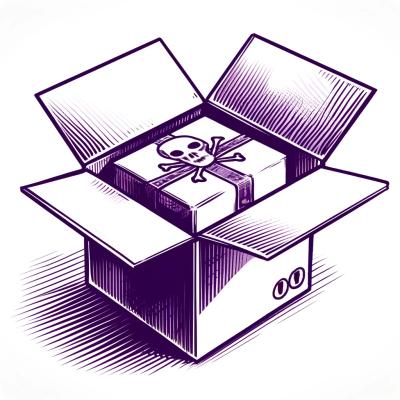
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
bk-crypto-python-sdk
Advanced tools
bk-crypto-python-sdk is a lightweight cryptography toolkit for Python applications based on Cryptodome / tongsuopy and other encryption libraries.
️🔧 BlueKing crypto-python-sdk 是一个基于 pyCryptodome / tongsuopy 等加密库的轻量级密码学工具包,为 Python 应用统一的加解密实现, 便于项目在不同的加密方式之间进行无侵入切换
$ pip install bk-crypto-python-sdk
更多用法参考:使用文档
非对称加密
from bkcrypto import constants
from bkcrypto.asymmetric import options
from bkcrypto.asymmetric.ciphers import BaseAsymmetricCipher
from bkcrypto.contrib.basic.ciphers import get_asymmetric_cipher
asymmetric_cipher: BaseAsymmetricCipher = get_asymmetric_cipher(
cipher_type=constants.AsymmetricCipherType.SM2.value,
# 传入 None 将随机生成密钥,业务可以根据场景选择传入密钥或随机生成
cipher_options={
constants.AsymmetricCipherType.SM2.value: options.SM2AsymmetricOptions(
private_key_string=None
),
constants.AsymmetricCipherType.RSA.value: options.SM2AsymmetricOptions(
private_key_string=None
),
}
)
# 加解密
assert "123" == asymmetric_cipher.decrypt(asymmetric_cipher.encrypt("123"))
# 验签
assert asymmetric_cipher.verify(plaintext="123", signature=asymmetric_cipher.sign("123"))
对称加密
import os
from bkcrypto import constants
from bkcrypto.symmetric.ciphers import BaseSymmetricCipher
from bkcrypto.contrib.basic.ciphers import get_symmetric_cipher
symmetric_cipher: BaseSymmetricCipher = get_symmetric_cipher(
cipher_type=constants.SymmetricCipherType.SM4.value,
common={"key": os.urandom(16)},
)
assert "123" == symmetric_cipher.decrypt(symmetric_cipher.encrypt("123"))
在 Django Settings 中配置加密算法类型
from bkcrypto import constants
BKCRYPTO = {
# 声明项目所使用的非对称加密算法
"ASYMMETRIC_CIPHER_TYPE": constants.AsymmetricCipherType.SM2.value,
# 声明项目所使用的对称加密算法
"SYMMETRIC_CIPHER_TYPE": constants.SymmetricCipherType.SM4.value,
}
非对称加密
from bkcrypto.asymmetric.ciphers import BaseAsymmetricCipher
from bkcrypto.contrib.django.ciphers import get_asymmetric_cipher
asymmetric_cipher: BaseAsymmetricCipher = get_asymmetric_cipher()
# 加解密
assert "123" == asymmetric_cipher.decrypt(asymmetric_cipher.encrypt("123"))
# 验签
assert asymmetric_cipher.verify(plaintext="123", signature=asymmetric_cipher.sign("123"))
对称加密
from bkcrypto.symmetric.ciphers import BaseSymmetricCipher
from bkcrypto.contrib.django.ciphers import get_symmetric_cipher
symmetric_cipher: BaseSymmetricCipher = get_symmetric_cipher()
assert "123" == symmetric_cipher.decrypt(symmetric_cipher.encrypt("123"))
在 Django Settings 中配置加密算法类型
from bkcrypto import constants
from bkcrypto.symmetric.options import AESSymmetricOptions, SM4SymmetricOptions
from bkcrypto.asymmetric.options import RSAAsymmetricOptions, SM2AsymmetricOptions
BKCRYPTO = {
# 声明项目所使用的非对称加密算法
"ASYMMETRIC_CIPHER_TYPE": constants.AsymmetricCipherType.SM2.value,
# 声明项目所使用的对称加密算法
"SYMMETRIC_CIPHER_TYPE": constants.SymmetricCipherType.SM4.value,
"SYMMETRIC_CIPHERS": {
# default - 所配置的对称加密实例,根据项目需要可以配置多个
"default": {
# 可选,用于在 settings 没法直接获取 key 的情况
# "get_key_config": "apps.utils.encrypt.key.get_key_config",
# 可选,用于 ModelField,加密时携带该前缀入库,解密时分析该前缀并选择相应的解密算法
# ⚠️ 前缀和 cipher type 必须一一对应,且不能有前缀匹配关系
# "db_prefix_map": {
# SymmetricCipherType.AES.value: "aes_str:::",
# SymmetricCipherType.SM4.value: "sm4_str:::"
# },
# 公共参数配置,不同 cipher 初始化时共用这部分参数
"common": {"key": "your key"},
"cipher_options": {
constants.SymmetricCipherType.AES.value: AESSymmetricOptions(key_size=16),
# 蓝鲸推荐配置
constants.SymmetricCipherType.SM4.value: SM4SymmetricOptions(mode=constants.SymmetricMode.CTR)
}
},
},
"ASYMMETRIC_CIPHERS": {
# 配置同 SYMMETRIC_CIPHERS
"default": {
"common": {"public_key_string": "your key"},
"cipher_options": {
constants.AsymmetricCipherType.RSA.value: RSAAsymmetricOptions(
padding=constants.RSACipherPadding.PKCS1_v1_5
),
constants.AsymmetricCipherType.SM2.value: SM2AsymmetricOptions()
},
},
}
}
非对称加密
使用 asymmetric_cipher_manager
获取 BKCRYPTO.ASYMMETRIC_CIPHERS
配置的 cipher
from bkcrypto.asymmetric.ciphers import BaseAsymmetricCipher
from bkcrypto.contrib.django.ciphers import asymmetric_cipher_manager
asymmetric_cipher: BaseAsymmetricCipher = asymmetric_cipher_manager.cipher(using="default")
# 加解密
assert "123" == asymmetric_cipher.decrypt(asymmetric_cipher.encrypt("123"))
# 验签
assert asymmetric_cipher.verify(plaintext="123", signature=asymmetric_cipher.sign("123"))
对称加密
使用 symmetric_cipher_manager
获取 BKCRYPTO.SYMMETRIC_CIPHERS
配置的 cipher
from bkcrypto.symmetric.ciphers import BaseSymmetricCipher
from bkcrypto.contrib.django.ciphers import symmetric_cipher_manager
# using - 指定对称加密实例,默认使用 `default`
symmetric_cipher: BaseSymmetricCipher = symmetric_cipher_manager.cipher(using="default")
assert "123" == symmetric_cipher.decrypt(symmetric_cipher.encrypt("123"))
Django ModelField
from django.db import models
from bkcrypto.contrib.django.fields import SymmetricTextField
class IdentityData(models.Model):
password = SymmetricTextField("密码", blank=True, null=True)
如果你有好的意见或建议,欢迎给我们提 Issues 或 Pull Requests,为蓝鲸开源社区贡献力量。
腾讯开源激励计划 鼓励开发者的参与和贡献,期待你的加入。
基于 MIT 协议, 详细请参考 LICENSE
FAQs
bk-crypto-python-sdk is a lightweight cryptography toolkit for Python applications based on Cryptodome / tongsuopy and other encryption libraries.
We found that bk-crypto-python-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.