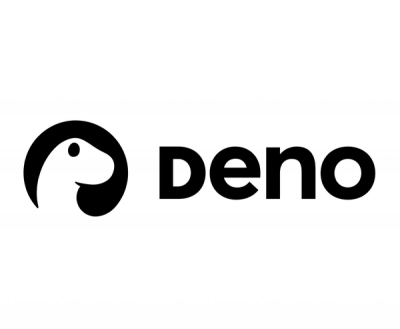
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Build dumb little "state machines" with Python decorators.
To install decorstate from pip:
$ pip install decorstate
To install decorstate from source:
$ git clone git@github.com:ahawker/decorstate.git
$ cd decorstate
$ python setup.py install
How do I use this pile?
import decorstate
class Switch(object):
state = 'off'
@decorstate.transition('off', 'on')
def on(self, *args, **kwargs):
print 'You turned me on!'
@decorstate.transition('on', 'off')
def off(self, *args, **kwargs):
print 'You turned me off!'
>>> switch = Switch()
>>> switch.state
'off'
>>> switch.on()
You turned me on!
'on'
>>> switch.off()
You turned me off!
'off'
A switch? Really? How lame.
import decorstate
class BrokenSwitch(object):
state = 'off'
@decorstate.transition('off', 'on')
def on(self, *args, **kwargs):
print 'You turned me on!'
@decorstate.transition('on', 'off')
def off(self, *args, **kwargs):
print 'You turned me off? Nah!'
@off.guard
def off(self, *args, **kwargs):
print 'Ha! I laugh at your feeble attempt!'
>>> broken_switch = BrokenSwitch()
>>> broken_switch.state
'off'
>>> broken_switch.on()
You turned me on!
'on'
>>> broken_switch.off()
Ha! I laugh at your feeble attempt!
'on'
>>> broken_switch.state
'on'
>>> broken_switch.off()
Ha! I laugh at your feeble attempt!
'on'
>>> broken_switch.state
'on'
A broken switch? Yawn.
import decorstate
class InstantOffSwitch(object):
state = 'off'
@decorstate.transition('off', 'off')
def on(self, *args, **kwargs):
print 'You turned me on!'
@decorstate.transition('on', 'off')
def off(self, *args, **kwargs):
print 'You turned me off!'
@on.after
def on(self, *args, **kwargs):
print 'Ha! No light for you!'
>>> instant_off_switch = InstantOffSwitch()
>>> instant_off_switch.state
'off'
>>> instant_off_switch.on()
You turned me on!
Ha! No light for you!
'off'
>>> instant_off_switch.state
'off'
Well, that's kinda mean.
import decorstate
import random
class IoTSwitch(object):
state = 'off'
@decorstate.transition('off', 'off')
def on(self, *args, **kwargs):
print 'You turned me on? Maybe...'
@decorstate.transition('on', 'off')
def off(self, *args, **kwargs):
print 'You turned me off? Maybe...'
@on.guard
def on(self, *args, **kwargs):
return self.coin_flip()
@off.guard
def off(self, *args, **kwargs):
return not self.coin_flip()
@staticmethod
def coin_flip():
return random.randint(1, 2) == 1
>>> iot_switch = IoTSwitch()
>>> iot_switch.state
'off'
>>> iot_switch.on()
'off'
>>> iot_switch.on()
'off'
>>> iot_switch.on()
'off'
>>> iot_switch.on()
You turned me on? Maybe...
'on'
>>> iot_switch.off()
'on'
>>> iot_switch.off()
'on'
>>> iot_switch.off()
'on'
>>> iot_switch.off()
'on'
>>> iot_switch.off()
You turned me off? Maybe...
'off'
Hey now, why you hating? Internet powered light switches are next level shit. My living room has its own twitter feed.
I was interesting in doing something a bit more complex using the Python descriptor protocol.
Random thoughts and musing about potential changes/features.
FAQs
Simple "state machines" with Python decorators
We found that decorstate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.