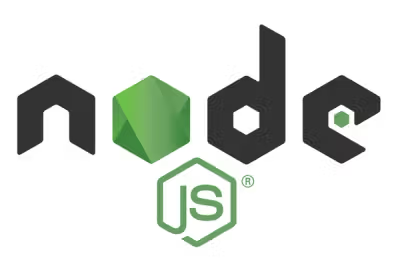
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
A class used for querying parts in Octopart using Nexar API v4.0.
The following class has all the needed methods required by NSG to create a query to Octopart.
This package is maintained and used by NSG, please refer to the licensing before using.
Use the package manager pip to install nsg-parts-search.
pip install nsg-parts-search
Use the application client ID and secret. Refer to the NSG Documentation.
Set environment variables CLIENT_ID and CLIENT_SECRET.
General example to get a response from a list of parts search:
from nsgsearch import *
# set credentials
clientId = os.environ['CLIENT_ID']
clientSecret = os.environ['CLIENT_SECRET']
# start the client
search = NSG_OctopartSearch()
search.setCredentials(clientId, clientSecret)
search.startClient()
# search for parts
parts_list = []
results = search.partsSearch(parts_list)
print(results)
Say that from a list of parts you want a table of the Manufacturer, Suppliers, Quantity and Price per unit in USD:
# loop through all parts
for part in parts_list:
# enter just if the part number was found
if results[part]['found']:
part_data = results[part]['data']
# get a table of prices for the part
priceTable = search.partPricesTable(part_data)
Maybe now you want to get the lowest price from the table for that part number:
def get_lowest_price(table):
price = table[0]['price']
supplier = str()
qty = int()
for row in table:
if row['price'] < price:
price = row['price']
supplier = row['supplier']
qty = row['reqPurchase']
return ([supplier, qty, price])
Now let's say you want to get the lead times for all suppliers and filter your prices to be no more than 30 days old:
The response from the search is a dictionary containing all the requested part numbers as keys.
Each key contains another dictionary with the keys found and data and have the following format:
{
"PN#": {
"found" : True / False,
"data" : {...}
},
{...}
}
The default query response has the following format:
part {
mpn
manufacturer {
name
}
shortDescription
specs {
attribute {
name
group
}
displayValue
}
octopartUrl
similarParts {
mpn
shortDescription
manufacturer {
name
}
octopartUrl
category {
name
}
}
companionProducts {
part {
mpn
shortDescription
manufacturer {
name
}
octopartUrl
category {
name
}
}
}
category {
name
}
bestDatasheet {
url
}
counts
medianPrice1000 {
price
}
sellers {
company {
name
}
country
offers {
sku
inventoryLevel
moq
prices {
quantity
price
currency
}
clickUrl
updated
factoryLeadDays
}
isRfq
}
}
You can change the query response by using the method changeQuery():
newResponse =
'''
query Search($mpn: String!) {
supSearchMpn(q: $mpn, limit: 1) {
results {
part {
mpn
shortDescription
manufacturer {
name
}
}
}
}
}
'''
search.changeQuery(newResponse)
https://octopart.com/api/v4/reference#part
FAQs
NSG class for making parts searches in Octopart using the Nexar API v4
We found that nsg-parts-search demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.