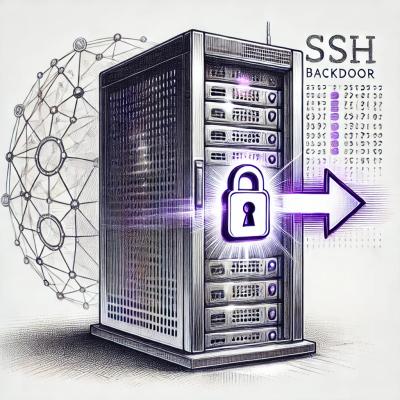
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Tools to simplify using face detection and ArUco recognition with PIL image objects
Tools to simplify using face detection and ArUco recognition with PIL image objects
This brings a range of CV2 image functionlaity into a form that is easy to use with PIL Image objects. The module was created to allow me to introduce image based functionality to my students while keeping things as simple as possible (switching between PIL and CV2 was an unnecessary complication I felt).
Key functions currently provided are:
A basic example
camera = ImageTools.Camera()
img = camera.take_photo()
img.save("my photo.png", "png")
img.show()
For the face detection function to work, you must supply a file path to a haarcascade file. You can obtain the relevant file from https://github.com/opencv/opencv/tree/master/data/haarcascades
The ImageTools.get_faces()
function will return a list of lists. The outer list represents the number of faces seen, where as the inner list represent the coordinates for an individual face being the [left, top, width, height]
pixel values.
To get the coordinates of each face in an image
from PIL import Image
import ImageTools
# ...
camera = ImageTools.Camera()
img = camera.take_photo()
faces = ImageTools.get_faces(img, "haarcascade_frontalface_default.xml")
print(faces)
To obtain seperate jpg image for each face detected
from PIL import Image
import ImageTools
# ...
counter = 0
camera = ImageTools.Camera()
img = camera.take_photo()
faces = ImageTools.get_faces(img, "haarcascade_frontalface_default.xml")
for a_face in faces: # for each individual face in the list of faces...
x,y,w,h = a_face # extract the left, top, width and height locations of a face
a_face_img = img.crop((x,y,x+w,y+h))
a_face_img.save(f"face_{counter:2}.jpg", "jpg")
a_face_img.show()
counter = counter + 1
To use a drawing tool to put rectangles highlighting the faces found in the original image...
from PIL import Image, ImageDraw
import ImageTools
# ...
camera = ImageTools.Camera()
img = camera.take_photo()
draw = ImageDraw.Draw(img) # create the drawing object
faces = ImageTools.get_faces(img, "haarcascade_frontalface_default.xml")
for a_face in faces: # for each individual face in the list of faces...
x,y,w,h = a_face # extract the left, top, width and height locations of a face
draw.rectangle((x,y,x+w,y+h), outline="#ffff00", width=5) # draw a rectangle around the face
# show the final image, highlighting each face
img.show()
The ImageTools.get_aruco()
function when provided a PIL Image object as a parameter, will return a Python list of the ArUco markers it detected within the image.
from PIL import Image
import ImageTools
# ....
camera = ImageTools.Camera()
image = camera.take_photo()
markers = ImageTools.get_aruco(image)
if 70 in markers:
print("I saw ArUco marker 70")
if 71 in markers:
print("I saw ArUco marker 71")
if 72 in markers:
print("I saw ArUco marker 72")
camera_got_image
function if you wishpip install ImageToolsMadeEAsy
These should all be installed for you automatically, so are just provided for informational purposes.
opencv-contrib-python
numpy
PIL
Paul Baumgarten 2019 @ https://github.com/paulbaumgarten/ImageToolsMadeEasy
FAQs
Tools to simplify using face detection and ArUco recognition with PIL image objects
We found that ImageToolsMadeEasy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.