Youtube API
A Node.JS module, which provides an object oriented wrapper for the Youtube v3 API.

Installation
Install with the Node.JS package manager npm:
$ npm install youtube-api
or
Install via git
:
$ git clone git://github.com/IonicaBizau/youtube-api.git
$ cd youtube-api
$ npm install
Documentation
The official Youtube documentation is a very useful resource.
If you have any questions, just open an issue.
Example
var Youtube = require("youtube-api");
Youtube.authenticate({
type: "oauth"
, token: ACCESS_TOKEN
});
Youtube.channels.list({
"part": "id"
, "mySubscribers": true
, "maxResults": 50
}, function (err, data) {
console.log(err || data);
});
Authentication
OAuth
Youtube.authenticate({
type: "oauth"
, token: "your access token"
});
Server Key
Youtube.authenticate({
type: "key"
, key: "your server key"
});
JWT
Youtube.authenticate({
type: "jwt"
, email: "77....3vv@developer.gserviceaccount.com"
, keyFile: "... auth.pem"
, key: "fb....d50"
, subject: "you@gmail.com"
, scopes: ["https://www.googleapis.com/auth/youtube"]
}).authorize(function (err, data) {
if (err) { throw err; }
});
Running the Tests
Download and test this module using this test application.
Note that a connection to the internet is required to run the tests.
Contributors
See package.json file.
Donate
Help the youtube-api
NPM package development. Any donation is welcome and I will be thankful!
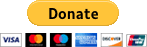
LICENSE
MIT license. See the LICENSE file for details.
Changelog
v0.3.1
v0.3.0
- JWT authentication type
- All request types are supported (using
googleapis
) - Deprecacted
setConfig
method
v0.2.2
- Removed debugging message from index.js
- Output an error in application logs if there is an unkwnown error in request
- Minor fix in util.js
v0.2.1
v0.2.0
v0.1.1
- Fixed #2 (pull request): fix in
videos
API requests.
v0.1.0
- Initial release
- Supports only
GET
requests