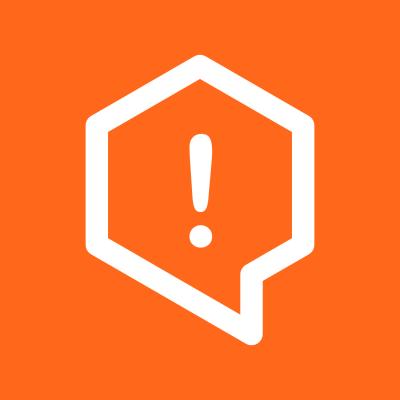
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
The LMSystems SDK provides flexible interfaces for integrating and executing purchased graphs from the LMSystems marketplace in your Python applications. The SDK offers two main approaches:
Get started quickly with our interactive Colab notebook:
This notebook provides a hands-on introduction to the LMSystems SDK with ready-to-run examples.
Install the package using pip:
pip install lmsystems
The client SDK provides direct interaction with LMSystems graphs:
from lmsystems.client import LmsystemsClient
import os
from dotenv import load_dotenv
# Load environment variables
load_dotenv()
# Async usage
async def main():
# Simple initialization with just graph name and API key
client = await LmsystemsClient.create(
graph_name="graph-name-id",
api_key=os.environ["LMSYSTEMS_API_KEY"]
)
# Create thread and run with error handling
try:
thread = await client.create_thread()
# No need to provide config - it's handled through your account settings
run = await client.create_run(
thread,
input={"messages": [{"role": "user", "content": "What's this repo about?"}],
"repo_url": "",
"repo_path": "",
"github_token": ""}
)
# Stream response
async for chunk in client.stream_run(thread, run):
print(chunk)
except Exception as e:
print(f"Error: {str(e)}")
if __name__ == "__main__":
import asyncio
asyncio.run(main())
For integration with LangGraph workflows:
from lmsystems.purchased_graph import PurchasedGraph
from langgraph.graph import StateGraph, START, MessagesState
import os
from dotenv import load_dotenv
# Load environment variables
load_dotenv()
# Set required state values
state_values = {
"repo_url": "https://github.com/yourusername/yourrepo",
"github_token": "your-github-token",
"repo_path": "/path/to/1234322"
}
# Initialize the purchased graph - no config needed!
purchased_graph = PurchasedGraph(
graph_name="github-agent-6",
api_key=os.environ.get("LMSYSTEMS_API_KEY"),
default_state_values=state_values
)
# Create a parent graph with MessagesState schema
builder = StateGraph(MessagesState)
builder.add_node("purchased_node", purchased_graph)
builder.add_edge(START, "purchased_node")
graph = builder.compile()
# Invoke the graph
result = graph.invoke({
"messages": [{"role": "user", "content": "what's this repo about?"}]
})
# Stream outputs (optional)
for chunk in graph.stream({
"messages": [{"role": "user", "content": "what's this repo about?"}]
}, subgraphs=True):
print(chunk)
The SDK now automatically handles configuration through your LMSystems account. To set up:
Your configured API keys and settings will be automatically used when running graphs - no need to include them in your code!
Note: While configuration is handled automatically, you can still override settings programmatically if needed:
# Optional: Override stored config
config = {
"configurable": {
"model": "gpt-4",
"openai_api_key": "your-custom-key"
}
}
purchased_graph = PurchasedGraph(
graph_name="github-agent-6",
api_key=os.environ.get("LMSYSTEMS_API_KEY"),
config=config # Optional override
)
Store your LMSystems API key securely using environment variables:
export LMSYSTEMS_API_KEY="your-api-key"
LmsystemsClient.create(
graph_name: str,
api_key: str
)
Parameters:
graph_name
: Name of the graph to interact withapi_key
: Your LMSystems API keyMethods:
create_thread()
: Create a new thread for graph executioncreate_run(thread, input)
: Create a new run within a threadstream_run(thread, run)
: Stream the output of a runget_run(thread, run)
: Get the status and result of a runlist_runs(thread)
: List all runs in a threadPurchasedGraph(
graph_name: str,
api_key: str,
config: Optional[RunnableConfig] = None,
default_state_values: Optional[dict[str, Any]] = None
)
Parameters:
graph_name
: Name of the purchased graphapi_key
: Your LMSystems API keyconfig
: Optional configuration for the graphdefault_state_values
: Default values for required state parametersMethods:
invoke()
: Execute the graph synchronouslyainvoke()
: Execute the graph asynchronouslystream()
: Stream graph outputs synchronouslyastream()
: Stream graph outputs asynchronouslyThe SDK provides specific exceptions for different error cases:
AuthenticationError
: API key or authentication issuesGraphError
: Graph execution or configuration issuesInputError
: Invalid input parametersAPIError
: Backend communication issuesExample error handling:
from lmsystems.exceptions import (
LmsystemsError,
AuthenticationError,
GraphError,
InputError,
APIError,
GraphNotFoundError,
GraphNotPurchasedError
)
try:
result = graph.invoke(input_data)
except AuthenticationError as e:
print(f"Authentication failed: {e}")
except GraphNotFoundError as e:
print(f"Graph not found: {e}")
except GraphNotPurchasedError as e:
print(f"Graph not purchased: {e}")
except GraphError as e:
print(f"Graph execution failed: {e}")
except InputError as e:
print(f"Invalid input: {e}")
except APIError as e:
print(f"API communication error: {e}")
except LmsystemsError as e:
print(f"General error: {e}")
The SDK supports different streaming modes through the StreamMode
enum:
from lmsystems import StreamMode
# Stream run with specific modes
async for chunk in client.stream_run(
thread=thread,
input=input_data,
stream_mode=[
StreamMode.MESSAGES, # Stream message updates
StreamMode.VALUES, # Stream value updates from nodes
StreamMode.UPDATES, # Stream general state updates
StreamMode.CUSTOM # Stream custom-defined updates
]
):
print(chunk)
Available stream modes:
StreamMode.MESSAGES
: Stream message updates from the graphStreamMode.VALUES
: Stream value updates from graph nodesStreamMode.UPDATES
: Stream general state updatesStreamMode.CUSTOM
: Stream custom-defined updatesFor support, feature requests, or bug reports:
FAQs
SDK for integrating purchased graphs from the lmsystems marketplace.
We found that lmsystems demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.