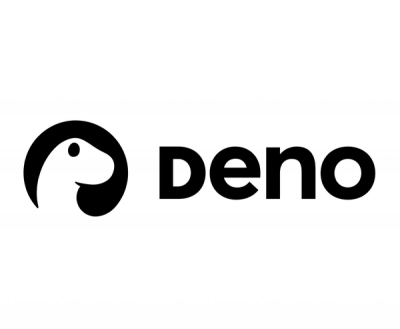
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Sockslib is a library designed to make the usage of socks proxies as easy as possible. This library can connect to proxies, authenticate, and then have the use of a normal python socket.
socket = sockslib.SocksSocket()
socket.set_proxy (
('127.0.0.1', 0), # Ip, Port
sockslib.Socks.SOCKS5, # SOCKS5/SOCKS4, (Optional) (Default SOCKS5)
authentication # Array of authentication methods (Optional) (Default NoAuth)
)
Default proxies can be useful for situations when other libraries use sockets to communicate and you want to force that library to use a proxy instead of a direct connection
sockslib.set_default_proxy(
('127.0.0.1', 0), # Ip, Port
sockslib.Socks.SOCKS5, # SOCKS5/SOCKS4, (Optional) (Default SOCKS5)
socket.AF_INET, # Default protocol (AF_INET, AF_INET6)
authentication # Array of authentication methods (Optional) (Default NoAuth)
)
import urllib.request
import sockslib
import socket
sockslib.set_default_proxy(('127.0.0.1', 9050)) # Set the default proxy
socket.socket = sockslib.SocksSocket # Make the default socket object a SocksSocket
html = urllib.request.urlopen('https://myexternalip.com/raw').read() # Request the page
print(html)
To connect to a proxy server via ipv6, you must explicitly specify the protocol by passing the ip_protocol
parameter to the SocksSocket constructor, OR by using the 3rd option of set_default_proxy.
Here is an example of connecting to a remote IPv6 proxy:
import sockslib
import socket # import socket for the protocol specifiers
with sockslib.SocksSocket(ip_protocol=socket.AF_INET6) as sock:
sock.set_proxy(('::1', 9050)) # Set proxy
sock.connect(('myexternalip.com', 80)) # Connect to Server via proxy
sock.sendall(b"GET /raw HTTP/1.1\r\nHost: myexternalip.com\r\n\r\n") # Send HTTP Request
print(sock.recv(1024)) # Print response
This is an example usage that connects to a Socks5 proxy at 127.0.0.1:9050
and then requests the page http://myexternalip.com/raw
import sockslib
with sockslib.SocksSocket() as sock:
sock.set_proxy(('127.0.0.1', 9050)) # Set proxy
sock.connect(('myexternalip.com', 80)) # Connect to Server via proxy
sock.sendall(b"GET /raw HTTP/1.1\r\nHost: myexternalip.com\r\n\r\n") # Send HTTP Request
print(sock.recv(1024)) # Print response
This is an example usage that connects to a Socks5 proxy at 127.0.0.1:9050
and then sends a UDP packet.
import sockslib
with sockslib.SocksSocket(udp=True) as sock:
sock.set_proxy(('127.0.0.1', 9050)) # Set proxy
sock.initudp() # Connect to Server and bind a UDP port for communication
sock.sendto(b"Hello, World", ("0.0.0.0", 12000)) # Send "Hello, World" to 0.0.0.0:12000 over UDP
Due to the nature of UDP in the SOCKS5 protocol, it is reccomended to use the context manager, as the remote proxy server will not drop the UDP associate request until the socket is closed.
To use more authentication methods like User/Pass auth, you pass an array of authentication methods to the third parameter of set_proxy
(Don't neglect to set the second parameter to the proxy type!)
import sockslib
with sockslib.SocksSocket() as sock:
auth_methods = [
sockslib.NoAuth(), # No authentication
sockslib.UserPassAuth('username', 'password'), # Username / Password authentication
]
sock.set_proxy(('127.0.0.1', 9050), sockslib.Socks.SOCKS5, auth_methods) # Set proxy
sock.connect(('myexternalip.com', 80)) # Connect to Server via proxy
sock.sendall(b"GET /raw HTTP/1.1\r\nHost: myexternalip.com\r\n\r\n") # Send HTTP Request
print(sock.recv(1024)) # Print response
To implement your own socks5 authentication method, you must make a class that implements sockslib.AuthenticationMethod
it requires that you implement a getId()
function, an authenticate(socket)
function, and a forP
function. Note: the authenticate function must return a boolean, True if authentication succeeded and False if it failed.
from sockslib.socks import AuthenticationMethod
from sockslib.socks import Socks
import struct
class UserPassAuth(AuthenticationMethod):
def __init__(self, username, password):
self.username = username
self.password = password
def getId(self):
return 0x02 # 0x02 means password authentication, see https://en.wikipedia.org/wiki/SOCKS#SOCKS5 for more
def forP(self):
return Socks.SOCKS5 # For SOCKS5
def authenticate(self, socket):
socket.sendall(b"\x01" + struct.pack("B", len(self.username)) + self.username.encode() + struct.pack("B", len(self.password)) + self.password.encode()) # Send authentication packet
ver, status = socket.recv(2) # Get authentication response
return status == 0x00
This is an example usage that connects to a Socks4 proxy at 127.0.0.1:9050
and then requests the page http://myexternalip.com/raw
import sockslib
with sockslib.SocksSocket() as sock:
sock.set_proxy(('127.0.0.1', 9050), sockslib.Socks.SOCKS4) # Set proxy
sock.connect(('myexternalip.com', 80)) # Connect to Server via proxy
sock.sendall(b"GET /raw HTTP/1.1\r\nHost: myexternalip.com\r\n\r\n") # Send HTTP Request
print(sock.recv(1024)) # Print response
import sockslib
with sockslib.SocksSocket() as sock:
auth_methods = [
sockslib.Socks4Ident("ident")
]
sock.set_proxy(('127.0.0.1', 9050), sockslib.Socks.SOCKS4, auth_methods) # Set proxy
sock.connect(('myexternalip.com', 80)) # Connect to Server via proxy
sock.sendall(b"GET /raw HTTP/1.1\r\nHost: myexternalip.com\r\n\r\n") # Send HTTP Request
print(sock.recv(1024)) # Print response
pip3 install sockslib
If you have any issues with this project please feel free to open a new issue on github https://github.com/woo200/sockslib/issues
FAQs
Simple Socks proxy library
We found that sockslib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.