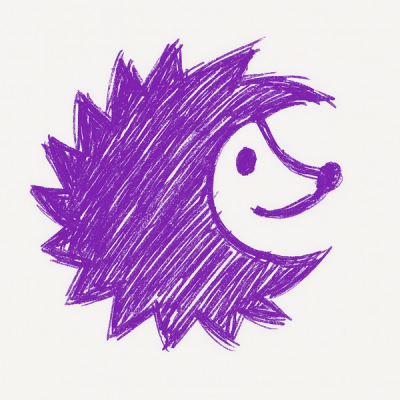
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
QuickSave is a fast, memory-efficient, and lightweight key-value database designed for use in small applications. It operates as a pure Python solution, offering a dictionary-like interface for easy integration. QuickSave works efficiently without dependencies, but if you want to boost its performance even further, you can install msgspec
, which significantly enhances its speed.
QuickSave stands out for its speed, memory efficiency, and simplicity. Here are some of the key reasons you should consider using it:
msgspec
: By installing the optional msgspec
library, you can further enhance QuickSave's performance, especially when handling complex data types.Install QuickSave using pip:
pip install --upgrade qsave
Optionally, install msgspec
to boost performance:
pip install msgspec==0.19.0
If you want use async version of QuickSave:
pip install qsave[async]
To start using QuickSave, import it and initialize your database:
from qsave import QuickSave
db = QuickSave(path="path/to/your/file.json", pretty=True)
The pretty argument beautifies the saved data for better readability (optional).
By default, changes are automatically saved when the with
block ends:
with db.session() as session:
session["key"] = "value"
print(session.get("key")) # Output: None, not yet saved
# Exiting the block automatically commits changes
with db.session() as session:
print(session.get("key")) # Output: value
For full control over when changes are saved, use commit_on_expire=False:
with db.session(commit_on_expire=False) as session:
session["key"] = "manual_value"
print(session.get("key")) # Output: None, not yet saved
session.commit() # Now changes are saved
print(session.get("key")) # Output: manual_value
with db.session() as session:
print(session.get("key")) # Output: manual_value
You can manually save or discard changes during a session:
with db.session() as session:
session["key"] = "temp_value"
session.rollback() # Discard changes
print(session.get("key")) # Output: None
with db.session() as session:
session["nested"] = {"key": [1, 2, 3]}
session.commit()
with db.session() as session:
print(session["nested"]) # Output: {"key": [1, 2, 3]}
from qsave.asyncio import AsyncQuickSave
db = AsyncQuickSave("path/to/your/file.json")
async def main():
async with db.session(False) as session:
print(len(session))
await session.commit()
await session.rollback() # NOTE: after commit, rollback does nothing :(
# only commit and rollback need to be awaited
# other functionalities remain the same as sync version
This repository is licensed under MIT License.
FAQs
QuickSave is a fast, memory-efficient, and lightweight key-value database.
We found that qsave demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.