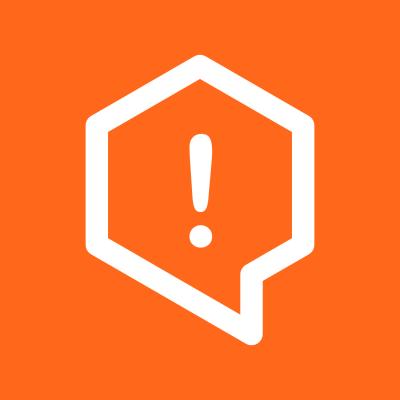
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Sub-class of Python str
that adds case conversion functions. They are especially useful for transforming data model field names, ex: REST (camelCase) to Python (snake_case).
From the command line:
pip install stringbender
or if you're using poetry:
poetry add stringbender
StringBender provides the following static functions for converting the case of a specified string:
These helper methods call a corresponding method in the stringbender.String
class and converts the output to str
.
from stringbender import camel, kebob, pascal, snake, String
# ================================================================================
# EXAMPLES # OUTPUT
s = "Hasta la vista baby"
print(camel(s)) # hastaLaVistaBaby
print(kebob(s) # hasta-la-vista-baby
print(pascal(s)) # HastaLaVistaBaby
print(snake(s)) # hasta_la_vista_baby
# ================================================================================
# Using a StringBender method with a built-in function
# Create an instance of stringbender.String:
s = String("vote*for*pedro")
# Check the default output:
print(s.camel()) # vote*For*Pedro (hmm... this isn't right)
# Pass in a custom delimiter:
print(s.replace("*", " ").camel()) # voteForPedro (Much better!)
# ================================================================================
# Using a list of delimiters
s = snake("Careful man, there's a beverage here!", delimiters=[",", "'", "!"])
print(snake(s)) # careful_man_there_s_a_beverage_here
stringbender.String
Methods
Optional argument definitions below
camel()
(String) :Combines all words and he first letter of the first word is lower case, while the first letter of every subsequent word is uppercase.
kebob()
(String)Creates a hyphen delimited lower-case string.
pascal()
(String)Combines all words, and capitalizes the first letter of each word.
snake()
(String)Creates an underscore delimited lower-case string.
as_str()
(str)Returns the value as a str
. This is the same as str(String(...))
DEFAULT_DELIMITERS: List[str] = [" ", ".", "-", "_", ":", "\\"]
delimiters: List[str] = DEFAULT_DELIMITERS
Used to split the string into words.
split_on_first_upper: bool
Splits on the first occurence of a capital letter following a lowercase letter.
title_case: bool
For character-delimited strings (kebob, snake), This will capitalize the first letter of each word.
FAQs
A sub-class of the Python str class that adds case conversion functions.
We found that stringbender demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.