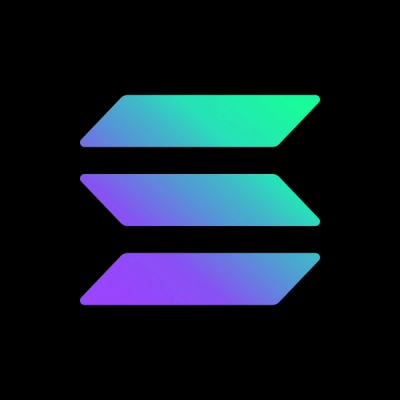
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
I took it upon myself to rewrite the original node module developed by the creators of ZeroPi, which can be found here.
The function names are the same, and so are the arguments passed to them. I just felt like the original code needed 'a bit of work'.
You will need the following:
The rest is up to you, I bought a Nema 23 Stepper motor and a stepper motor driver online to start me off.
You will need the following:
I will include the process I went through to get it all working, as I had a lot of trial and error. Hopefully it will help someone out in the same situation.
I followed the steps shown here
When you first log in you will need to stop Raspbian using the serial ports, so they are free for you to use.
sudo raspi-config
You will need to restart for the settings to take effect. Once rebooted you will have full control over the serial ports.
The ZeroPi requires firmware to be uploaded to it via the Arduino IDE. I had to do this bit on windows, because I couldn't get the board connected on Mac OSX (El Capitan).
Follow the steps shown here
Follow the steps shown here
Once you have completed the setup of the Raspberry Pi and the ZeroPi has it's firmware uploaded, you can connect them together.
If you have your own way of setting up node feel free to do it your way. I did the following:
sudo apt-get install npm
sudo npm install n -g
n latest
This should download and install the latest version of node. To check: node --version
(as of today that will be 6.0.0)
This will detail the setup of your application. If you know how to do this already, skip to Basic Usage.
mkdir MyApp
cd MyApp
npm init
zeropi
module: npm install zeropi --save
(this will save the reference to the module in your package.json file)npm install zeropi --save
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// The serial port has been opened successfully
// We can do the business in here
zeropi.stepperRun(0, 1000);
});
Note: you must always call the main methods after the serial port has been opened. Doing it before will have no effect
Devices work differently based on what you are targeting:
The methods mirror the original module exactly. I will list them here for convenience.
digitalWrite(pin, level)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Turn the blue LED off
zeropi.digitalWrite(13, 0);
// Turn it back on after 5 seconds
setTimeout(() => zeropi.digitalWrite(13, 1), 5000);
});
pwmWrite(pin, pwm)
See here for an explanation of PWM
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Use pwm to flash the led
zeropi.digitalWrite(13, 64);
});
digitalRead(pin, callback)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Get the level of pin 13
zeropi.digitalRead(13, level => {
console.log('Read: ', level);
});
});
analogRead(pin, callback)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Get the level of pin 1
zeropi.analogRead(1, level => {
console.log('Read: ', level);
});
});
dcMotorRun(device, pwm)
See here for an explanation of PWM
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Run the motor on slot 1 at 25% Duty Cycle
zeropi.dcMotorRun(0, 64);
});
dcMotorStop(device)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Stop DC motor in slot 1
zeropi.dcMotorStop(0);
});
stepperRun(device, speed)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Run stepper motor continuosly on slot 1 at speed 1000
zeropi.stepperRun(0, 1000);
});
stepperMove(device, distance, speed, callback)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Move stepper motor in slot 1 1000 steps at speed 1000
zeropi.stepperMove(0, 1000, 1000, () => {
console.log('Stepper motor move complete');
});
});
stepperMoveTo(device, position, speed, callback)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Move stepper motor in slot 1 to position 1000 steps at speed 1000
zeropi.stepperMoveTo(0, 1000, 1000, () => {
console.log('Stepper motor move to complete');
});
});
stepperStop(device)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Stop stepper motor in slot 1
zeropi.stepperStop(0);
});
steppersEnable()
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Enable all stepper motors
zeropi.steppersEnable();
});
steppersDisable()
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Disable all stepper motors
zeropi.steppersDisable();
});
stepperSetting(device, microstep, acceleration)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Set settings for stepper motor in slot 1
zeropi.stepperSetting(0, 2, 1000);
});
servoRun(device, angle)
const ZeroPi = require('zeropi');
const zeropi = new ZeroPi();
zeropi.onOpen(() => {
// Move servo on pins A0 to 90 degrees
zeropi.servoRun(0, 90);
});
At a stretch I am a beginner when it comes to electronics, I am a software developer by trade. Most of my information has been gleaned via Google searches... So I welcome feedback.
If you want to make a contribution to improve any aspect of this node module please feel free to put up a pull request. I will try to keep the module as up to date as possible.
FAQs
A node 5.0.0+ module for running a zeropi
We found that zeropi demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.