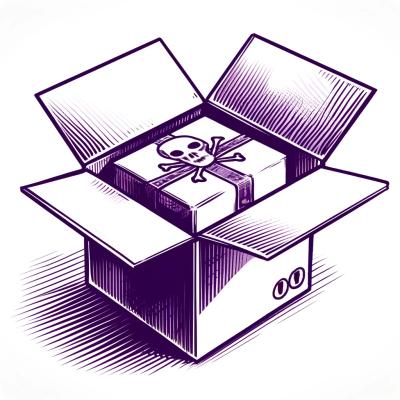
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
The Python Novu SDK and package provides a fluent and expressive interface for interacting with Novu's API and managing notifications.
To install this package
# Via pip
pip install novu
# Via poetry
poetry add novu
This package is a wrapper of all the resources offered by Novu, we will just start by triggering an event on Novu.
To do this, you will need to:
from novu.api import EventApi
event_api = EventApi("https://api.novu.co", "<NOVU_API_KEY>")
event_api.trigger(
name="<YOUR_WORKFLOW_ID>", # The workflow ID is the slug of the workflow name. It can be found on the workflow page.
recipients="<YOUR_SUBSCRIBER_ID>",
payload={}, # Your Novu payload goes here
)
This will trigger a notification to the subscribers.
Firstly, make imports and declare the needed variables this way:
from novu.api import EventApi
url = "https://api.novu.co"
api_key = "<NOVU_API_KEY>"
# You can sign up on https://web.novu.co to get your API key from https://web.novu.co/settings
Trigger an event - Send notification to subscribers:
from novu.api import EventApi
novu = EventApi(url, api_key).trigger(
name="digest-workflow-example", # This is the Workflow ID. It can be found on the workflow page.
recipients="<SUBSCRIBER_IDENTIFIER>", # The subscriber ID can be gotten from the dashboard.
payload={}, # Your custom Novu payload goes here
)
Bulk Trigger events - Trigger multiple events at once:
from novu.dto.event import InputEventDto
from novu.api import EventApi
url = "https://api.novu.co"
api_key = "<NOVU_API_KEY>"
event_1 = InputEventDto(
name="digest-workflow-example", # The workflow ID is the slug of the workflow name. It can be found on the workflow page.
recipients="<SUBSCRIBER_IDENTIFIER>",
payload={}, # Your custom Novu payload goes here
)
event_2 = InputEventDto(
name="digest-workflow-example",
recipients="<SUBSCRIBER_IDENTIFIER>",
payload={},
)
novu = EventApi("https://api.novu.co", api_key).trigger_bulk(events=[event1, event2])
Include actor field:
from novu.api import EventApi
novu = EventApi(url, api_key).trigger(
name="workflow_trigger_identifier",
recipients="subscriber_id",
actor={
"subscriberId": "subscriber_id_actor"
},
payload={
"key":"value"
},
)
Broadcast to all current subscribers:
novu = EventApi(url, api_key).broadcast(
name="digest-workflow-example",
payload={"customVariable": "value"}, # Optional
)
from novu.dto.subscriber import SubscriberDto
from novu.api.subscriber import SubscriberApi
url = "https://api.novu.co"
api_key = "<NOVU_API_KEY>"
# Define a subscriber instance
subscriber = SubscriberDto(
email="novu_user@mail.com",
subscriber_id="82a48af6ac82b3cc2157b57f", #This is what the subscriber_id looks like
first_name="", # Optional
last_name="", # Optional
phone="", # Optional
avatar="", # Optional
)
# Create a subscriber
novu = SubscriberApi(url, api_key).create(subscriber)
# Get a subscriber
novu = SubscriberApi(url, api_key).get(subscriber_id)
# Get list of subscribers
novu = SubscriberApi(url, api_key).list()
from novu.api import TopicApi
url = "<NOVU_URL>"
api_key = "<NOVU_API_KEY>"
# Create a topic
novu = TopicApi(url, api_key).create(
key="new-customers", name="New business customers"
)
# Get a topic
novu = TopicApi(url, api_key).get(key="new-customers")
# List topics
novu = TopicApi(url, api_key).list()
# Rename a topic
novu = TopicApi(url, api_key).rename(key="new-customers", name="New business customers")
# Subscribe a list of subscribers to a topic
novu = TopicApi(url, api_key).subscribe(key="old-customers", subscribers="<LIST_OF_SUBSCRIBER_IDs>")
# Unsubscribe a list of subscribers from a topic
novu = TopicApi(url, api_key).unsubscribe(key="old-customers", subscribers="<LIST_OF_SUBSCRIBER_IDs>")
from novu.api.feed import FeedApi
url = "<NOVU_URL>"
api_key = "<NOVU_API_KEY>"
# Create a Feed
novu = FeedApi(url, api_key).create(name="<SUPPLY_NAME_FOR_FEED>")
# Delete a Feed
FeedApi(url, api_key).delete(feed_id="<FEED_NOVU_INTERNAL_ID>")
# List feeds
novu = FeedApi(url, api_key).list()
from novu.api.environment import EnvironmentApi
url = "<NOVU_URL>"
api_key = "<NOVU_API_KEY>"
# Create an Environment
novu = EnvironmentApi(url, api_key).create(
name="<INSERT_NAME>",
parent_id="<INSERT_PARENT_ID>" # Optional. Defaults to None
)
# # List existing environments
novu = EnvironmentApi(url, api_key).list()
# # Get the current environment
novu = EnvironmentApi(url, api_key).current()
# # Retrieve an environment's API_KEY
novu = EnvironmentApi(url, api_key).api_keys()
from novu.api.tenant import TenantApi
url = "<NOVU_URL>"
api_key = "<NOVU_API_KEY>"
# Create an Environment
tenant = TenantApi(url, api_key).create(
identifier="<INSERT_UNIQUE_TENANT_ID>",
name="<INSERT_NAME>",
data={} # Optional. Defaults to {}
)
# List existing tenants
tenants = TenantApi(url, api_key).list()
tenants = TenantApi(url, api_key).list(page=1, limit=10)
# Get a tenant
tenant = TenantApi(url, api_key).get("<TENANT-IDENTIFIER>")
# Patch some field of a tenant
tenant = TenantApi(url, api_key).patch(
"<CURRENT-TENANT-IDENTIFIER>",
identifier="<NEW-IDENTIFIER>",
name="<NEW-NAME>",
data="<NEW-DATA>"
)
# Delete a tenant
TenantApi(url, api_key).delete("<TENANT-IDENTIFIER>")
After a quick start with the SDK, you'll quickly get to grips with the advanced use of the SDK and the other APIs available.
For this purpose, documentation is available here: https://novu-python.readthedocs.io/
# install deps
poetry install
# pre-commit
poetry run pre-commit install --install-hook
poetry run pre-commit install --install-hooks --hook-type commit-msg
Feature requests, bug reports and pull requests are welcome. Please create an issue.
Be sure to visit the Novu official documentation website for additional information about our SDK. If you need additional assistance, join our Discord server here.
Novu Python SDK is licensed under the MIT License - see the LICENSE file for details.
FAQs
This project aims to provide a wrapper for the Novu API.
We found that novu demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.