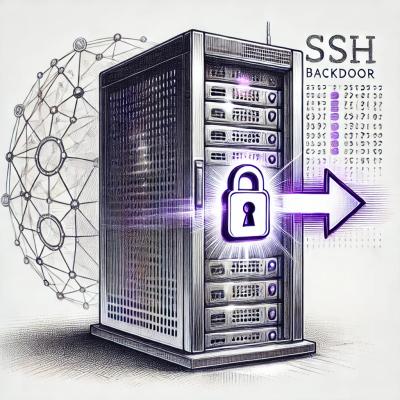
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A library which regulates traffic, with respect to concurrency or time. It implements sync and async context managers for a semaphore- and a token bucket-implementation.
The rate limiters are distributed, using Redis, and leverages Lua scripts to improve performance and simplify the code. Lua scripts run on Redis, and make each implementation fully atomic, while also reducing the number of round-trips required.
Use is supported for standalone redis instances, and clusters. We currently only support Python 3.11, but can add support for older versions if needed.
pip install redis-rate-limiters
The semaphore classes are useful when you have concurrency restrictions; e.g., say you're allowed 5 active requests at the time for a given API token.
Beware that the client will block until the Semaphore is acquired,
or the max_sleep
limit is exceeded. If the max_sleep
limit is exceeded, a MaxSleepExceededError
is raised.
Here's how you might use the async version:
import asyncio
from httpx import AsyncClient
from redis.asyncio import Redis
from limiters import AsyncSemaphore
limiter = AsyncSemaphore(
name="foo", # name of the resource you are limiting traffic for
capacity=5, # allow 5 concurrent requests
max_sleep=30, # raise an error if it takes longer than 30 seconds to acquire the semaphore
expiry=30, # set expiry on the semaphore keys in Redis to prevent deadlocks
connection=Redis.from_url("redis://localhost:6379"),
)
async def get_foo():
async with AsyncClient() as client:
async with limiter:
client.get(...)
async def main():
await asyncio.gather(
get_foo() for i in range(100)
)
and here is how you might use the sync version:
import requests
from redis import Redis
from limiters import SyncSemaphore
limiter = SyncSemaphore(
name="foo",
capacity=5,
max_sleep=30,
expiry=30,
connection=Redis.from_url("redis://localhost:6379"),
)
def main():
with limiter:
requests.get(...)
The TocketBucket
classes are useful if you're working with time-based
rate limits. Say, you are allowed 100 requests per minute, for a given API token.
If the max_sleep
limit is exceeded, a MaxSleepExceededError
is raised.
Here's how you might use the async version:
import asyncio
from httpx import AsyncClient
from redis.asyncio import Redis
from limiters import AsyncTokenBucket
limiter = AsyncTokenBucket(
name="foo", # name of the resource you are limiting traffic for
capacity=5, # hold up to 5 tokens
refill_frequency=1, # add tokens every second
refill_amount=1, # add 1 token when refilling
max_sleep=30, # raise an error there are no free tokens for 30 seconds
connection=Redis.from_url("redis://localhost:6379"),
)
async def get_foo():
async with AsyncClient() as client:
async with limiter:
client.get(...)
async def main():
await asyncio.gather(
get_foo() for i in range(100)
)
and here is how you might use the sync version:
import requests
from redis import Redis
from limiters import SyncTokenBucket
limiter = SyncTokenBucket(
name="foo",
capacity=5,
refill_frequency=1,
refill_amount=1,
max_sleep=30,
connection=Redis.from_url("redis://localhost:6379"),
)
def main():
with limiter:
requests.get(...)
We don't ship decorators in the package, but if you would like to limit the rate at which a whole function is run, you can create your own, like this:
from limiters import AsyncSemaphore
# Define a decorator function
def limit(name, capacity):
def middle(f):
async def inner(*args, **kwargs):
async with AsyncSemaphore(name=name, capacity=capacity):
return await f(*args, **kwargs)
return inner
return middle
# Then pass the relevant limiter arguments like this
@limit(name="foo", capacity=5)
def fetch_foo(id: UUID) -> Foo:
Contributions are very welcome. Here's how to get started:
pip install poetry
poetry install
pre-commit install
to set up pre-commitjust setup
If you prefer not to install just, just take a look at the justfile and
run the commands yourself.To publish a new version:
pyproject.toml
v0.4.2
)Once the release is published, our publish workflow should be triggered to push the new version to PyPI.
FAQs
Distributed rate limiters
We found that redis-rate-limiters demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.