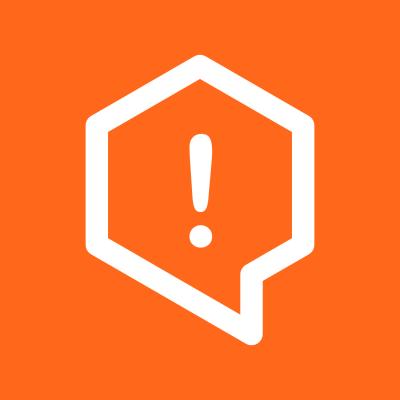
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Flask Endpoints for User Management and Authentication Middleware
from frappyflaskauth import register_endpoints
from flask import Flask
app = Flask(__name__)
# create store instances for users
user_store = ...
# this is a minimal configuration
register_endpoints(app, user_store)
Parameters
app
- the Flask app instanceuser_store
- an store class providing user related methodstoken_store
- optional - if you want login sessions to survive a server restartoptions_override
- default {}
- a dictionary containing configuration options that override the defaults:Options
api_prefix
- default /api/user
- the API prefix used for all endpoints (e.g. /api/user/login
)token_expiration
- default 86400
- the number of seconds a login session is valid for before it expiresdefault_permissions
- default []
- the initial permissions any user receives on creation (local users)user_admin_permission
- default admin
- the permission a user requires to be able to invoke user management
endpoints like update permissions, delete users, fetch all users, update passwords of other users.no_user_management
- default False
- if you don't want any user management endpoints to be registeredapi_keys
- default False
- if you need API keys to access endpoints (integrated into check_login_state
). API
keys are provided in the Authorization
header prefixed with Token $KEY
(where $KEY
is the user's API key)allow_own_profile_edit
- default False
- if this is set to true, any user can update their own profile info
(user.profile
).page_size
- default 25
- the number of users returned with the /users
endpoint (lists all users)To check if a user is authenticated and get the currently logged in user in your own endpoints, simply use the
check_login_state
function. It will
from frappyflaskauth import check_login_state
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("/api/my-endpoint", methods=["GET"])
def my_custom_endpoint():
user = check_login_state("view")
# execution will only go past this point, if user is logged in AND has "view" permission
print(user.id, user.permissions) # this is the currently logged in user
return jsonify({})
@app.route("/api/my-endpoint", methods=["GET"])
def my_logged_in_endpoint():
_ = check_login_state() # simply check if the user is logged in, ignore the returned user
return jsonify({})
@app.route("/api/my-endpoint", methods=["GET"])
def my_api_key_enabled_endpoint():
_ = check_login_state(allow_api_key=True)
Parameters:
permission
, default None
which is a string that is checked against the user.permissions
field (which is a list
)allow_api_key
, default False
which is a flag enabling API keys to access the endpoint protected by this function
call.FAQs
Flask endpoints for user management and authentication.
We found that frappyflaskauth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.