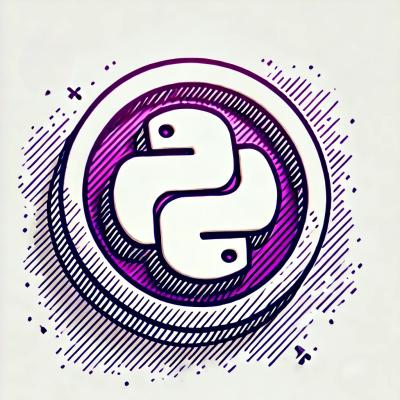
Security News
Python Adopts Standard Lock File Format for Reproducible Installs
Python has adopted a standardized lock file format to improve reproducibility, security, and tool interoperability across the packaging ecosystem.
discord-embedbuilder
Advanced tools
Discord library built on discord.py to simplify source code by rendering templates of embeds and menus
Discord templating engine built on discord.py, to help separate text of embeds from the source code. Inspired by Flask.
Key Features:
Python3.8 or higher is required
To run the tests, run the following command in the root directory:
Windows:
python -m unittest tests -v
Linux:
python3 -m unittest tests -v
This is explained in more detail in the wiki
Wrap expressions that need to evaluated with {}
, such as {player.name}
or {todays_date}
Sample XML file:
<discord>
<message key="test_key">
<embed>
<title>Test</title>
<description>Test Description</description>
<colour>magenta</colour>
<timestamp format="%Y-%m-%d %H:%M:%S.%f">{todays_date}</timestamp>
<url>https://www.discord.com</url>
<fields>
<field>
<name>Test Field</name>
<value>Test Text</value>
</field>
</fields>
<footer>
<text>Test Footer</text>
<icon>https://cdn.discordapp.com/embed/avatars/0.png</icon>
</footer>
<thumbnail>https://cdn.discordapp.com/embed/avatars/0.png</thumbnail>
<image>https://cdn.discordapp.com/embed/avatars/0.png</image>
<author>
<name>Test Author</name>
<icon>https://cdn.discordapp.com/embed/avatars/0.png</icon>
<url>https://discordapp.com</url>
</author>
</embed>
<view>
<components>
<button key="understood_button">
<label>Understood</label>
<style>success</style>
</button>
</components>
</view>
</message>
</discord>
using the above xml file, for example, you can create an embed with the following code:
import datetime
from typing import Literal
import discord
from discord.ext import commands
import embedbuilder
from embedbuilder.template_engines import formatter
bot = commands.AutoShardedBot(command_prefix="!", intents=discord.Intents.all())
Messages = Literal["test_key"]
async def acknowledged(interaction: discord.Interaction):
await interaction.response.send_message("Acknowledged", ephemeral=True)
@bot.command()
@embedbuilder.embedbuilder_context(formatter.Formatter(), "templates/test.xml")
async def test(ctx: embedbuilder.embedbuilderContext[Messages]):
callables = {"understood_button": acknowledged}
await ctx.rendered_send("test_key", callables, keywords={
"todays_date": datetime.datetime.now()
})
FAQs
Discord library built on discord.py to simplify source code by rendering templates of embeds and menus
We found that discord-embedbuilder demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Python has adopted a standardized lock file format to improve reproducibility, security, and tool interoperability across the packaging ecosystem.
Security News
OpenGrep has restored fingerprint and metavariable support in JSON and SARIF outputs, making static analysis more effective for CI/CD security automation.
Security News
Security experts warn that recent classification changes obscure the true scope of the NVD backlog as CVE volume hits all-time highs.