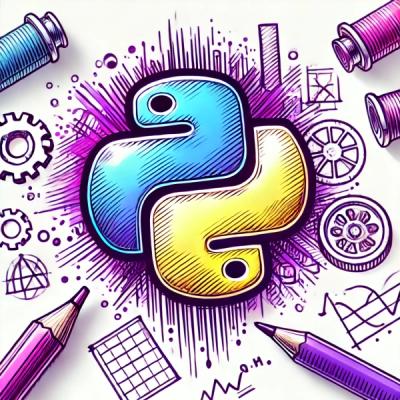
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
zkToken | JS SDK
library designed to interact with zkETH contracts on Ethereum and other supported blockchains. It provides methods to deposit native tokens and ERC-20 tokens, manage token approvals, and handle authentication.
To install ZkETH, use npm or yarn:
npm install zketh
or
yarn add zketh
Initiate zketh instance:
import { ZkEth, ethers } from "zkt-sdk";
const main = async () => {
const provider = new ethers.JsonRpcProvider('https://ethereum-holesky.publicnode.com');
const signer = new ethers.Wallet(privateKey, provider);
// Define the chain ID (e.g., 17000 for holesky)
const zkEth = new ZkEth(signer, 17000); // holesky
try {
const tx = await zkEth.depositNative(20n, '0x3077Bf667dBD81d3c718684Da4DE4Dc8448220E1', 0); // 20 wei
const txReceipt = await tx.wait();
} catch (e) {
console.log("error:", e);
}
console.log('venu!');
};
main();
Here's an example of how to use the zkt-lib library with ethers v5 lib:
using Ethers v6:
import { ZkEth } from "zkt-sdk";
import { ethers } from 'ethers';
const provider = new ethers.BrowserProvider(window.ethereum, { name: 'polygon', chainId: 137 });
const zkEth = new ZkEth(provider, 137);
await zkEth.depositNative(33, '0x3077Bf667dBD81d3c718684Da4DE4Dc8448220E1', 0)
zkt-sdk also export ethers v6 instance:
import { ZkEth, SupportedChain ,ethers} from "zketh";
const provider = new ethers.JsonRpcProvider("YOUR_RPC_URL");
const signer = provider.getSigner();
// Define the chain ID (1 for Ethereum mainnet)
const chainId: SupportedChain = 1;
// Instantiate the ZkEth class
const zkEth = new ZkEth(signer, chainId);
// Use the ZkEth instance to interact with zkETH contracts
async function main() {
const tokenContract = await zkEth.getTokenContract("usdt");
console.log("Token contract address:", tokenContract.address);
const depositTx = await zkEth.depositNative(BigInt("1000000000000000000"), "0xRecipientAddress");
console.log("Deposit transaction:", depositTx);
}
main().catch(console.error);
yarn
yarn run build
Node js version of the files will be generated to lib
async getZkETHContract(): Promise<ethers.Contract>
Returns an instance of the zkETH contract.
getTokenAddress(token: TokenName): string
Returns the address of a specified token.
token: The name of the token. Valid names are "usdt", "usdc", "link", "wsteth".
getTokenListsWithMetadata(): TokenMetadataType[]
Returns a list of tokens with their metadata.
async getTokenContract(token: TokenName): Promise<ethers.Contract>
Returns an instance of the specified token's contract.
token: The name of the token.
async depositNative(
amountInWei: bigint,
onBehalfOf: string
): Promise<any>
Deposits native tokens (ETH/MATIC) into the zkETH contract.
amountInWei: The amount to deposit, in wei. onBehalfOf: The address on whose behalf the deposit is made.
async approve(token: TokenName, amount: bigint): Promise<any>
Approves the zkETH contract to spend a specified amount of a token.
token: The name of the token. amount: The amount to approve.
async revokeApprove(token: TokenName): Promise<any>
Revokes the approval for the zkETH contract to spend a specified token.
token: The name of the token.
async allowance(token: TokenName): Promise<any>
get the allowed amount for the zkETH contract to spend on specified token.
token: The name of the token.
async depositTokens(
token: TokenName,
amount: bigint,
onBehalfOf: string
): Promise<any>
Deposits ERC-20 tokens into the zkETH contract.
token: The name of the token. amount: The amount to deposit. onBehalfOf: The address on whose behalf the deposit is made.
To contribute to the development of ZkETH, clone the repository and install the dependencies:
git clone https://github.com/spaceandtimelabs/zkt-sdk-js.git
cd zketh
npm install
We welcome contributions to zkt-sdk. Please open an issue or submit a pull request on GitHub.
ZkETH is released under the MIT License. See the LICENSE file for more details.
FAQs
zkToken | JS SDK
We found that zkt-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.