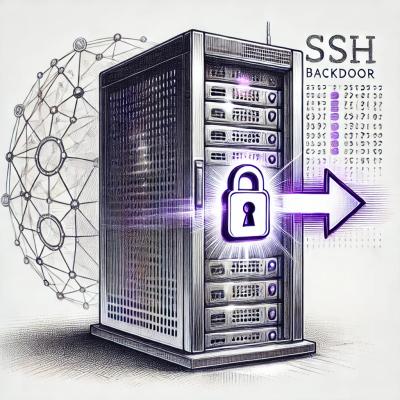
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
This library is a full python implementation of Bernard Chazelle's soft heap [1].
The soft heap is a heap-like data structure which gives all heap operations a constant runtime, with the exception of insert. However, in order to maintain this seemingly impossible runtime, some of the data is "corrupted". The result of this is that insert takes O(log 1/e) where e is the rate of corruption.
[1] http://www.cs.cmu.edu/afs/cs/academic/class/15859-f05/www/documents/p1012-chazelle.pdf
To get started, import the module. We'll also import random for this example.
from softheap import *
import random
The soft heap requires one input, the error rate r. The number of corrupted nodes in the heap is inversely proportional to r.
s = SoftHeap(2)
We can test the heap by randomly inserting 100 elements into the heap.
arr = list(range(100))
random.shuffle(arr)
for a in arr:
s.insert(a)
Now when we deletemin repeatedly, the output will be approximately sorted.
output = []
for b in range(len(arr))
output.append(s.deletemin())
print(output)
This takes O(n log 1/e) time.
Use pip to install softheap. Via the command line:
pip install softheap
FAQs
Python Soft Heap Implementation
We found that softheap demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.