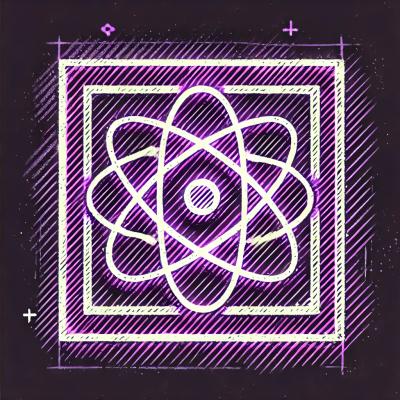
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Parser for Excel XLS (BIFF5/BIFF8) and 2003-2004 (XML) files. Pure-JS cleanroom implementation from the Microsoft Open Specifications and related documents.
In nodejs:
npm install xlsjs
In the browser:
<script src="xls.js"></script>
In bower:
bower install js-xls
CDNjs automatically pulls the latest version and makes all versions available at http://cdnjs.com/libraries/xls
The nodejs version automatically requires modules for additional features. Some of these modules are rather large in size and are only needed in special circumstances, so they do not ship with the core. For browser use, they must be included directly:
<!-- international support from https://github.com/sheetjs/js-codepage -->
<script src="dist/cpexcel.js"></script>
An appropriate version for each dependency is included in the dist/ directory.
The complete single-file version is generated at dist/xls.full.min.js
Since xlsx.js uses ES5 functions like Array#forEach
, older browsers require
Polyfills. This repo and the gh-pages branch include
a shim
To use the shim, add the shim before the script tag that loads xlsx.js:
<script type="text/javascript" src="/path/to/shim.js"></script>
For parsing, the first step is to read the file.
if(typeof require !== 'undefined') XLS = require('xlsjs');
var workbook = XLS.readFile('test.xls');
/* DO SOMETHING WITH workbook HERE */
/* set up XMLHttpRequest */
var url = "test_files/formula_stress_test_ajax.xls";
var oReq = new XMLHttpRequest();
oReq.open("GET", url, true);
oReq.responseType = "arraybuffer";
oReq.onload = function(e) {
var arraybuffer = oReq.response;
/* convert data to binary string */
var data = new Uint8Array(arraybuffer);
var arr = new Array();
for(var i = 0; i != data.length; ++i) arr[i] = String.fromCharCode(data[i]);
var bstr = arr.join("");
/* Call XLS */
var workbook = XLS.read(bstr, {type:"binary"});
/* DO SOMETHING WITH workbook HERE */
}
oReq.send();
/* set up drag-and-drop event */
function handleDrop(e) {
e.stopPropagation();
e.preventDefault();
var files = e.dataTransfer.files;
var i,f;
for (i = 0, f = files[i]; i != files.length; ++i) {
var reader = new FileReader();
var name = f.name;
reader.onload = function(e) {
var data = e.target.result;
/* if binary string, read with type 'binary' */
var wb = XLS.read(data, {type: 'binary'});
/* DO SOMETHING WITH workbook HERE */
};
reader.readAsBinaryString(f);
}
}
drop_dom_element.addEventListener('drop', handleDrop, false);
This example gets the value of cell A1 of the first worksheet:
var sheet_name_list = workbook.SheetNames;
var Sheet1A1 = workbook.Sheets[sheet_name_list[0]]['A1'].v;
Complete examples:
Note that older versions of IE does not support HTML5 File API, so the base64 mode is provided for testing. On OSX you can get the base64 encoding with:
$ <target_file.xls base64 | pbcopy
http://oss.sheetjs.com/js-xls/ajax.html XMLHttpRequest
https://github.com/SheetJS/js-xls/blob/master/bin/xls.njs nodejs
The nodejs version installs a binary xls
which can read XLS and XML2003
files and output the contents in various formats. The source is available at
xls.njs
in the bin directory.
Some helper functions in XLS.utils
generate different views of the sheets:
XLS.utils.sheet_to_csv
generates CSVXLS.utils.sheet_to_json
generates an array of objectsXLS.utils.get_formulae
generates a list of formulaeXLS
is the exposed variable in the browser and the exported nodejs variable
XLS.read(data, read_opts)
attempts to parse data
.
XLS.readFile(filename, read_opts)
attempts to read filename
and parse.
js-xls conforms to the Common Spreadsheet Format (CSF):
.SheetNames
is an ordered list of the sheets in the workbook
.Sheets[sheetname]
returns a data structure representing the sheet. Each key
that does not start with !
corresponds to a cell (using A-1
notation).
.Sheets[sheetname][address]
returns the specified cell:
.v
: the raw value of the cell.w
: the formatted text of the cell (if applicable).t
: the type of the cell (constrained to the enumeration ST_CellType
as
documented in page 4215 of ISO/IEC 29500-1:2012(E) ).f
: the formula of the cell (if applicable).z
: the number format string associated with the cell (if requested)For dates, .v
holds the raw date code from the sheet and .w
holds the text
The exported read
and readFile
functions accept an options argument:
Option Name | Default | Description |
---|---|---|
cellFormula | true | Save formulae to the .f field ** |
cellNF | false | Save number format string to the .z field |
sheetRows | 0 | If >0, read the first sheetRows rows ** |
bookFiles | false | If true, add raw files to book object ** |
bookProps | false | If true, only parse enough to get book metadata ** |
bookSheets | false | If true, only parse enough to get the sheet names |
password | "" | If defined and file is encrypted, use password ** |
cellFormula
only applies to constructing XLS formulae. XLML R1C1 formulae
are stored in plaintext, but XLS formulae are stored in a binary format.cellNF
is false, formatted text (.w) will be generatedbookSheets
is false.bookSheets
and bookProps
combine to give both sets of informationbookFiles
adds a cfb
object (XLS only)sheetRows-1
rows will be generated when looking at the JSON object output
(since the header row is counted as a row when parsing the data)Tests utilize the mocha testing framework. Travis-CI and Sauce Labs links:
Test files are housed in another repo.
Running make init
will refresh the test_files
submodule and get the files.
make test
will run the nodejs-based tests. To run the in-browser tests, clone
the oss.sheetjs.com repo and
replace the xls.js file (then fire up the browser and go to stress.html
):
$ cp xls.js ../SheetJS.github.io
$ cd ../SheetJS.github.io
$ simplehttpserver # or "python -mSimpleHTTPServer" or "serve"
$ open -a Chromium.app http://localhost:8000/stress.html
For a much smaller test, run make test_misc
.
CFB
refers to the Microsoft Compound File Binary Format, a container format for XLS as well as DOC and other pre-OOXML data formats.
The mechanism is split into a CFB parser (which scans through the file and produces concrete data chunks) and a Workbook parser (which does excel-specific parsing). XML files are not processed by the CFB parser.
Due to the precarious nature of the Open Specifications Promise, it is very important to ensure code is cleanroom. Consult CONTRIBUTING.md
XLSX/XLSM/XLSB is available in js-xlsx.
Please consult the attached LICENSE file for details. All rights not explicitly granted by the Apache 2.0 license are reserved by the Original Author.
It is the opinion of the Original Author that this code conforms to the terms of the Microsoft Open Specifications Promise, falling under the same terms as OpenOffice (which is governed by the Apache License v2). Given the vagaries of the promise, the Original Author makes no legal claim that in fact end users are protected from future actions. It is highly recommended that, for commercial uses, you consult a lawyer before proceeding.
Certain features are shared with the Office Open XML File Formats, covered in:
ISO/IEC 29500:2012(E) "Information technology — Document description and processing languages — Office Open XML File Formats"
OSP-covered specifications:
FAQs
Excel 5.0/95 and 97-2004 spreadsheet (BIFF5 XLS / BIFF8 XLS / XML 2003) parser
The npm package xlsjs receives a total of 0 weekly downloads. As such, xlsjs popularity was classified as not popular.
We found that xlsjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.