replyowl: Email reply body generator for HTML and text in Python

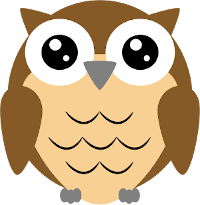
replyowl creates email bodies with quoted messages. Provide the original message
and your reply message, and replyowl will combine them into a new message. The
returned content can be used as the text and/or HTML body content of a new
email. HTML-to-text conversion is performed with html2text.
Installation
replyowl is available on PyPI:
pip install replyowl
Usage
from replyowl import ReplyOwl
owl = ReplyOwl()
text, html = owl.compose_reply(
content="<i>New</i> reply <b>content</b>",
quote_attribution="You wrote:",
quote_text="Original message text",
quote_html="<b>Original</b> message text",
)
print(text)
print(html)
Links in HTML are preserved when creating plain text email bodies:
from replyowl import ReplyOwl
owl = ReplyOwl()
text, html = owl.compose_reply(
content=(
'Check <a href="https://example.com/">this</a> out<br />'
'Or this: <a href="https://example.net/">https://example.net/</a>'
),
quote_attribution="You wrote:",
quote_text="Send me a URL",
quote_html="Send me a <i>URL</i>",
)
print(text)
If the quoted HTML content contains a <body>
tag, that is preserved:
from replyowl import ReplyOwl
owl = ReplyOwl()
text, html = owl.compose_reply(
content="Hello there",
quote_attribution="You wrote:",
quote_text="Hi",
quote_html='<html><body class="sender_body"><b>Hi</b></body></html>',
)
print(html)
A custom value can be provided for the <blockquote>
's style
tag:
from replyowl import ReplyOwl
owl = ReplyOwl(blockquote_style="font-weight: bold;")
text, html = owl.compose_reply(
text, html = owl.compose_reply(
content="Your quote is in bold",
quote_attribution="You wrote:",
quote_text="I'm going to be in bold when you reply",
quote_html="I'm going to be in bold when you reply",
)
)
print(html)
Development
Poetry installation
Via pipx
:
pip install pipx
pipx install poetry
pipx inject poetry poetry-pre-commit-plugin
Via pip
:
pip install poetry
poetry self add poetry-pre-commit-plugin
Development tasks
- Setup:
poetry install
- Run static checks:
poetry run poe lint
or
poetry run pre-commit run --all-files
- Run static checks and tests:
poetry run poe test
Created from smkent/cookie-python using
cookiecutter