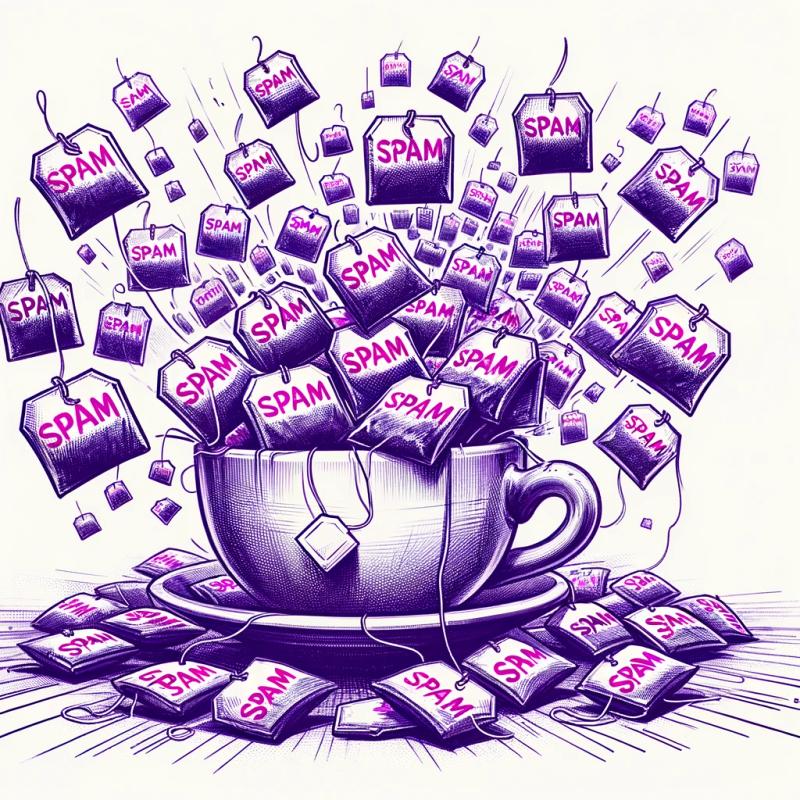
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
python3-anticaptcha
Readme
Python 3.6+ library for Anti-Captcha service.
Tested on UNIX based OS. The library is intended for software developers and is used to work with the AntiCaptcha service API.
pip install python3-anticaptcha
git clone https://github.com/AndreiDrang/python3-anticaptcha.git
cd python3-anticaptcha
python setup.py install
По всем вопросам можете писать в Telegram чат.
With any questions, please contact us in Telegram.
Присутствуют примеры работы с библиотекой.
Full examples you can find here.
from python3_anticaptcha import CustomResultHandler
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = "your_key"
# Task ID to get result
TASK_ID = 123456
# This module is used to obtain the result of solving the task in "manual" mode
custom_result = CustomResultHandler.CustomResultHandler(
anticaptcha_key=ANTICAPTCHA_KEY
)
user_answer = custom_result.task_handler(task_id=TASK_ID)
print(user_answer)
from python3_anticaptcha import ImageToTextTask
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# Link to captcha image.
image_link = "https://pythoncaptcha.tech/static/image/common_image_example/800070.png"
# Get string for solve captcha, and some other info.
user_answer = ImageToTextTask.ImageToTextTask(anticaptcha_key = ANTICAPTCHA_KEY).\
captcha_handler(captcha_link=image_link)
print(user_answer)
from python3_anticaptcha import NoCaptchaTaskProxyless
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# G-ReCaptcha ключ сайта. Website google key.
SITE_KEY = '6LeuMjIUAAAAAODtAglF13UiJys0y05EjZugej6b'
# Page url.
PAGE_URL = 'https://www.google.com/recaptcha/intro/android.html'
# Get string for solve captcha, and other info.
user_answer = NoCaptchaTaskProxyless.NoCaptchaTaskProxyless(anticaptcha_key = ANTICAPTCHA_KEY)\
.captcha_handler(websiteURL=PAGE_URL,
websiteKey=SITE_KEY)
print(user_answer)
from python3_anticaptcha import ReCaptchaV3TaskProxyless
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# G-ReCaptcha - website google key.
SITE_KEY = '6LeuMjIUAAAAAODtAglF13UiJys0y05EjZugej6b'
# Page url.
PAGE_URL = 'https://some_link'
# The filter by which the employee with the required minimum score is selected.
# possible options - 0.3, 0.5, 0.7
MIN_SCORE=0.3
# The value of the `action` parameter, which is passed by the recaptcha widget to google.
PAGE_ACTION='login'
# Get string for solve captcha, and other info.
user_answer = ReCaptchaV3TaskProxyless.ReCaptchaV3TaskProxyless(anticaptcha_key = ANTICAPTCHA_KEY)\
.captcha_handler(websiteURL=PAGE_URL,
websiteKey=SITE_KEY,
minScore=MIN_SCORE,
pageAction=PAGE_ACTION
)
print(user_answer)
from python3_anticaptcha import FunCaptchaTask
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# G-ReCaptcha site key
SITE_KEY = ''
# Link to the page with captcha
PAGE_URL = ''
# Get full data for solve captcha.
user_answer = FunCaptchaTask.FunCaptchaTask(anticaptcha_key=ANTICAPTCHA_KEY,
proxyType="http",
proxyAddress="8.8.8.8",
proxyPort=8080)\
.captcha_handler(websiteURL=PAGE_URL,
websitePublicKey=SITE_KEY)
print(user_answer)
from python3_anticaptcha import AntiCaptchaControl
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# Balance info
result = AntiCaptchaControl.AntiCaptchaControl(anticaptcha_key = ANTICAPTCHA_KEY).get_balance()
# Submitting a complaint about incorrectly resolved captcha images
result = AntiCaptchaControl.AntiCaptchaControl(anticaptcha_key = ANTICAPTCHA_KEY).complaint_on_result(
reported_id=543212, captcha_type="image"
)
# Submitting a complaint about incorrectly resolved ReCaptcha
result = AntiCaptchaControl.AntiCaptchaControl(anticaptcha_key = ANTICAPTCHA_KEY).complaint_on_result(
reported_id=5432134, captcha_type="recaptcha"
)
# Giving information about loading the queue, depending on the queue ID
result = AntiCaptchaControl.AntiCaptchaControl(anticaptcha_key = ANTICAPTCHA_KEY).get_queue_status(queue_id=1)
from python3_anticaptcha import CustomCaptchaTask
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# ссылка на изображение
imageUrl = "https://files.anti-captcha.com/26/41f/c23/7c50ff19.jpg"
# минимальный пример использования модуля
my_custom_task = CustomCaptchaTask.CustomCaptchaTask(anticaptcha_key=ANTICAPTCHA_KEY).\
captcha_handler(imageUrl=imageUrl)
print(my_custom_task)
from python3_anticaptcha import GeeTestTaskProxyless
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
# обязательные параметры
websiteURL = "http:\/\/mywebsite.com\/geetest\/test.php"
gt = "874703612e5cac182812a00e273aad0d"
challenge = "a559b82bca2c500101a1c8a4f4204742"
# пример работы с GeeTestTask без прокси
result = GeeTestTaskProxyless.GeeTestTaskProxyless(anticaptcha_key=ANTICAPTCHA_KEY,
websiteURL=websiteURL,
gt=gt).\
captcha_handler(challenge=challenge)
print(result)
from python3_anticaptcha import HCaptchaTaskProxyless
# Enter the key to the AntiCaptcha service from your account. Anticaptcha service key.
ANTICAPTCHA_KEY = ""
WEB_URL = "https://dashboard.hcaptcha.com/signup"
SITE_KEY = "00000000-0000-0000-0000-000000000000"
result = HCaptchaTaskProxyless.HCaptchaTaskProxyless(anticaptcha_key=ANTICAPTCHA_KEY).\
captcha_handler(websiteURL=WEB_URL, websiteKey=SITE_KEY)
print(result)
Кроме того, для тестирования различных типов капчи предоставляется специальный сайт, на котором собраны все имеющиеся типы капчи, с удобной системой тестирования ваших скриптов.
Some examples you can test with our web-site.
export anticaptcha_key=SERVICE_KEY
make test
FAQs
Python 3 Anti-Captcha service library with AIO module.
We found that python3-anticaptcha demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.