cv2_enumerate_cameras

Retrieve camera's name, VID, PID, and the corresponding OpenCV index.
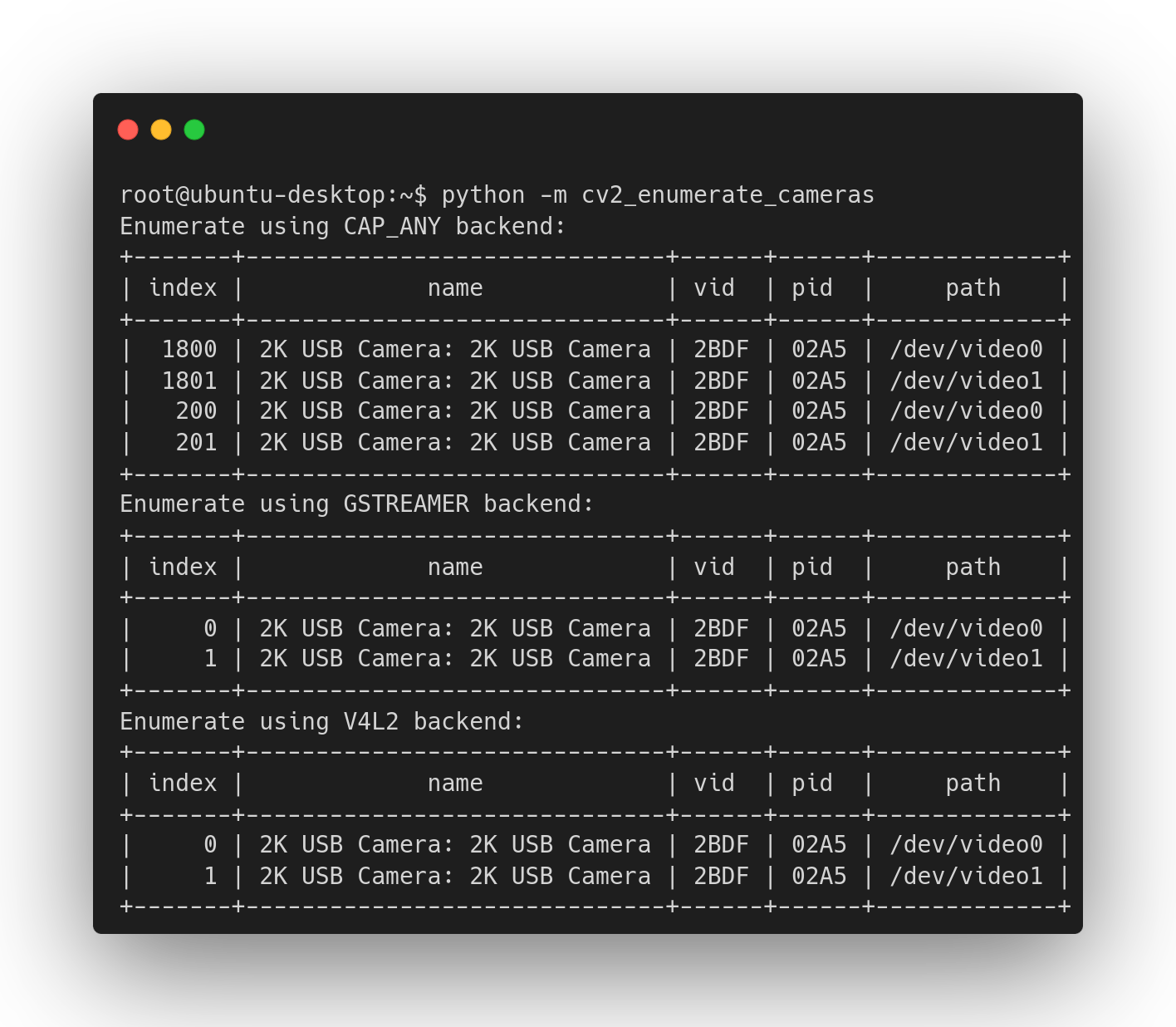
Installation
Install from PyPI
pip install cv2_enumerate_cameras
Install from Source
pip install git+https://github.com/chinaheyu/cv2_enumerate_cameras.git
Example
Run as Script
We have provided a simple script that prints out information for all cameras. Open a shell and simply run:
python -m cv2_enumerate_cameras
Supported Backends
Since OpenCV supports many different backends, not all of them provide support for camera enumeration. The following code demonstrates how to retrieve the names of the supported backends.
from cv2.videoio_registry import getBackendName
from cv2_enumerate_cameras import supported_backends
for backend in supported_backends:
print(getBackendName(backend))
Currently supported backends on windows:
- Microsoft Media Foundation (CAP_MSMF)
- DirectShow (CAP_DSHOW)
Currently supported backends on linux:
- GStreamer (CAP_GSTREAMER)
- V4L/V4L2 (CAP_V4L2)
Enumerate Cameras
This is an example showing how to enumerate cameras.
from cv2_enumerate_cameras import enumerate_cameras
for camera_info in enumerate_cameras():
print(f'{camera_info.index}: {camera_info.name}')
The enumerate_cameras(apiPreference: int = CAP_ANY)
function comes with the default parameter CAP_ANY
, and you will receive output similar to the following:
1400: HD Webcam
...
700: HD Webcam
701: OBS Virtual Camera
...
These indices may seem strange, since opencv defaults to using the high digits of index to represent the backend. For example, 701 indicates the second camera on the DSHOW backend (700).
You can also select a supported backend for enumerating camera devices.
Here's an example of enumerating camera devices via the CAP_MSMF
backend on windows.
import cv2
from cv2_enumerate_cameras import enumerate_cameras
for camera_info in enumerate_cameras(cv2.CAP_MSMF):
print(f'{camera_info.index}: {camera_info.name}')
Output:
0: HD Webcam
...
Once you have found the target camera, you can create a cv2.VideoCapture
by its index and backend properties.
cap = cv2.VideoCapture(camera_info.index, camera_info.backend)
When using CAP_ANY
as an option for the enumerate_cameras
function, the backend parameter can be omitted. However, it is highly recommended to pass it along when creating a VideoCapture
.
Camera Info
The cv2_enumerate_cameras.enumerate_cameras()
function will return a list of CameraInfo
objects, each containing information about a specific camera.
def enumerate_cameras(apiPreference: int = CAP_ANY) -> list[CameraInfo]:
...
CameraInfo.index
: Camera index for creating cv2.VideoCapture
ach containing all the information about a camera;CameraInfo.name
: Camera name;CameraInfo.path
: Camera device path;CameraInfo.vid
: Vendor identifier;CameraInfo.pid
: Product identifier;CameraInfo.backend
: Camera backend.
Find Camera by Vendor and Product Identifier
This example demonstrates how to automatically select a camera based on its VID and PID and create a VideoCapture
object.
import cv2
from cv2_enumerate_cameras import enumerate_cameras
def find_camera(vid, pid, apiPreference=cv2.CAP_ANY):
for i in enumerate_cameras(apiPreference):
if i.vid == vid and i.pid == pid:
return cv2.VideoCapture(i.index, i.backend)
return None
cap = find_camera(0x04F2, 0xB711)
while True:
result, frame = cap.read()
if not result:
break
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
TODO
Currently, support for MacOS is in the planning stage, and pull requests are very welcome.