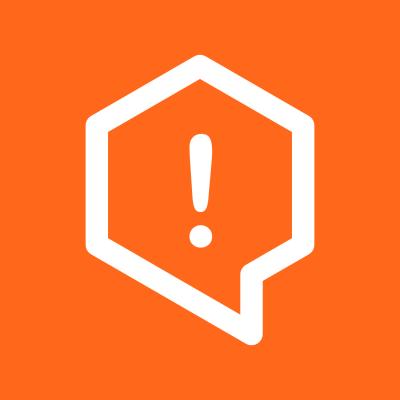
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
rocraters is a python library that is built upon a rust backend for interfacing with RO-Crates. This implementation was specifically created to address the challenges of data handling on automated robotic systems within an automated synthetic biology lab, however it's noted that this could have more general applicability to other environments.
It's aim is to provide a robust, portable and scalable solution to dealing with RO-Crates within the varying software environments of a synthetic biology lab stack. It's designed to be maximally flexible with minimal onboarding, allowing you to incorprate it into scrpits/ data pipelines as easily as possible. This also relies on you to have an understanding of the structure of an RO-Crate, but focuses more on the fact that some metadata is better than no metadata.
This is not the go-to python libary for RO-Crate interfacing, please see ro-crate-py for a full python implementation.
Built using PyO3 and maturin. Recommended to setup python venv, then install maturin (and remember maturin[patchelf])
pip install -i https://test.pypi.org/simple/ rocraters
The RO-Crate specification defines an RO-Crate as a JSON-LD file, consisting of a context and a graph. As such, in python it is a dictionary containing a "context" key, with some form of vocab context (default is the RO-Crate context) and a "graph" key, which contains a list of json objects (dictionaries).
To create an empty RO-Crate, you need to do the following:
from rocraters import PyRoCrateContext, PyRoCrate
# Define context
context = PyRoCrateContext.from_string(" https://w3id.org/ro/crate/1.1/context")
# Initialise empty crate
crate = PyRoCrate(context)
# For an easy start, you can make a default crate!
default_crate = PyRoCrate.new_default()
Now, there are 4 primary objects (dictionaries) that can be added to the crate:
These are all based upon the specification.
To populate the basic crate, with the essential keys to conform to specification:
# Continuation of the above examples
# Metadata descriptor
descriptor = {
"type": "CreativeWork",
"id": "ro-crate-metadata.json",
"conformsTo": {"id": "https://w3id.org/ro/crate/1.1"},
"about": {"id": "./"}
}
# Root data entity
root = {
"id": "./",
"type": "Dataset",
"datePublished": "2017",
"license": {"id": "https://creativecommons.org/licenses/by-nc-sa/3.0/au/"}
}
# Data entity
data = {
"id": "data_file.txt",
"type": "Dataset"
}
# Contextual entity
contextual = {
"id": "#JohnDoe",
"type": "Person",
}
# Update the RO-Crate object
crate.update_descriptor(descriptor)
crate.update_root(root)
crate.update_data(data)
crate.update_contextual(contextual)
To then write the crate to a ro-crate-metadata.json
file in the current working directory:
# Continuation of the above examples
# Write crate
crate.write()
To then read a ro-crate-metadata.json
file and load it in as a structured object:
# New example
from rocraters import read
# Read RO-Crate at specified path
crate = read("ro-crate-metadata.json", True)
To zip the folder and all contained directories within the ro-crate-metadata.json
directory:
# new example
from rocraters import zip
zip("ro-crate-metadata.json", True)
As per the libraries purpose, modification, ie the deletion, update and addition of entites is intended to be as simple as possible, whilst ensuring minimal conformance:
# Example based upon previously made crate
from rocraters import read
crate = read("ro-crate-metadata.json", True)
# Update the data entity and make modification
data_target = crate.get_entity("data_file.txt")
data_target["description"] = "A text file dataset containing information"
# Update the contextual entity and make modification
contextual_target = crate.get_entity("#JohnDoe")
contextual_target.update({"id" : "#JaneDoe"})
crate.update_contextual(contextual_target)
# To delete a key:value
data_target.pop("description")
# To delete an entity - this immediately updates the crate object
contextual_target.delete_entity("#JaneDoe")
# We then update the crate the same way we make it
# The ID will be used to serach the crate and overwrites the object with an indentical "id" key
crate.update_data(data_target)
crate.write()
PyO3 is used to handle python bindings. Maturin is used as the build tool.
FAQs
Lightweight Python library for RO-Crate manipulation implemented in Rust
We found that rocraters demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.