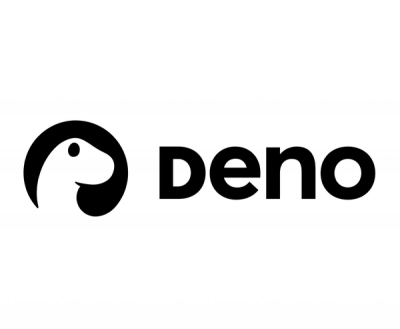
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
[!WARNING] This is beta version, not recomended to use in production. Join whatsapp community for latest info WhatsApp Channel
Zaileys is a powerful and flexible WhatsApp API library for Node.js, built on top of Baileys. It simplifies the process of integrating WhatsApp functionalities into your applications, providing a comprehensive set of features for building robust and scalable WhatsApp-based solutions.
npm add zaileys
pnpm add zaileys
yarn add zaileys
bun add zaileys
deno add npm:zaileys
[!TIP] If you don't want to take time for setup and configuration, use the example.ts file that I have provided.
// ESM
import { Client } from "zaileys";
// CJS
const { Client } = require("zaileys");
[!WARNING] Warning! in beta version this library uses built-in Baileys
makeInMemoryStore
function which will most likely use quite a lot of RAM. If you experience this, you can delete the.zaileys/memory.json
file then restart the server.
const wa = new Client({
prefix: "/", // for command message, example '/'
ignoreMe: true, // ignore messages from bot (bot phone number)
phoneNumber: 628xxx, // fill bot phone number if auth type is 'pairing'
authPath: ".zaileys", // auth directory path for session and chat store
authType: "pairing", // auth type 'pairing' or 'qr'
showLogs: true, // show logs of any chats
autoMentions: true, // bot will be auto mentioned if text contains sender number with '@' prefix
autoOnline: true, // bot status will be mark online
autoRead: true, // auto read message from any chats
autoRejectCall: true, // auto reject call if someone call you
citation: {
// your citation will be boolean object based on validate with your value
// system will be validate your value with 'senderId' and 'roomId'
// if one is valid then the key will return 'boolean'
// sample output: { isAuthors: boolean }
// just sample, you can rename with any key
authors: () => ["628xxxx"], // key 'authors' will be 'isAuthors'
myGroups: () => ["1203633xxxxx"], // key 'myGroups' will be 'isMyGroups'
...otherKey // key 'otherKey' will be 'isOtherKey'
},
});
[!NOTE] The functions and parameters below may change at any time considering that this library is a beta version. So when you update a library to the latest version but an error occurs, there may be changes to certain functions and parameters.
You can find out the output type of each object in the listener below:
connection
message
command
call
wa.on("connection", (ctx) => {}); // listener for current connection
wa.on("message", (ctx) => {}); // listener for message from any chats
wa.on("command", (ctx) => {}); // listener for message that starts with prefix at beginning of word
wa.on("call", (ctx) => {}); // listener for someone call to bot
wa.on("connection", (ctx) => {
if (ctx.status == "open") {
// do something
}
});
Here you can find out the complete parameters for the .sendText()
function
wa.on("message", (ctx) => {
if (ctx.text == "ping") {
wa.sendText("Hello! " + ctx.senderName);
}
// text from reply message
if (ctx.reply?.text == "ping") {
wa.sendText("Pong from reply!");
}
// text from nested reply message
// you can retrieve reply messages of any depth
if (ctx.reply?.reply?.reply?.text == "ping") {
wa.sendText("Pong from nested reply!");
}
// text with footer message (doesn't work on whatsapp desktop)
if (ctx.text == "pong") {
wa.sendText("Ping!", { footer: "Footer message" });
}
});
Here you can find out the complete parameters for the .sendReply()
function
wa.on("message", (ctx) => {
if (ctx.text == "ping") {
wa.sendReply("Pong!");
}
// reply with footer message (doesn't work on whatsapp desktop)
if (ctx.text == "pong") {
wa.sendReply("Ping!", { footer: "Footer message" });
}
// reply with fake verified badge
if (ctx.text == "fake") {
wa.sendReply("Fake Verified!", { fakeVerified: "whatsapp" });
}
});
Here you can find out all the verified platforms provided
Here you can find out the complete parameters for the .sendSticker()
function
wa.on("message", async (ctx) => {
if (ctx.chatType == "sticker") {
const sticker = await ctx.media?.buffer!();
wa.sendSticker(sticker);
}
if (ctx.text == "sticker") {
wa.sendSticker("https://gtihub.com/zeative.png");
}
});
Here you can find out the complete parameters for the .sendImage()
function
wa.on("message", async (ctx) => {
if (ctx.chatType == "image") {
const image = await ctx.media?.buffer!();
wa.sendImage(image);
}
if (ctx.text == "image") {
wa.sendImage("https://gtihub.com/zeative.png");
}
if (ctx.text == "mypp") {
const picture = await ctx.senderImage();
wa.sendImage(picture);
}
});
Here you can find out the complete parameters for the .sendVideo()
function
wa.on("message", async (ctx) => {
if (ctx.chatType == "video") {
const video = await ctx.media?.buffer!();
wa.sendVideo(video);
}
if (ctx.text == "video") {
wa.sendVideo("https://gtihub.com/zeative.png");
}
});
Here you can find out the complete parameters for the .sendAudio()
function
wa.on("message", async (ctx) => {
if (ctx.chatType == "audio") {
const audio = await ctx.media?.buffer!();
wa.sendAudio(audio);
}
if (ctx.text == "audio") {
wa.sendAudio("https://gtihub.com/zeative.png");
}
});
[!NOTE] You must set
prefix
option to anything character
wa.on("message", async (ctx) => {
// for example set prefix to "/"
// and user text "/test"
if (ctx.command == "test") {
wa.sendText("From command message!");
}
});
[!NOTE] You must set
autoMentions
option totrue
and bot will send text as mentions
wa.on("message", async (ctx) => {
if (ctx.text == "mentions") {
wa.sendText("Here user mentioned: @0 @18002428478");
// example output: "Here user mentioned: @WhatsApp @ChatGPT"
// if `autoMentions` is inactive or `false`
// output can be plain text: "Here user mentioned: @0 @18002428478"
}
});
[!NOTE] You must set
citation
like example above before
const wa = new Client({
...,
// just example you can edit with your own
citation: {
authors: () => ["628xxxx"],
myPrivateGroups: () => ["1203633xxxxx"],
bannedUsers: async () => {
const res = await fetch("/get/user/banned")
const users = await res.json()
return users // ["628xx", "628xx", "628xx"]
}
}
})
wa.on("message", async (ctx) => {
const isAuthors = ctx.citation?.isAuthors;
const isMyPrivateGroups = ctx.citation?.isMyPrivateGroups;
const isBannedUsers = ctx.citation?.isBannedUsers;
if (isAuthors && ctx.text == "test1") {
wa.sendText("Message for my author: kejaa");
}
if (isMyPrivateGroups && ctx.text == "test2") {
wa.sendText("Message for my private group!");
}
if (isBannedUsers && ctx.text) {
wa.sendText("Your number is banned!");
}
});
Contributions are welcome! Please follow these steps to contribute:
git checkout -b feature/your-feature-name
or git checkout -b fix/bug-description
).git commit -m 'Add some AmazingFeature'
).git push origin feature/your-feature-name
).Please ensure your code follows the project's coding standards and includes appropriate tests.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Zaileys - Simplify Typescript/Javascript WhatsApp NodeJS API
The npm package zaileys receives a total of 56 weekly downloads. As such, zaileys popularity was classified as not popular.
We found that zaileys demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.