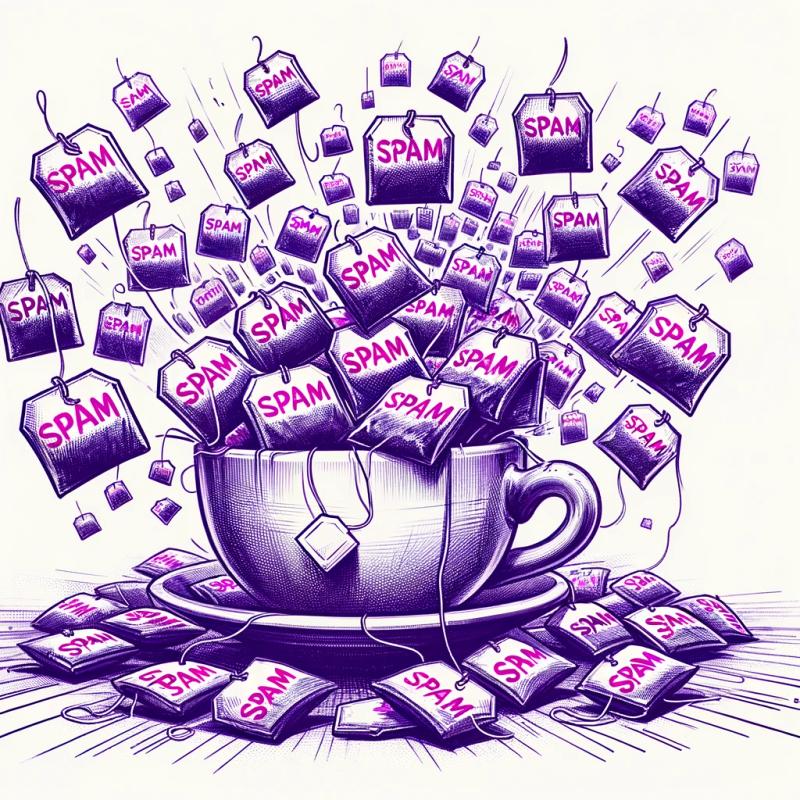
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Readme
Model CRUD manager to handle databases with asynchronous SQLAlchemy sessions.
⚠️ Warning: This project is currently in development phase.
This project is in an early stage of development and may contain bugs. It is not recommended for use in production environments.
sa_crudmodel
?Python 3.8+
SQLAlchemy Model CRUD stands on the soulders of giants:
$ pip install sa-modelcrud
from sqlalchemy.orm import Mapped, mapped_column
from sa_modelcrud.models import ModelBase, Timestamp
class Sample(Timestamp, ModelBase):
# ModelBase contains id and uid properties
email: Mapped[str] = mapped_column(nullable=True, unique=True)
from typing import Optional
from uuid import UUID
from pydantic import BaseModel
class SampleBase(BaseModel):
id: Optional[UUID] = None
email: Optional[str] = None
class SampleCreate(SampleBase):
...
class SampleUpdate(SampleBase):
...
from sa_modelcrud import CRUDBase
class CRUDSample(CRUDBase[Sample, SampleCreate, SampleUpdate]):
model = Sample
samples = CRUDSample()
from sqlalchemy.ext.asyncio import (
AsyncSession,
create_async_engine,
async_sessionmaker
)
DB_URI = "sqlite+aiosqlite:///./db.sqlite3"
async_engine: AsyncEngine = create_async_engine(
DB_URI,
future=True,
connect_args={"check_same_thread": False}
)
AsyncSessionLocal: AsyncSession = async_sessionmaker(
bind=async_engine,
class_=AsyncSession,
expire_on_commit=False
)
async with AsyncSessionLocal() as db:
data = SampleCreate(email="sample@sample")
# save data into database
sample_obj = await samples.create(db=db, element=data)
All inherited CRUDBase instances have the following methods:
.get(..., id)
: Get row from model by uid..get_or_raise(..., id)
: Try to get row from model by uid. Raise if not object found..list(...)
: Get multi items from database..filter(..., whereclause)
: Get items from database using whereclause
to filter..find(..., **kwargs)
: Find elements with kwargs..find_one(..., **kwargs)
: Find an element with kwargs..create(..., element)
: Create an element into database..bulk_create(..., elements)
: Create elements into database..update(..., obj, data)
: Update a database obj with an update data schema..delete(..., id)
: Delete an item from database.list
, filter
and find
.offset
and limit
on paginated methods.I would love to receive contributions and feedback! If you'd like to get involved, please contact me through one of the contact methods in my Profile.
This project is licensed under the terms of the MIT license.
FAQs
Model CRUD manager to handle databases with asynchronous SQLAlchemy sessions
We found that sa-modelcrud demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.