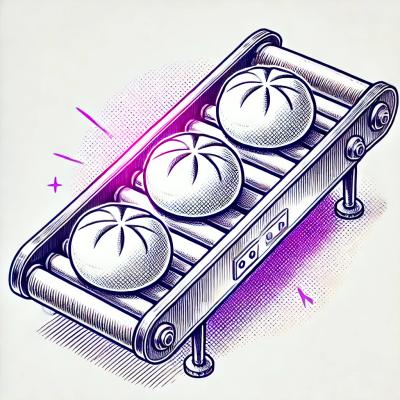
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
The official ZKpay Javascript SDK by Space and time labs.
library designed to interact with ZKpay contracts on testnet. It provides methods to buy credit with native tokens and ERC-20 tokens, manage token approvals, and handle authentication.
To install ZKpay, use npm or yarn:
npm install zkpay
or
yarn add zkpay
Initiate ZKpay instance:
import { ZKpay, ethers } from "zkpay";
const main = async () => {
const provider = new ethers.JsonRpcProvider('https://sepolia.infura.io/v3/');
const signer = new ethers.Wallet(privateKey, provider);
// Define the chain ID (e.g., 11155111 for sepolia)
const zkpay = new ZKpay(signer, 11155111); // sepolia
try {
const tx = await zkpay.buyWithNative(20n, '0x3077Bf667dBD81d3c718684Da4DE4Dc8448220E1', 0); // 20 wei
const txReceipt = await tx.wait();
} catch (e) {
console.log("error:", e);
}
};
main();
Here's an example of how to use the ZKpay sdk with ethers v5 lib:
using Ethers v6:
import { ZKpay } from "zkpay";
import { ethers } from 'ethers';
const provider = new ethers.BrowserProvider(window.ethereum, { name: 'sepolia', chainId: 11155111 });
const zkpay = new ZKpay(provider, 11155111);
await zkpay.buyWithNative(33, '0x3077Bf667dBD81d3c718684Da4DE4Dc8448220E1', 0)
ZKpay sdk also export ethers v6 instance:
import { ZKpay, SupportedChain ,ethers} from "zkpay";
const provider = new ethers.JsonRpcProvider("YOUR_RPC_URL");
const signer = provider.getSigner();
// Define the chain ID
const chainId: SupportedChain = 11155111;
// Instantiate the ZKpay class
const zkpay = new ZKpay(signer, chainId);
// Use the ZKpay instance to interact with ZKpay contracts
async function main() {
const tokenContract = await zkpay.getTokenContract("usdc");
console.log("Token contract address:", tokenContract.address);
const buyTx = await zkpay.buyWithNative(BigInt("1000000000000000000"), "0xRecipientAddress");
console.log("buyWithNative transaction:", buyTx);
}
main().catch(console.error);
yarn
yarn run build
Node js version of the files will be generated to lib
async getZKpayContract(): Promise<ethers.Contract>
Returns an instance of the ZKpay contract.
getTokenAddress(token: TokenName): string
Returns the address of a specified token.
token: The name of the token. Valid names are "usdc"
getTokenListsWithMetadata(): TokenMetadataType[]
Returns a list of tokens with their metadata.
async getTokenContract(token: TokenName): Promise<ethers.Contract>
Returns an instance of the specified token's contract.
token: The name of the token.
async buyWithNative(
amountInWei: bigint,
onBehalfOf: string
): Promise<any>
buy compute credit with native tokens (ETH) into the ZKpay contract.
amountInWei: The amount to deposit, in wei. onBehalfOf: The address on whose behalf the buy is made.
async approve(token: TokenName, amount: bigint): Promise<any>
Approves the ZKpay contract to spend a specified amount of a token.
token: The name of the token. amount: The amount to approve.
async revokeApprove(token: TokenName): Promise<any>
Revokes the approval for the ZKpay contract to spend a specified token.
token: The name of the token.
async allowance(token: TokenName): Promise<any>
get the allowed amount for the ZKpay contract to spend on specified token.
token: The name of the token.
async buy(
token: TokenName,
amount: bigint,
onBehalfOf: string
): Promise<any>
buy credit with ERC-20 tokens.
token: The name of the token. amount: The ERC20 amount. onBehalfOf: The address on whose behalf the buy is made.
To contribute to the development of ZKpay, clone the repository and install the dependencies:
git clone https://github.com/spaceandtimelabs/zkt-sdk-js.git
cd zkpay
npm install
We welcome contributions to zkt-sdk. Please open an issue or submit a pull request on GitHub.
ZKpay is released under the MIT License. See the LICENSE file for more details.
FAQs
The official ZKpay Javascript sdk
We found that zkpay demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.