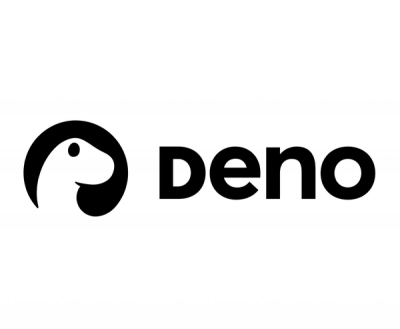
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
zoxios-nock
Advanced tools
A testing package that returns a nock scope when provided a zoxios request-maker.
npm i zoxios axios zod
npm i zoxios-nock
Parsing request's query and response's body.
import { zoxios } from 'zoxios';
import { zoxiosNock } from 'zoxios-nock';
describe('when provided a GET request', () => {
const requestMaker = zoxios('http://google.com')
.method('get')
.concatPath('api')
.responseSchema(
z.object({ name: z.string(), age: z.number().min(0).max(120) }),
);
it('should return GET scope', async () => {
// Providing zoxiosNock a requestMaker from zoxios.
const scope = zoxiosNock(getRequestMaker);
const response = await getRequestMaker.exec();
expect(response).toMatchObject({
name: expect.any(String),
age: expect.any(Number),
});
expect(scope.isDone()).toBeTruthy();
});
});
FAQs
Nock creation using zoxios definitions
The npm package zoxios-nock receives a total of 0 weekly downloads. As such, zoxios-nock popularity was classified as not popular.
We found that zoxios-nock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.