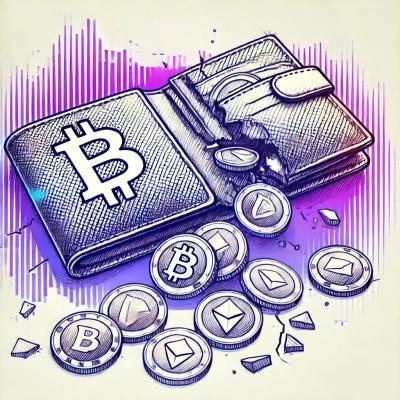
Security News
Bybit Hack Puts Crypto Losses at $1.6B, Surpassing All of Last Year in Just Two Months
Bybit's $1.46B hack by North Korea's Lazarus Group pushes 2025 crypto losses to $1.6B in just two months, already surpassing all of 2024's $1.49B total.