
write-file-atomic
Advanced tools
The write-file-atomic npm package is designed to write files to disk atomically, meaning that it ensures that the file contents are either fully written or not written at all, preventing partial writes that can occur in crash scenarios. It also allows for setting file mode and ownership atomically with the file write.
Atomic file writing
This feature ensures that the file is either completely written or not written at all, which is useful for preventing data corruption during write operations.
const writeFileAtomic = require('write-file-atomic');
writeFileAtomic('message.txt', 'Hello, World!', function (err) {
if (err) throw err;
console.log('The file has been saved!');
});
Setting file mode
This feature allows the user to set the file mode (permissions) at the time of writing the file atomically.
const writeFileAtomic = require('write-file-atomic');
writeFileAtomic('message.txt', 'Hello, World!', { mode: 0o755 }, function (err) {
if (err) throw err;
console.log('The file has been saved with the specified mode!');
});
Setting file ownership
This feature allows the user to set the file ownership (user ID and group ID) atomically with the file write operation.
const writeFileAtomic = require('write-file-atomic');
writeFileAtomic('message.txt', 'Hello, World!', { chown: { uid: 1000, gid: 50 } }, function (err) {
if (err) throw err;
console.log('The file has been saved with the specified ownership!');
});
fs-extra is a package that builds on the native fs module, providing additional methods and ensuring compatibility across different platforms. It includes methods for atomic file writing, but also offers a wide range of other file system operations, making it more extensive than write-file-atomic.
graceful-fs is a drop-in replacement for the fs module that makes file system operations more robust by queuing them and retrying on failure. It does not specifically focus on atomic writes, but it enhances the overall reliability of file system interactions.
atomic-write is a minimalistic module that provides atomic file writing capabilities. It is similar to write-file-atomic but with a smaller feature set and API surface area, focusing solely on the atomicity of the write operation.
This is an extension for node's fs.writeFile
that makes its operation
atomic and allows you set ownership (uid/gid of the file).
Atomically and asynchronously writes data to a file, replacing the file if it already exists. data can be a string or a buffer.
The file is initially named filename + "." + murmurhex(__filename, process.pid, ++invocations)
.
If writeFile completes successfully then, if passed the chown option it will change
the ownership of the file. Finally it renames the file back to the filename you specified. If
it encounters errors at any of these steps it will attempt to unlink the temporary file and then
pass the error back to the caller.
If provided, the chown option requires both uid and gid properties or else you'll get an error.
The encoding option is ignored if data is a buffer. It defaults to 'utf8'.
Example:
writeFileAtomic('message.txt', 'Hello Node', {chown:{uid:100,gid:50}}, function (err) {
if (err) throw err;
console.log('It\'s saved!');
});
The synchronous version of writeFileAtomic.
FAQs
Write files in an atomic fashion w/configurable ownership
We found that write-file-atomic demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.