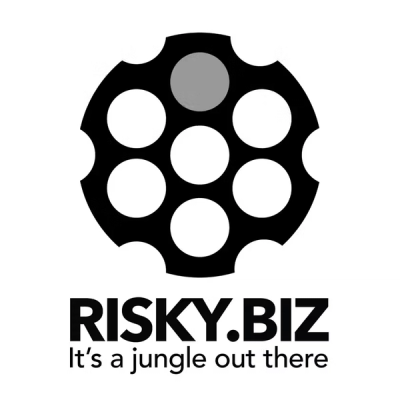
Security News
Risky Business Podcast: Why Open Source Software Needs Better Malware Tracking
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.