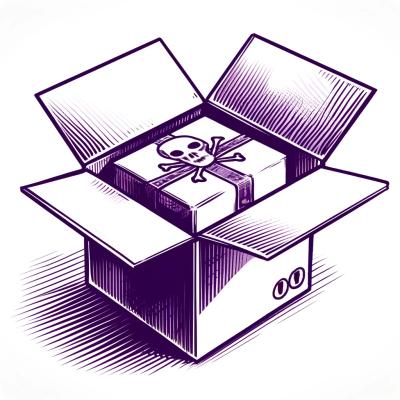
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
The Ruby SDK of 51Tracking API
Contact: service@51tracking.org
gem install 51tracking
require '51tracking'
Tracking51.api_key = 'you api key'
begin
response = Tracking51::Courier.get_all_couriers
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
rspec
Throw by the new SDK client
require '51tracking'
Tracking51.api_key = ''
begin
response = Tracking51::Courier.get_all_couriers
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
end
# API Key is missing
Throw by the parameter validation in function
require '51tracking'
Tracking51.api_key = 'you api key'
begin
params = {"tracking_number" => "","courier_code"=>"usps"}
response = Tracking51::Tracking.create_tracking(params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
end
# Tracking number cannot be empty
https://api.51Tracking.com/v4/couriers/all
begin
response = Tracking51::Courier.get_all_couriers
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/create
begin
params = {"tracking_number" => "92612913029511573130094547","courier_code"=>"usps"}
response = Tracking51::Tracking.create_tracking(params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/get
begin
# Perform queries based on various conditions
# params = {"tracking_numbers" => "92612903029511573130094547","courier_code"=>"usps"}
# params = {"tracking_numbers" => "92612903029511573130094547,92612903029511573030094548","courier_code"=>"usps"}
params = {"created_date_min" => "2023-08-23T14:00:00+00:00","created_date_max"=>"2023-08-23T15:04:00+00:00"}
response = Tracking51::Tracking.get_tracking_results(params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/batch
begin
params = [{"tracking_number" => "92612903029611573130094547","courier_code"=>"usps"},{"tracking_number" => "92612903029711573130094547","courier_code"=>"usps"}]
response = Tracking51::Tracking.batch_create_trackings(params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/update/{id}
begin
params = {"customer_name" => "New name","note"=>"New tests order note"}
id_string = '9a3aec583781c7096cf744d68287d3d1'
response = Tracking51::Tracking.update_tracking_by_id(id_string, params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/delete/{id}
begin
id_string = '9a3aec583781c7096cf744d68287d3d1'
response = Tracking51::Tracking.delete_tracking_by_id(id_string)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/trackings/retrack/{id}
begin
id_string = '9a3aec583781c7096cf744d68287d3d1'
response = Tracking51::Tracking.retrack_tracking_by_id(id_string)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
https://api.51Tracking.com/v4/awb
begin
params = {"awb_number" => "235-69030430"}
response = Tracking51::AirWaybill.create_an_air_waybill(params)
puts response
rescue Tracking51::Tracking51Exception => e
puts "Caught Custom Exception: #{e.message}"
rescue StandardError => e
puts "Caught Standard Error: #{e.message}"
end
51Tracking 使用传统的HTTP状态码来表明 API 请求的状态。通常,2xx形式的状态码表示请求成功,4XX形式的状态码表请求发生错误(比如:必要参数缺失),5xx格式的状态码表示 51tracking 的服务器可能发生了问题。
Http CODE | META CODE | TYPE | MESSAGE |
---|---|---|---|
200 | 200 | 成功 | 请求响应成功。 |
400 | 400 | 错误请求 | 请求类型错误。请查看 API 文档以了解此 API 的请求类型。 |
400 | 4101 | 错误请求 | 物流单号已存在。 |
400 | 4102 | 错误请求 | 物流单号不存在。请先使用「Create接口」将单号添加至系统。 |
400 | 4103 | 错误请求 | 您已超出 API 调用的创建数量。每次创建的最大数量为 40 个快递单号。 |
400 | 4110 | 错误请求 | 物流单号(tracking_number) 不符合规则。 |
400 | 4111 | 错误请求 | 物流单号(tracking_number)为必填字段。 |
400 | 4112 | 错误请求 | 查询ID无效。 |
400 | 4113 | 错误请求 | 不允许重新查询。您只能重新查询过期的物流单号。 |
400 | 4120 | 错误请求 | 物流商简码(courier_code)的值无效。 |
400 | 4121 | 错误请求 | 无法识别物流商。 |
400 | 4122 | 错误请求 | 特殊物流商字段缺失或填写不符合规范。 |
400 | 4130 | 错误请求 | 请求参数的格式无效。 |
400 | 4160 | 错误请求 | 空运单号(awb_number)是必需的或有效的格式。 |
400 | 4161 | 错误请求 | 此空运航空不支持查询。 |
400 | 4165 | 错误请求 | 查询失败:未创建空运单号。 |
400 | 4166 | 错误请求 | 删除未创建的空运单号失败。 |
400 | 4167 | 错误请求 | 空运单号已存在,无需再次创建。 |
400 | 4190 | 错误请求 | 当前查询额度不足。 |
401 | 401 | 未经授权 | 身份验证失败或没有权限。请检查并确保您的 API 密钥正确无误。 |
403 | 403 | 禁止 | 禁止访问。请求被拒绝或不允许访问。 |
404 | 404 | 未找到 | 页面不存在。请检查并确保您的链接正确无误。 |
429 | 429 | 太多请求 | 超出 API 请求限制,请稍后重试。请查看 API 文档以了解此 API 的限制。 |
500 | 511 | 服务器错误 | 服务器错误。请联系我们: service@51Tracking.org。 |
500 | 512 | 服务器错误 | 服务器错误。请联系我们:service@51Tracking.org。 |
500 | 513 | 服务器错误 | 服务器错误。请联系我们: service@51Tracking.org。 |
FAQs
Unknown package
We found that 51tracking demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.