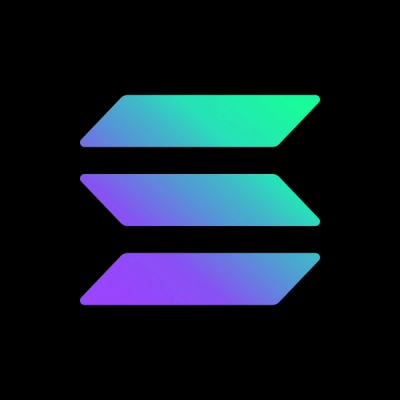
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
xml-sitemap
Advanced tools
Utilities for quickly making custom XML sitemaps
Add it to a project with npm
:
$ npm install xml-sitemap --save
Then just require
it in your project:
var XmlSitemap = require('xml-sitemap');
var sitemap = new XmlSitemap();
For more info on anything check out the docs.
All methods that don't return values return the sitemap, so you can chain them all together.
var sitemap = new XmlSitemap();
// add some urls
sitemap.add('http://domain.com/')
.add('http://domain.com/another-page', {
lastmod: 'now',
priority: 0.8
})
.add({
url: 'http://domain.com/sitemapz-rule',
changefreq: 'never',
lastmod: '1999-12-31'
});
We could have made the same sitemap with a single array:
var sitemap = new XmlSitemap()
.add([
'http://domain.com/',
{
url: 'http://domain.com/another-page',
lastmod: 'now',
priority: 0.8
},
{
loc: 'http://domain.com/sitemapz-rule',
changefreq: 'never',
lastmod: '1999-12-31'
}
])
Update and read some info from it:
// update some values
sitemap.update('http://domain.com/', new Date())
.setOptionValue('http://domain.com/sitemapz-rule', 'priority', 1);
// an array of the urls is in sitemap.urls
sitemap.urls
//=> [ 'http://domain.com/', 'http://domain.com/magic', 'http://domain.com/another-page', 'http://domain.com/sitemapz-rule' ]
// and the xml is in sitemap.xml
require('fs').writeFileSync('sitemap.xml', sitemap.xml)
// we can also read info from the sitemap
sitemap.hasUrl('http://domain.com/another-page');
//=> true
sitemap.getOptionValue('http://domain.com/', 'lastmod');
//=> {today's date!}
sitemap.getOptionValue('http://domain.com/magic', 'lastmod');
//=> {today's date!}
sitemap.getOptionValue('http://domain.com/another-page', 'lastmod');
//=> null
We can read in an existing sitemap (like the one we made above!) and modify it on the fly.
// get the XML as a string
var xmlString = require('fs').readFileSync('sitemap.xml');
// and pass it to the constructor
var sitemap = new XmlSitemap(xmlString);
sitemap.hasUrl('http://domain.com/magic');
//=> true
// modify it
sitemap.remove('http://domain.com/another-page')
.setOptionValues('http://domain.com/magic', {
lastmod: '2012-12-21',
priority: 0.9
});
sitemap.hasUrl('http://domain.com/another-page');
//=> false
sitemap.getOptionValue('http://domain.com/magic', 'priority');
//=> '0.9'
// quickly update lastmod vlaues
sitemap.getOptionValue('http://domain.com/magic', 'lastmod');
//=> '2012-12-21'
sitemap.update('http://domain.com/magic');
sitemap.getOptionValue('http://domain.com/magic', 'lastmod');
//=> {today's date}
For easily updating a url's lastmod, we can link a file to it. Here are a few ways to link the file:
var filename = 'path/to/file.html'; // last modified on '2012-12-21'
// link by adding a filename to the 'file' key to its options when adding it
sitemap.add({
url: 'http://domain.com/',
file: filename
});
// or after it is already in the sitemap
sitemap.linkFile('http://domain.com/', filename);
// the lastmod has been updated accordingly in both cases
sitemap.getOptionValue('http://domain.com/', 'lastmod');
//=> '2012-12-21'
// linked files are listed in sitemap.files
sitemap.files
//=> {'http://domain.com/': 'path/to/file.html'}
Linked files automatically update the lastmod of their respective urls when updateAll()
is called. So you can link many files to urls already in the sitemap by assigning the files object and then updating all.
sitemap.files = {
url1: filename1,
url2: filename2,
...
}
sitemap.updateAll();
To update a lastmod from a file without linking it, use sitemap.updateFromFile(url, filepath)
. Unlink a file with sitemap.unlinkFile(url)
.
An object representation of XML sitemaps and methods for editing them.
Kind: global class
Object
Array.<String>
Object
Array.<String>
Object
String
String
| null
XmlSitemap
XmlSitemap
String
| null
XmlSitemap
XmlSitemap
XmlSitemap
XmlSitemap
XmlSitemap
Bool
XmlSitemap
XmlSitemap
Object
Object
String
String
String
String
String
Create a new sitemap or create a sitemap object from an XML string.
Param | Type | Description |
---|---|---|
[xmlAsString] | String | Buffer | Optional, the sitemap XML as a string or Buffer. Must be a valid sitemap. |
Example
See Basic Usage above
Object
XML object tree of sitemap, as generated by xml2js. Automatically created and udated as the sitemap is modified.
Kind: instance property of XmlSitemap
Example
{
urlset: {
'$': { xmlns: 'http://www.sitemaps.org/schemas/sitemap/0.9' },
url: [
{ loc: 'http://domain.com/',
lastmod: '1985-12-25',
priority: '0.9' },
{ loc: 'http://domain.com/magic',
lastmod: '2012-12-21',
changefreq: 'never' }
]
}
}
Array.<String>
Array of urls in the sitemap. Add urls by using add.
Kind: instance property of XmlSitemap
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/')
.add('http://domain.com/magic');
console.log(sitemap.urls);
// [ 'http://domain.com/', 'http://domain.com/magic' ]
Object
Object of files linked to a url, for determining lastmod. To link a file use linkFile or pass the filename under the key file
in the initial options.
Kind: instance property of XmlSitemap
Array.<String>
Allowable option tags for urls in sitemap. Add options with or without handlers by using addOption.
Kind: instance property of XmlSitemap
Default: ['lastmod', 'changefreq', 'priority']
Example
var sitemap = new XmlSitemap();
console.log(sitemap.urlOptions);
// [ 'lastmod', 'changefreq', 'priority' ]
sitemap.addOption('foo');
console.log(sitemap.urlOptions);
// [ 'lastmod', 'changefreq', 'priority', 'foo' ]
Object
Optional handlers for custom option settings. Handler functions are listed under the option name key. Add options with addOption.
Kind: instance property of XmlSitemap
Default: {
lastmod: XmlSitemap.handleLastmod,
changefreq: XmlSitemap.handleChangefreq,
priority: XmlSitemap.handlePriority
}
String
Output the sitemap as XML.
Kind: instance property of XmlSitemap
Returns: String
- The sitemap XML
String
| null
Determine if and how to set an options given it's value. Will process option through handler if set in optionHandlers. Add options with addOption.
Kind: instance method of XmlSitemap
Returns: String
| null
- How the option will appear in the XML
Throws:
Error
Unavailable options throw an errorParam | Type | Description |
---|---|---|
option | String | The name of the option |
value | * | The object from which to derive the XML value |
XmlSitemap
Add a custom option tag to the allowed url tags. Adds the option and handler to urlOptions and optionHandlers.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The updated sitemap
Throws:
Error
Cannot add options that already exist without setting overwrite to true. They must be removed with removeOption.Param | Type | Default | Description |
---|---|---|---|
optionName | String | The name of the option | |
[handler] | function | An optional function to process option values for XML representation. Must return string. | |
[overwrite] | Bool | false | Acknowledge that you are overwriting the option if it already exists. This will remove all instances of the option in the sitemap. |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com.');
// before adding option
sitemap.setOptionValue('http://domain.com/', 'foo', 'bar');
// Error: Unrecognized option foo. Expected one of lastmod, changefreq, priority.
// adding option without handler
sitemap.addOption('foo');
sitemap.setOptionValue('http://domain.com/', 'foo', 'bar');
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// <foo>bar</foo>
// </url>
// </urlset>
// adding option with handler
sitemap.addOption('foo', function(str) {return str.toUpperCase();});
// Error: Option 'foo' already exists.
// using overwrite
sitemap.addOption('foo', function(str) {return str.toUpperCase();}, true);
sitemap.setOptionValue('http://domain.com/', 'foo', 'bar');
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// <foo>BAR</foo>
// </url>
// </urlset>
XmlSitemap
Remove an existing available option. Removes its handler if applicable and removes all instances of it in the sitemap's urls.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- - The updated sitemap
Param | Type | Description |
---|---|---|
optionName | String | The option to remove |
Example
var sitemap = new XmlSitemap()
.addOption('foo')
.add('http://domain.com/', {'foo': 'bar'});
// BEFORE
console.log(sitemap.urlOptions); // [ 'lastmod', 'changefreq', 'priority', 'foo' ]
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// <foo>bar</foo>
// </url>
// </urlset>
sitemap.removeOption('foo');
// AFTER
console.log(sitemap.urlOptions); // [ 'lastmod', 'changefreq', 'priority' ]
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// </url>
// </urlset>
String
| null
Get an option value from the XML object tree's url. Valid options that are unset return null. Invalid options or requesting options for urls not in the sitemap throw errors.
Kind: instance method of XmlSitemap
Returns: String
| null
- The option value or null if option not assigned or url isn't in tree.
Throws:
Error
Url must be in sitemapError
Option must be valid, add options with addOptionParam | Type | Description |
---|---|---|
url | String | The url to get the option for |
option | String | The desired option |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/', {priority: 0.7});
sitemap.getOptionValue('http://domain.com/', 'priority'); // '0.7'
sitemap.getOptionValue('http://domain.com/', 'lastmod'); // null
sitemap.getOptionValue('http://notreal.com/', 'lastmod'); // throws error
XmlSitemap
Set an option on a url node. Set multiple options using XmlSitemap#updateUrl. To remove an option, set its value to null or use XmlSitemap#removeOptionValue.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The updated XmlSitemap
Param | Type | Description |
---|---|---|
url | string | Url in Sitemap to update |
option | string | The name of the option |
value | * | The object from which to derive the XML value |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/');
sitemap.setOptionValue('http://domain.com/', 'priority', 0.3);
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// <priority>0.3</priority>
// </url>
// </urlset>
XmlSitemap
Update a url's options. Options must be in the sitemap's allowable urlOptions. Not to be confused with setOptionValue To remove an existing option, set its value to null.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The updated sitemap object
Throws:
Error
Options object is requiredParam | Type | Description |
---|---|---|
url | String | The url to add |
options | Object | Options for setting various information about the url |
Example
var url = 'http://domain.com/'
var sitemap = new XmlSitemap()
.add(url, {lastmod:'1900-10-31', priority: 0.7});
sitemap.getOptionValue(url, 'lastmod'); // '1900-10-31'
sitemap.getOptionValue(url, 'priority'); // '0.7'
sitemap.getOptionValue(url, 'changefreq'); // null
sitemap.setOptionValues(url, {priority: 0.8, changefreq: 'weekly'});
sitemap.getOptionValue(url, 'lastmod'); // '1900-10-31'
sitemap.getOptionValue(url, 'priority'); // '0.8'
sitemap.getOptionValue(url, 'changefreq'); // 'weekly'
XmlSitemap
Update when a url was last modified. Aliased with updateLastmod
. Updates lastmod date to today if no date is passed unless there's a linked file. If there's a linked file, it will use that date regardless. To link a file, use linkFile.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The sitemap object
Param | Type | Default | Description |
---|---|---|---|
url | String | Url in Sitemap to update | |
[date] | String | Date | today | Date to update the lastmod value to. |
Example
var url = 'http://domain.com/'
var sitemap = new XmlSitemap()
.add(url);
sitemap.getOptionValue(url, 'lastmod'); // null
// no date
sitemap.update(url);
sitemap.getOptionValue(url, 'lastmod'); // {today's date}
// string date
sitemap.update(url, '2012-12-21');
sitemap.getOptionValue(url, 'lastmod'); // '2012-12-21'
// 'now'
sitemap.update(url, 'now');
sitemap.getOptionValue(url, 'lastmod'); // {today's date}
// Date object
var date = new Date();
date.setFullYear(2012);
date.setMonth(11);
date.setDate(21);
sitemap.update(url, date);
sitemap.getOptionValue(url, 'lastmod'); // '2012-12-21'
XmlSitemap
Update the lastmod of a url from a file's lastmod without linking the file.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The updated sitemap
Throws:
Error
Unable to resolve filepathParam | Type | Description |
---|---|---|
url | String | The url to link a file to |
filepath | String | The path to the file |
Example
// suppose 'file.html' was last modified 2016-01-01
var url = 'http://domain.com/';
var sitemap = new XmlSitemap()
.add(url);
sitemap.getOptionValue(url, 'lastmod');
//=> null
sitemap.updateFromFile(url, 'file.html');
sitemap.getOptionValue(url, 'lastmod');
//=> 2016-01-01
// there are still no linked files in the files object
sitemap.files
//=> {}
XmlSitemap
Link a file to a url. Their lastmod dates will sync. Update lastmod values that have linked files by calling XmlSitemap#updateAll. To unlink files use XmlSitemap#unlinkFile. Update lastmod from a file without linking the file using updateFromFile.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The updated XmlSitemap
Throws:
Error
Unable to resolve filepathParam | Type | Description |
---|---|---|
url | String | The url to link a file to |
filepath | String | The path to the file |
Example
// suppose 'file.html' was last modified 2016-01-01
var url = 'http://domain.com/';
var sitemap = new XmlSitemap()
.add(url);
sitemap.getOptionValue(url, 'lastmod');
//=> null
sitemap.linkFile(url, 'file.html');
sitemap.getOptionValue(url, 'lastmod');
//=> 2016-01-01
// the file has been linked and the lastmod for the url will be updated
// everytime sitemap.updateAll() is called.
sitemap.files
//=> {'http://domain.com/': 'file.html'}
Bool
Check if sitemap contains a url.
Kind: instance method of XmlSitemap
Returns: Bool
- Whether the url is in the sitemap
Param | Type | Description |
---|---|---|
url | String | The url to look for |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/');
sitemap.hasUrl('http://domain.com/'); // true
sitemap.hasUrl('http://otherdomain.com/'); // false
XmlSitemap
There are a few ways to add a urls:
url
or loc
attribute and optionsAliased with addUrl
or addUrls
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The sitemap object
Throws:
Error
Url object must contain a url
or loc
propertyError
Url is already in sitemapTypeError
Url must be object, string, or arrayParam | Type | Description |
---|---|---|
url | String | Object | Array | The url(s) to add |
[urlOptions] | Object | Options for setting various information about the url. Options must be in the objects urlOptions. |
Example
var sitemap = new XmlSitemap();
.add('http://domain.com/')
.add('http://domain.com/other', {lastmod: '2012-11-21'})
.add({
url: 'http://domain.com/magic',
priority: 0.7
})
.add({
loc: 'http://domain.com/another-page',
changefreq: 'never'
});
// which makes the same sitemap as:
var sitemap = new XmlSitemap()
.add([
'http://domain.com/',
{
url: 'http://domain.com/other',
lastmod: '2012-11-21'
},
{
url: 'http://domain.com/magic'
priority: 0.7
},
{
loc: 'http://domain.com/another-page',
changefreq: 'never'
}
];
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// </url>
// <url>
// <loc>http://domain.com/other</loc>
// <lastmod>2012-11-21</lastmod>
// </url>
// <url>
// <loc>http://domain.com/magic</loc>
// <priority>0.7</priority>
// </url>
// <url>
// <loc>http://domain.com/another-page</loc>
// <changefreq>never</changefreq>
// </url>
// </urlset>
XmlSitemap
Remove url from the Sitemap. Aliased with removeUrl
.
Kind: instance method of XmlSitemap
Returns: XmlSitemap
- The sitemap object
Param | Type | Description |
---|---|---|
url | String | The url to remove |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/')
.add('http://domain.com/other');
// BEFORE
console.log(sitemap.urls); // ['http://domain.com/', 'http://domain.com/other']
sitemap.hasUrl('http://domain.com/other'); // true
sitemap.remove('http://domain.com/other');
// AFTER
console.log(sitemap.urls); // ['http://domain.com/']
console.log(sitemap.xml);
// <?xml version="1.0" encoding="UTF-8"?>
// <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">
// <url>
// <loc>http://domain.com/</loc>
// </url>
// </urlset>
Object
Get node of the XML object tree for a url.
Kind: instance method of XmlSitemap
Returns: Object
- The XML element of the url
Param | Type | Description |
---|---|---|
url | String | The url of the desired XML node |
Example
var sitemap = new XmlSitemap()
.add('http://domain.com/', {lastmod: '2012-12-21', changefreq: 'never'});
sitemap.getUrlNode('http://domain.com/');
// { loc: 'http://domain.com/',
// lastmod: '2012-12-21',
// changefreq: 'never' }
Object
Create a url node XML object
Kind: instance method of XmlSitemap
Returns: Object
- The url XML node
Param | Type | Description |
---|---|---|
url | String | The url to add |
[options] | Object | Options for setting various information about the url, must be one of urlOptions. Add options with addOption. |
String
Format a Date object to W3 standard string.
Kind: static method of XmlSitemap
Returns: String
- Date formatted as year-month-day
Throws:
TypeError
Argument must be Date objectParam | Type | Description |
---|---|---|
date | Date | the Date to format |
Example
var date = new Date();
date.setFullYear(2012);
date.setMonth(11);
date.setDate(21);
XmlSitemap.w3Date(date); // '2012-12-21'
String
Determine what to put in the lastmod field given the input. Will NOT check format of string.
Kind: static method of XmlSitemap
Returns: String
- The value of lastmod
Param | Type | Description |
---|---|---|
[date] | String | Date | The lastmod value as a Date object, the string 'now', or a string (will not confirm format) |
String
Check if changfreq value is valid.
Kind: static method of XmlSitemap
Returns: String
- A valid changefreq value
Throws:
Error
The changefreq must be valid. Allowed values are "always", "hourly", "daily", "weekly", "monthly", "yearly", "never".Param | Type | Description |
---|---|---|
value | String | The changefreq value to check |
String
Check for valid priority value and return it as a string. Priority must be between 0 and 1.
Kind: static method of XmlSitemap
Returns: String
- The priority value as a string
Throws:
Error
Priority must be number between 0 & 1, inclusive.Param | Type | Description |
---|---|---|
value | Double | The Priority value |
String
Get W3-standard formatted date string from file's last modified time.
Kind: static method of XmlSitemap
Returns: String
- W3-standard formatted date string
Throws:
Error
Unable to resolve filepathParam | Type | Description |
---|---|---|
filepath | String | The path to the file |
MIT © 2016 Robert Pirtle
FAQs
Utilities for quickly writing XML sitemaps
We found that xml-sitemap demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.