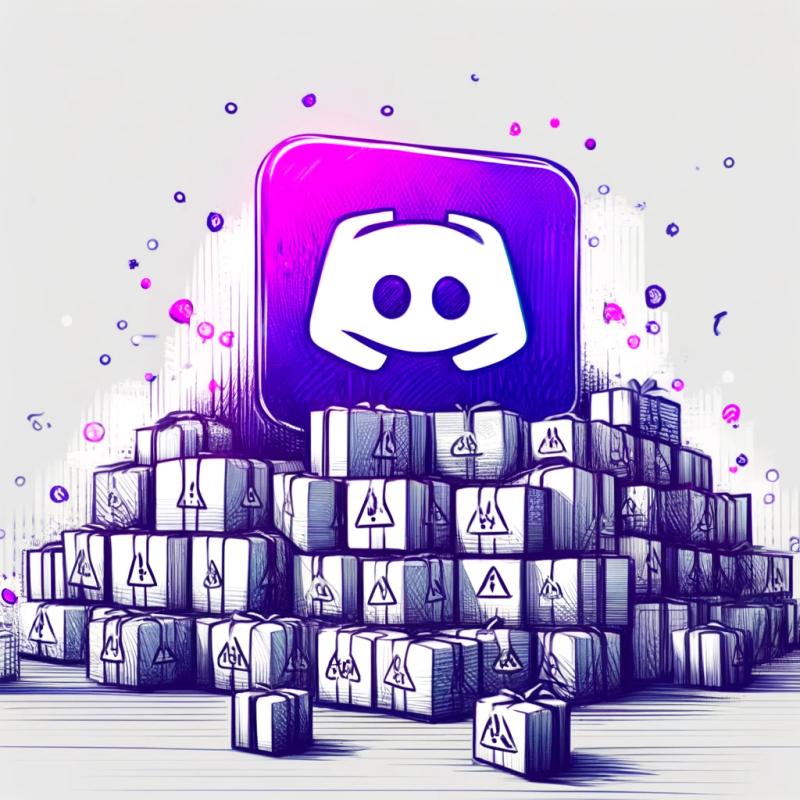
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Readme
Simple plugin to easily enable CORS support in Bottle routes.
from bottle import Bottle, request, run
from truckpad.bottle.cors import CorsPlugin, enable_cors
app = Bottle()
@app.get('/')
def index():
"""
CORS is disabled for this route
"""
return "cors is disabled here"
@enable_cors
@app.get('/endpoint_1')
def endpoint_1():
"""
CORS is enabled for this route.
OPTIONS requests will be handled by the plugin itself
"""
return "cors is enabled, OPTIONS handled by plugin"
@enable_cors
@app.route('/endpoint_2', method=['GET', 'POST', 'OPTIONS'])
def endpoint_2():
"""
CORS is enabled for this route.
OPTIONS requests will be handled by *you*
"""
if request.method == 'OPTIONS':
# do something here?
pass
return "cors is enabled, OPTIONS handled by you!"
app.install(CorsPlugin(origins=['http://list.of.allowed.domains.com', 'https://another.domain.org']))
run(app)
FAQs
CORS plugin for Bottle framework
We found that bottle-cors demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.