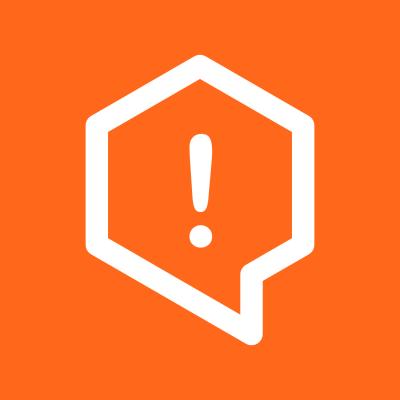
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
API for sending and managing payments
Install the gem from the command line:
gem install apimatic-ci-cd-test -v 1.0.24
Or add the gem to your Gemfile and run bundle
:
gem 'apimatic-ci-cd-test', '1.0.24'
For additional gem details, see the RubyGems page for the apimatic-ci-cd-test gem.
The following parameters are configurable for the API Client:
Parameter | Type | Description |
---|---|---|
x_api_key | String | API Key |
timeout | Float | The value to use for connection timeout. Default: 60 |
max_retries | Integer | The number of times to retry an endpoint call if it fails. Default: 0 |
retry_interval | Float | Pause in seconds between retries. Default: 1 |
backoff_factor | Float | The amount to multiply each successive retry's interval amount by in order to provide backoff. Default: 2 |
retry_statuses | Array | A list of HTTP statuses to retry. Default: [408, 413, 429, 500, 502, 503, 504, 521, 522, 524] |
retry_methods | Array | A list of HTTP methods to retry. Default: %i[get put] |
The API client can be initialized as follows:
client = PaymentsApi::Client.new(
x_api_key: 'x-api-key',
)
This API uses Custom Header Signature
.
The gateway for the SDK. This class acts as a factory for the Controllers and also holds the configuration of the SDK.
Name | Description |
---|---|
quotes | Gets QuotesController |
payments | Gets PaymentsController |
Quotes
An instance of the QuotesController
class can be accessed from the API Client.
quotes_controller = client.quotes
Create new Quote
def create_new_quote(bank_id,
body)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
body | Quote | Body, Required | Quote data |
bank_id = 'bankId0'
body = Quote.new
body.id = 0
body.beneficiary_amount = 12345.67
body.beneficiary_currency = 'EUR'
body.originator_amount = 76543.21
body.originator_amount_is_fixed = true
body.exchange_rate = 1.2345
body.locked = false
body.revision = 1
result = quotes_controller.create_new_quote(bank_id, body)
{
"id": 0,
"beneficiaryAmount": 12345.67,
"beneficiaryCurrency": "EUR",
"originatorAmount": 76543.21,
"originatorAmountIsFixed": true,
"exchangeRate": 1.2345,
"locked": false,
"revision": 1
}
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Attempts to cancel a Quote
def cancel_quote(bank_id,
quote_id)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
quote_id | Integer | Template, Required | ID of quote to refresh |
void
bank_id = 'bankId0'
quote_id = 124
result = quotes_controller.cancel_quote(bank_id, quote_id)
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Lock the rate for a given Quote
def lock_quote(bank_id,
quote_id)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
quote_id | Integer | Template, Required | ID of quote to lock |
void
bank_id = 'bankId0'
quote_id = 124
result = quotes_controller.lock_quote(bank_id, quote_id)
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Refresh the rates for an existing Quote
def refresh_quote(bank_id,
quote_id)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
quote_id | Integer | Template, Required | ID of quote to refresh |
bank_id = 'bankId0'
quote_id = 124
result = quotes_controller.refresh_quote(bank_id, quote_id)
{
"id": 0,
"beneficiaryAmount": 12345.67,
"beneficiaryCurrency": "EUR",
"originatorAmount": 76543.21,
"originatorAmountIsFixed": true,
"exchangeRate": 1.2345,
"locked": false,
"revision": 1
}
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Payments
An instance of the PaymentsController
class can be accessed from the API Client.
payments_controller = client.payments
Creates a new Payment
def create_payment(bank_id,
body)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
body | Payment | Body, Required | Create Payment Body Data |
bank_id = 'bankId0'
body = Payment.new
body.id = 987654
body.quote_id = 123456
body.originator = Originator.new
body.originator.name = 'Jake Johnson'
body.originator.address = Address.new
body.originator.address.address1 = '555 South North Lane'
body.originator.address.address2 = 'Floor 5'
body.originator.address.city = 'Springfield'
body.originator.address.state = 'VA'
body.originator.address.postal_code = '47060'
body.originator.address.country = 'US'
body.originator_bank_account = BankAccount.new
body.originator_bank_account.account_number = 'DE89370400440532013000'
body.originator_bank_account.bank = Bank.new
body.originator_bank_account.bank.name = 'Bank of America'
body.originator_bank_account.bank.address = Address.new
body.originator_bank_account.bank.address.address1 = '555 South North Lane'
body.originator_bank_account.bank.address.address2 = 'Floor 5'
body.originator_bank_account.bank.address.city = 'Springfield'
body.originator_bank_account.bank.address.state = 'VA'
body.originator_bank_account.bank.address.postal_code = '47060'
body.originator_bank_account.bank.address.country = 'US'
body.originator_bank_account.bank.routing_code = 'string'
body.originator_bank_account.bank.swift_code = 'AGCAAM22'
body.beneficiary = Beneficiary.new
body.beneficiary.name = 'Jake Johnson'
body.beneficiary.address = Address.new
body.beneficiary.address.address1 = '555 South North Lane'
body.beneficiary.address.address2 = 'Floor 5'
body.beneficiary.address.city = 'Springfield'
body.beneficiary.address.state = 'VA'
body.beneficiary.address.postal_code = '47060'
body.beneficiary.address.country = 'US'
body.beneficiary.email = 'beneficiary@example.com'
body.beneficiary.phone_number = '111-111-1111'
body.beneficiary_bank_account = BankAccount.new
body.beneficiary_bank_account.account_number = 'DE89370400440532013000'
body.beneficiary_bank_account.bank = Bank.new
body.beneficiary_bank_account.bank.name = 'Bank of America'
body.beneficiary_bank_account.bank.address = Address.new
body.beneficiary_bank_account.bank.address.address1 = '555 South North Lane'
body.beneficiary_bank_account.bank.address.address2 = 'Floor 5'
body.beneficiary_bank_account.bank.address.city = 'Springfield'
body.beneficiary_bank_account.bank.address.state = 'VA'
body.beneficiary_bank_account.bank.address.postal_code = '47060'
body.beneficiary_bank_account.bank.address.country = 'US'
body.beneficiary_bank_account.bank.routing_code = 'string'
body.beneficiary_bank_account.bank.swift_code = 'AGCAAM22'
body.intermediary_bank_account = BankAccount.new
body.intermediary_bank_account.account_number = 'DE89370400440532013000'
body.intermediary_bank_account.bank = Bank.new
body.intermediary_bank_account.bank.name = 'Bank of America'
body.intermediary_bank_account.bank.address = Address.new
body.intermediary_bank_account.bank.address.address1 = '555 South North Lane'
body.intermediary_bank_account.bank.address.address2 = 'Floor 5'
body.intermediary_bank_account.bank.address.city = 'Springfield'
body.intermediary_bank_account.bank.address.state = 'VA'
body.intermediary_bank_account.bank.address.postal_code = '47060'
body.intermediary_bank_account.bank.address.country = 'US'
body.intermediary_bank_account.bank.routing_code = 'string'
body.intermediary_bank_account.bank.swift_code = 'AGCAAM22'
body.details = PaymentDetails.new
body.details.purpose_of_payment = 'Purchase of Goods'
body.details.memo = 'Invoice 12345678'
body.details.payment_reference = '1234567890'
body.status = PaymentStatus.new
body.status.approved = false
body.status.sent = false
result = payments_controller.create_payment(bank_id, body)
{
"id": 987654,
"quoteId": 123456,
"originator": {
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
}
},
"originatorBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"beneficiary": {
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"email": "beneficiary@example.com",
"phoneNumber": "111-111-1111"
},
"beneficiaryBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"intermediaryBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"details": {
"purposeOfPayment": "Purchase of Goods",
"memo": "Invoice 12345678",
"paymentReference": "1234567890"
},
"status": {
"approved": false,
"sent": false
}
}
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Attempts to cancel a Payment. Does not automatically cancel the linked Quote.
def cancel_payment(bank_id,
payment_id)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
payment_id | Integer | Template, Required | ID of payment to cancel |
void
bank_id = 'bankId0'
payment_id = 250
result = payments_controller.cancel_payment(bank_id, payment_id)
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Update the data for a Payment before it is approved or sent
def update_payment(bank_id,
payment_id,
body)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
payment_id | Integer | Template, Required | ID of payment to update |
body | Payment | Body, Required | Update Payment Body Data |
bank_id = 'bankId0'
payment_id = 250
body = Payment.new
body.id = 987654
body.quote_id = 123456
body.originator = Originator.new
body.originator.name = 'Jake Johnson'
body.originator.address = Address.new
body.originator.address.address1 = '555 South North Lane'
body.originator.address.address2 = 'Floor 5'
body.originator.address.city = 'Springfield'
body.originator.address.state = 'VA'
body.originator.address.postal_code = '47060'
body.originator.address.country = 'US'
body.originator_bank_account = BankAccount.new
body.originator_bank_account.account_number = 'DE89370400440532013000'
body.originator_bank_account.bank = Bank.new
body.originator_bank_account.bank.name = 'Bank of America'
body.originator_bank_account.bank.address = Address.new
body.originator_bank_account.bank.address.address1 = '555 South North Lane'
body.originator_bank_account.bank.address.address2 = 'Floor 5'
body.originator_bank_account.bank.address.city = 'Springfield'
body.originator_bank_account.bank.address.state = 'VA'
body.originator_bank_account.bank.address.postal_code = '47060'
body.originator_bank_account.bank.address.country = 'US'
body.originator_bank_account.bank.routing_code = 'string'
body.originator_bank_account.bank.swift_code = 'AGCAAM22'
body.beneficiary = Beneficiary.new
body.beneficiary.name = 'Jake Johnson'
body.beneficiary.address = Address.new
body.beneficiary.address.address1 = '555 South North Lane'
body.beneficiary.address.address2 = 'Floor 5'
body.beneficiary.address.city = 'Springfield'
body.beneficiary.address.state = 'VA'
body.beneficiary.address.postal_code = '47060'
body.beneficiary.address.country = 'US'
body.beneficiary.email = 'beneficiary@example.com'
body.beneficiary.phone_number = '111-111-1111'
body.beneficiary_bank_account = BankAccount.new
body.beneficiary_bank_account.account_number = 'DE89370400440532013000'
body.beneficiary_bank_account.bank = Bank.new
body.beneficiary_bank_account.bank.name = 'Bank of America'
body.beneficiary_bank_account.bank.address = Address.new
body.beneficiary_bank_account.bank.address.address1 = '555 South North Lane'
body.beneficiary_bank_account.bank.address.address2 = 'Floor 5'
body.beneficiary_bank_account.bank.address.city = 'Springfield'
body.beneficiary_bank_account.bank.address.state = 'VA'
body.beneficiary_bank_account.bank.address.postal_code = '47060'
body.beneficiary_bank_account.bank.address.country = 'US'
body.beneficiary_bank_account.bank.routing_code = 'string'
body.beneficiary_bank_account.bank.swift_code = 'AGCAAM22'
body.intermediary_bank_account = BankAccount.new
body.intermediary_bank_account.account_number = 'DE89370400440532013000'
body.intermediary_bank_account.bank = Bank.new
body.intermediary_bank_account.bank.name = 'Bank of America'
body.intermediary_bank_account.bank.address = Address.new
body.intermediary_bank_account.bank.address.address1 = '555 South North Lane'
body.intermediary_bank_account.bank.address.address2 = 'Floor 5'
body.intermediary_bank_account.bank.address.city = 'Springfield'
body.intermediary_bank_account.bank.address.state = 'VA'
body.intermediary_bank_account.bank.address.postal_code = '47060'
body.intermediary_bank_account.bank.address.country = 'US'
body.intermediary_bank_account.bank.routing_code = 'string'
body.intermediary_bank_account.bank.swift_code = 'AGCAAM22'
body.details = PaymentDetails.new
body.details.purpose_of_payment = 'Purchase of Goods'
body.details.memo = 'Invoice 12345678'
body.details.payment_reference = '1234567890'
body.status = PaymentStatus.new
body.status.approved = false
body.status.sent = false
result = payments_controller.update_payment(bank_id, payment_id, body)
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Approves a Payment to be sent
def approve_payment(bank_id,
payment_id)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
payment_id | Integer | Template, Required | ID of payment to approve |
void
bank_id = 'bankId0'
payment_id = 250
result = payments_controller.approve_payment(bank_id, payment_id)
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Validates an IBAN and returns the bank account information
def validate_iban(bank_id,
iban)
Parameter | Type | Tags | Description |
---|---|---|---|
bank_id | String | Header, Required | Bank ID (Routing Number) Constraints: Pattern: ^\d{9}$ |
iban | String | Query, Required | Currency that is required by the client, sell foreign currency in exchange for local currency Constraints: Minimum Length: 10 , Maximum Length: 35 |
bank_id = 'bankId0'
iban = 'iban4'
result = payments_controller.validate_iban(bank_id, iban)
{
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
}
HTTP Status Code | Error Description | Exception Class |
---|---|---|
400 | Error in Request | RequestErrorException |
403 | Forbidden | APIException |
500 | System Error | RequestErrorException |
Address Information
Address
Name | Type | Tags | Description |
---|---|---|---|
address_1 | String | Required | Address Line 1 Constraints: Maximum Length: 35 |
address_2 | String | Optional | Address Line 2 Constraints: Maximum Length: 35 |
city | String | Required | City Constraints: Maximum Length: 35 |
state | String | Optional | State / Province Constraints: Maximum Length: 60 |
postal_code | String | Required | Postal Code Constraints: Maximum Length: 12 |
country | String | Required | Country (ISO 3166-1 Alpha-2 Code) Constraints: Minimum Length: 2 , Maximum Length: 2 , Pattern: ^[A-Z]{2}$ |
{
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
}
Bank Information Object
Bank
Name | Type | Tags | Description |
---|---|---|---|
name | String | Required | Bank Name Constraints: Maximum Length: 50 |
address | Address | Required | Address Information |
routing_code | String | Optional | Routing Code. Currently only supported for USD wires. When using, please only provide SWIFT code OR routing code (not both) Constraints: Maximum Length: 35 |
swift_code | String | Optional | SWIFT BIC Constraints: Minimum Length: 8 , Maximum Length: 11 , Pattern: ^[A-Za-z0-9]{4}[A-Za-z]{2}[A-Za-z0-9]{2}([A-Za-z0-9]{3})?$ |
{
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
Bank Account Information Object.NOTE - originatorBankAccount bank data should not be provided when creating a new Payment. This information is retrieved from the database based on the provided bank ID.NOTE - bank object is required for all BankAccount objects except originatorBankAccount
BankAccount
Name | Type | Tags | Description |
---|---|---|---|
account_number | String | Optional | Bank Account Number / IBAN. Required for Beneficiary Bank. Optional for Intermediary Bank. Constraints: Maximum Length: 35 |
bank | Bank | Optional | Bank Information Object |
{
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
}
Beneficiary Information Object
Beneficiary
Name | Type | Tags | Description |
---|---|---|---|
name | String | Required | Beneficiary Name Constraints: Maximum Length: 80 |
address | Address | Required | Address Information |
email | String | Optional | Beneficiary Email Address Constraints: Maximum Length: 35 |
phone_number | String | Optional | Beneficiary Phone Number Constraints: Maximum Length: 35 |
{
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"email": "beneficiary@example.com",
"phoneNumber": "111-111-1111"
}
Originator Information Object
Originator
Name | Type | Tags | Description |
---|---|---|---|
name | String | Required | Originator Name Constraints: Maximum Length: 80 |
address | Address | Required | Address Information |
{
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
}
}
Payment Information Object
Payment
Name | Type | Tags | Description |
---|---|---|---|
id | Integer | Optional | Payment ID |
quote_id | Integer | Required | Quote ID |
originator | Originator | Required | Originator Information Object |
originator_bank_account | BankAccount | Optional | Bank Account Information Object.NOTE - originatorBankAccount bank data should not be provided when creating a new Payment. This information is retrieved from the database based on the provided bank ID.NOTE - bank object is required for all BankAccount objects except originatorBankAccount |
beneficiary | Beneficiary | Required | Beneficiary Information Object |
beneficiary_bank_account | BankAccount | Required | Bank Account Information Object.NOTE - originatorBankAccount bank data should not be provided when creating a new Payment. This information is retrieved from the database based on the provided bank ID.NOTE - bank object is required for all BankAccount objects except originatorBankAccount |
intermediary_bank_account | BankAccount | Optional | Bank Account Information Object.NOTE - originatorBankAccount bank data should not be provided when creating a new Payment. This information is retrieved from the database based on the provided bank ID.NOTE - bank object is required for all BankAccount objects except originatorBankAccount |
details | PaymentDetails | Required | Payment Information Data |
status | PaymentStatus | Optional | Payment Information Data |
{
"id": 987654,
"quoteId": 123456,
"originator": {
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
}
},
"originatorBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"beneficiary": {
"name": "Jake Johnson",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"email": "beneficiary@example.com",
"phoneNumber": "111-111-1111"
},
"beneficiaryBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"intermediaryBankAccount": {
"accountNumber": "DE89370400440532013000",
"bank": {
"name": "Bank of America",
"address": {
"address1": "555 South North Lane",
"address2": "Floor 5",
"city": "Springfield",
"state": "VA",
"postalCode": "47060",
"country": "US"
},
"routingCode": "string",
"swiftCode": "AGCAAM22"
}
},
"details": {
"purposeOfPayment": "Purchase of Goods",
"memo": "Invoice 12345678",
"paymentReference": "1234567890"
},
"status": {
"approved": false,
"sent": false
}
}
Payment Information Data
PaymentDetails
Name | Type | Tags | Description |
---|---|---|---|
purpose_of_payment | String | Required | Purpose of payment Constraints: Maximum Length: 30 |
memo | String | Optional | Memo from Originator to Beneficiary Constraints: Maximum Length: 140 |
payment_reference | String | Optional | Payment Reference Information. Typically includes FI to FI notes Constraints: Maximum Length: 140 |
{
"purposeOfPayment": "Purchase of Goods",
"memo": "Invoice 12345678",
"paymentReference": "1234567890"
}
Payment Information Data
PaymentStatus
Name | Type | Tags | Description |
---|---|---|---|
approved | Boolean | Optional | Set to true once the payment has been approved |
sent | Boolean | Optional | Set to true once the payment has been sent |
{
"approved": false,
"sent": false
}
Quote Information Object
Quote
Name | Type | Tags | Description |
---|---|---|---|
id | Integer | Optional | Quote ID |
beneficiary_amount | Float | Optional | Amount to send in beneficiary currency. Not required if originatorAmount is provided. |
beneficiary_currency | String | Required | Beneficiary currency code in ISO 4217 format Constraints: Minimum Length: 3 , Maximum Length: 3 , Pattern: ^[A-Z]{3}$ |
originator_amount | Float | Optional | Amount to send in originator currency. Not required if beneficiaryAmount is provided |
originator_amount_is_fixed | Boolean | Optional | If true, then the originator amount is fixed to the provided value. If false, then the beneficiary amount is fixed to the provided value. This field is automatically set based on whether the originator or beneficary amount was provided. |
exchange_rate | Float | Optional | The exchange rate for the quote |
locked | Boolean | Optional | Set to true if the quote rate is locked |
revision | Integer | Optional | Quote revision number. This is automatically incremented each time the quote is refreshed or updated, and starts from 1 |
{
"id": 0,
"beneficiaryAmount": 12345.67,
"beneficiaryCurrency": "EUR",
"originatorAmount": 76543.21,
"originatorAmountIsFixed": true,
"exchangeRate": 1.2345,
"locked": false,
"revision": 1
}
Format for 400 Errors
RequestErrorException
Name | Type | Tags | Description |
---|---|---|---|
message | String | Optional | Message indicating the source of the error in the request |
{
"message": "Error occured while performing field validation"
}
API utility class.
Name | Return Type | Description |
---|---|---|
json_deserialize | Hash | Deserializes a JSON string to a Ruby Hash. |
rfc3339 | DateTime | Safely converts a string into an RFC3339 DateTime object. |
Http response received.
Name | Type | Description |
---|---|---|
status_code | Integer | The status code returned by the server. |
reason_phrase | String | The reason phrase returned by the server. |
headers | Hash | Response headers. |
raw_body | String | Response body. |
request | HttpRequest | The request that resulted in this response. |
Represents a single Http Request.
Name | Type | Tag | Description |
---|---|---|---|
http_method | HttpMethodEnum | The HTTP method of the request. | |
query_url | String | The endpoint URL for the API request. | |
headers | Hash | Optional | Request headers. |
parameters | Hash | Optional | Request body. |
FAQs
Unknown package
We found that apimatic-ci-cd-test demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.