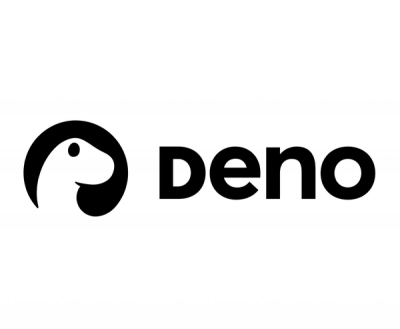
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
xhr-mock is a library for mocking XMLHttpRequests in JavaScript. It is particularly useful for testing purposes, allowing developers to simulate HTTP requests and responses without actually making network calls.
Mocking GET Requests
This feature allows you to mock GET requests. The code sample sets up the mock, defines a GET request handler that returns a mocked response, and then tears down the mock after the test.
const xhrMock = require('xhr-mock');
xhrMock.setup();
xhrMock.get('/api/data', (req, res) => {
return res.status(200).body('Mocked GET response');
});
// Your test code here
xhrMock.teardown();
Mocking POST Requests
This feature allows you to mock POST requests. The code sample sets up the mock, defines a POST request handler that returns a mocked response, and then tears down the mock after the test.
const xhrMock = require('xhr-mock');
xhrMock.setup();
xhrMock.post('/api/data', (req, res) => {
return res.status(201).body('Mocked POST response');
});
// Your test code here
xhrMock.teardown();
Mocking with Custom Headers
This feature allows you to mock requests with custom headers. The code sample sets up the mock, defines a GET request handler that returns a mocked response with custom headers, and then tears down the mock after the test.
const xhrMock = require('xhr-mock');
xhrMock.setup();
xhrMock.get('/api/data', (req, res) => {
return res.status(200).header('Content-Type', 'application/json').body(JSON.stringify({ message: 'Mocked response with headers' }));
});
// Your test code here
xhrMock.teardown();
Nock is a HTTP mocking and expectations library for Node.js. It intercepts HTTP requests and allows you to define custom responses. Compared to xhr-mock, Nock is more focused on Node.js environments and supports a wider range of HTTP methods and features.
Fetch-mock is a library for mocking fetch requests. It provides a similar functionality to xhr-mock but is designed for the Fetch API instead of XMLHttpRequest. It is useful for environments where fetch is used instead of XMLHttpRequest.
Sinon is a versatile library for spies, stubs, and mocks. It can mock XMLHttpRequests as well as other types of functions and objects. Compared to xhr-mock, Sinon offers a broader range of testing utilities beyond just HTTP request mocking.
Utility for mocking XMLHttpRequest
.
Great for testing. Great for prototyping while your backend is still being built.
Works in NodeJS and in the browser. Is compatible with Axios, jQuery, Superagent
and probably every other library built on XMLHttpRequest
. Standard compliant (http://xhr.spec.whatwg.org/).
If you are using a bundler like Webpack or Browserify then install xhr-mock
using yarn
or npm
:
yarn add --dev xhr-mock
Now import xhr-mock
and start using it in your scripts:
import mock from 'xhr-mock';
If you aren't using a bundler like Webpack or Browserify then add this script to your HTML:
<script src="https://unpkg.com/xhr-mock/dist/xhr-mock.js"></script>
Now you can start using the global, XHRMock
, in your scripts.
First off lets write some code that uses XMLHttpRequest
...
./createUser.js
// we could have just as easily use Axios, jQuery, Superagent
// or another package here instead of using the native XMLHttpRequest object
export default function(data) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = () => {
if (xhr.readyState == XMLHttpRequest.DONE) {
if (xhr.status === 201) {
try {
resolve(JSON.parse(xhr.responseText).data);
} catch (error) {
reject(error);
}
} else if (xhr.status) {
try {
reject(JSON.parse(xhr.responseText).error);
} catch (error) {
reject(error);
}
} else {
reject(new Error('An error ocurred whilst sending the request.'));
}
}
};
xhr.open('post', '/api/user');
xhr.setRequestHeader('Content-Type', 'application/json');
xhr.send(JSON.stringify({data: data}));
});
}
Now lets test the code we've written...
./createUser.test.js
import mock from 'xhr-mock';
import createUser from './createUser';
describe('createUser()', () => {
// replace the real XHR object with the mock XHR object before each test
beforeEach(() => mock.setup());
// put the real XHR object back and clear the mocks after each test
afterEach(() => mock.teardown());
it('should send the data as JSON', async () => {
expect.assertions(2);
mock.post('/api/user', (req, res) => {
expect(req.header('Content-Type')).toEqual('application/json');
expect(req.body()).toEqual('{"data":{"name":"John"}}');
return res.status(201).body('{"data":{"id":"abc-123"}}');
});
await createUser({name: 'John'});
});
it('should resolve with some data when status=201', async () => {
expect.assertions(1);
mock.post('/api/user', {
status: 201,
reason: 'Created',
body: '{"data":{"id":"abc-123"}}'
});
const user = await createUser({name: 'John'});
expect(user).toEqual({id: 'abc-123'});
});
it('should reject with an error when status=400', async () => {
expect.assertions(1);
mock.post('/api/user', {
status: 400,
reason: 'Bad request',
body: '{"error":"A user named \\"John\\" already exists."}'
});
try {
const user = await createUser({name: 'John'});
} catch (error) {
expect(error).toMatch('A user named "John" already exists.');
}
});
});
Replace the global XMLHttpRequest
object with the MockXMLHttpRequest
.
Restore the global XMLHttpRequest
object to its original state.
Forget all the request handlers.
Register a factory function to create mock responses for each GET request to a specific URL.
mock.get(/\.*.json$/, {
body: JSON.stringify({ data: { id: "abc" } })
});
Register a factory function to create mock responses for each POST request to a specific URL.
Register a factory function to create mock responses for each PUT request to a specific URL.
Register a factory function to create mock responses for each PATCH request to a specific URL.
Register a factory function to create mock responses for each DELETE request to a specific URL.
Register a factory function to create mock responses for each request to a specific URL.
Register a factory function to create mock responses for every request.
Log errors thrown by handlers.
Get the request method.
Get the request URL.
Set a request header.
Get a request header.
Get the request headers.
Set the request headers.
Get the request body.
Set the request body.
Get the response status.
Set the response status.
Get the response reason.
Set the response reason.
Set a response header.
Get a response header.
Get the response headers.
Set the response headers.
Get the response body.
Set the response body.
Set the Content-Length
header and send a body. xhr-mock
will emit ProgressEvent
s.
import mock from 'xhr-mock';
mock.setup();
mock.post('/', {});
const xhr = new XMLHttpRequest();
xhr.upload.onprogress = event => console.log(event.loaded, event.total);
xhr.open('POST', '/');
xhr.setRequestHeader('Content-Length', '12');
xhr.send('Hello World!');
Set the Content-Length
header and send a body. xhr-mock
will emit ProgressEvent
s.
import mock from 'xhr-mock';
mock.setup();
mock.get('/', {
headers: {'Content-Length': '12'},
body: 'Hello World!'
});
const xhr = new XMLHttpRequest();
xhr.onprogress = event => console.log(event.loaded, event.total);
xhr.open('GET', '/');
xhr.send();
Return a Promise
that never resolves or rejects.
import mock from 'xhr-mock';
mock.setup();
mock.get('/', () => new Promise(() => {}));
const xhr = new XMLHttpRequest();
xhr.timeout = 100;
xhr.ontimeout = event => console.log('timeout');
xhr.open('GET', '/');
xhr.send();
A number of major libraries don't use the
timeout
event and usesetTimeout()
instead. Therefore, in order to mock timeouts in major libraries, we have to wait for the specified amount of time anyway.
Return a Promise
that rejects. If you want to test a particular error you an use one of the pre-defined error classes.
import mock from 'xhr-mock';
mock.setup();
mock.get('/', () => Promise.reject(new Error()));
const xhr = new XMLHttpRequest();
xhr.onerror = event => console.log('error');
xhr.open('GET', '/');
xhr.send();
If you want to mock some requests, but not all of them, you can proxy unhandled requests to a real server.
import mock, {proxy} from 'xhr-mock';
mock.setup();
// mock specific requests
mock.post('/', {status: 204});
// proxy unhandled requests to the real servers
mock.use(proxy);
// this request will receive a mocked response
const xhr1 = new XMLHttpRequest();
xhr1.open('POST', '/');
xhr1.send();
// this request will receieve the real response
const xhr2 = new XMLHttpRequest();
xhr2.open('GET', 'https://jsonplaceholder.typicode.com/users/1');
xhr2.send();
Requests can be delayed using our handy delay
utility.
import mock, {delay} from 'xhr-mock';
mock.setup();
// delay the request for three seconds
mock.post('/', delay({status: 201}, 3000));
Requests can be made on one off occasions using our handy once
utility.
import mock, {once} from 'xhr-mock';
mock.setup();
// the response will only be returned the first time a request is made
mock.post('/', once({status: 201}));
In case you need to return a different response each time a request is made, you may use the sequence
utility.
import mock, {sequence} from 'xhr-mock';
mock.setup();
mock.post('/', sequence([
{status: 200}, // the first request will receive a response with status 200
{status: 500} // the second request will receive a response with status 500
// if a third request is made, no response will be sent
]
));
MIT Licensed. Copyright (c) James Newell 2014.
FAQs
Utility for mocking XMLHttpRequest.
The npm package xhr-mock receives a total of 148,515 weekly downloads. As such, xhr-mock popularity was classified as popular.
We found that xhr-mock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.