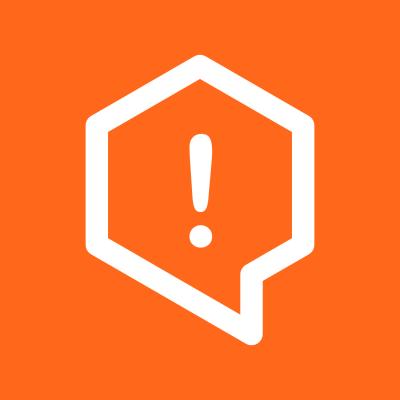
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
flask-structured-api
Advanced tools
A structured Flask API boilerplate with built-in AI capabilities, featuring SQLModel ORM and Pydantic validation
A production-ready Flask API framework with built-in storage, authentication, and AI capabilities. Designed for developers who need a robust foundation for building scalable APIs while following best practices.
# Install the core package
pip install flask-structured-api
# Create new project
mkdir my-api && cd my-api
# Initialize basic structure
flask-api init
# Install dependencies
pip install -r requirements.txt
Note: When using the PyPI package, you'll need to set up your own PostgreSQL and Redis services.
For a fresh start with full Docker support:
# Create and enter project directory
mkdir my-api && cd my-api
# Initialize empty git repo
git init
# Add boilerplate as remote
git remote add boilerplate https://github.com/julianfleck/flask-structured-api.git
# Pull files without history
git pull boilerplate main --allow-unrelated-histories
# Make initial commit
git add .
git commit -m "Initial commit from boilerplate"
# Start services
docker-compose up -d
If you want to preserve boilerplate history (not recommended for production):
# Clone the repo
git clone https://github.com/julianfleck/flask-structured-api.git my-api
cd my-api
# Start services
docker-compose up -d
Essential environment variables:
# Required
FLASK_APP=flask_structured_api.main:app
DATABASE_URL=postgresql://user:password@localhost:5432/dbname
REDIS_URL=redis://localhost:6379/0
SECRET_KEY=your-secret-key
# Optional
AI_PROVIDER=openai
AI_API_KEY=your-api-key
from flask import Blueprint
from flask_structured_api.core.auth import require_auth
from flask_structured_api.models.responses import SuccessResponse
bp = Blueprint('example', __name__)
@bp.route('/hello', methods=['GET'])
@require_auth
def hello_world():
return SuccessResponse(
message="Hello, World!",
data={"authenticated": True}
).dict()
from flask_structured_api.core.storage import store_api_data
@bp.route('/ai/generate', methods=['POST'])
@require_auth
@store_api_data() # Automatically stores request/response
def generate():
result = ai_service.generate(request.json)
return SuccessResponse(data=result).dict()
See CONTRIBUTING.md for guidelines.
This project is licensed under the Apache License 2.0 - see LICENSE and NOTICE for details.
When using this project, please include the following attribution in your documentation:
Based on Flask Structured API (https://github.com/julianfleck/flask-structured-api)
Created by Julian Fleck and contributors
FAQs
A structured Flask API boilerplate with built-in AI capabilities, featuring SQLModel ORM and Pydantic validation
We found that flask-structured-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.