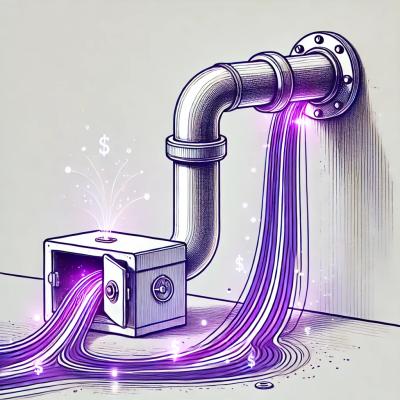
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Pretty-print your JSON in Ruby or JavaScript or Lua with more power than is provided by JSON.pretty_generate
(Ruby) or JSON.stringify
(JS). For example, like Ruby's pp
(pretty print), NeatJSON can keep objects on one line if they fit, but break them over multiple lines if needed.
Features:
42.0
instead of 42
).gem install neatjson
javascript/neatjson.js
npm install neatjson
Ruby:
require 'neatjson'
json = JSON.neat_generate( value, options )
JavaScript (web):
<script type="text/javascript" src="neatjson.js"></script>
<script type="text/javascript">
var json = neatJSON( value, options );
</script>
Node.js:
const { neatJSON } = require('neatjson');
var json = neatJSON( value, options );
Lua:
local neatJSON = require'neatjson'
local json = neatJSON(value, options)
The following are all in Ruby, but similar options apply in JavaScript and Lua.
require 'neatjson'
o = { b:42.005, a:[42,17], longer:true, str:"yes\nplease" }
puts JSON.neat_generate(o)
#=> {"b":42.005,"a":[42,17],"longer":true,"str":"yes\nplease"}
puts JSON.neat_generate(o, sort:true)
#=> {"a":[42,17],"b":42.005,"longer":true,"str":"yes\nplease"}
puts JSON.neat_generate(o,sort:true,padding:1,after_comma:1)
#=> { "a":[ 42, 17 ], "b":42.005, "longer":true, "str":"yes\nplease" }
puts JSON.neat_generate(o, sort:true, wrap:40)
#=> {
#=> "a":[42,17],
#=> "b":42.005,
#=> "longer":true,
#=> "str":"yes\nplease"
#=> }
puts JSON.neat_generate(o, sort:true, wrap:40, decimals:2)
#=> {
#=> "a":[42,17],
#=> "b":42.01,
#=> "longer":true,
#=> "str":"yes\nplease"
#=> }
puts JSON.neat_generate(o, sort:->(k){ k.length }, wrap:40, aligned:true)
#=> {
#=> "a" :[42,17],
#=> "b" :42.005,
#=> "str" :"yes\nplease",
#=> "longer":true
#=> }
puts JSON.neat_generate(o, sort:true, wrap:40, aligned:true, around_colon:1)
#=> {
#=> "a" : [42,17],
#=> "b" : 42.005,
#=> "longer" : true,
#=> "str" : "yes\nplease"
#=> }
puts JSON.neat_generate(o, sort:true, wrap:40, aligned:true, around_colon:1, short:true)
#=> {"a" : [42,17],
#=> "b" : 42.005,
#=> "longer" : true,
#=> "str" : "yes\nplease"}
a = [1,2,[3,4,[5]]]
puts JSON.neat_generate(a)
#=> [1,2,[3,4,[5]]]
puts JSON.pretty_generate(a) # oof!
#=> [
#=> 1,
#=> 2,
#=> [
#=> 3,
#=> 4,
#=> [
#=> 5
#=> ]
#=> ]
#=> ]
puts JSON.neat_generate( a, wrap:true, short:true )
#=> [1,
#=> 2,
#=> [3,
#=> 4,
#=> [5]]]
data = ["foo","bar",{dogs:42,piggies:{color:'pink', tasty:true},
barn:{jimmy:[1,2,3,4,5],jammy:3.141592653,hot:"pajammy"},cats:7}]
opts = { short:true, wrap:60, decimals:3, sort:true, aligned:true,
padding:1, after_comma:1, around_colon_n:1 }
puts JSON.neat_generate( data, opts )
#=> [ "foo",
#=> "bar",
#=> { "barn" : { "hot" : "pajammy",
#=> "jammy" : 3.142,
#=> "jimmy" : [ 1, 2, 3, 4, 5 ] },
#=> "cats" : 7,
#=> "dogs" : 42,
#=> "piggies" : { "color":"pink", "tasty":true } } ]
You may pass any of the following options to neat_generate
(Ruby) or neatJSON
(JavaScript/Lua). Note: option names with underscores below use camelCase in JavaScript and Lua. For example:
# Ruby
json = JSON.neat_generate my_value, array_padding:1, after_comma:1, before_colon_n:2
// JavaScript
var json = neatJSON( myValue, { arrayPadding:1, afterComma:1, beforeColonN:2 } );
-- Lua
local json = neatJSON( myValue, { arrayPadding=1, afterComma=1, beforeColonN=2 } )
wrap
— Maximum line width before wrapping. Use false
to never wrap, true
to always wrap. default:80
indent
— Whitespace used to indent each level when wrapping. default:" "
(two spaces)indent_last
— Indent the closing bracket/brace for arrays and objects? default:false
short
— Put opening brackets on the same line as the first value, closing brackets on the same line as the last? default:false
indent
and indent_last
options to be ignored, instead basing indentation on array and object padding.sort
— Sort objects' keys in alphabetical order (true
), or supply a lambda for custom sorting. default:false
sort
option, it will be passed three values: the (string) name of the key, the associated value, and the object being sorted, e.g. { sort:->(key,value,hash){ Float(value) rescue Float::MAX } }
aligned
— When wrapping objects, line up the colons (per object)? default:false
decimals
— Decimal precision for non-integer numbers; use false
to keep values precise. default:false
trim_trailing_zeros
— Remove extra zeros at the end of floats, e.g. 1.2000
becomes 1.2
. default:false
force_floats
— Force every integer value written to the file to be a float, e.g. 12
becomes 12.0
. default:false
force_floats_in
— Specify an array of object key names under which all integer values are treated as floats.
For example, serializing {a:[1, 2, {a:3, b:4}], c:{a:5, d:6}
with force_floats_in:['a']
would produce {"a":[1.0, 2.0, {"a":3.0, "b":4}], "c":{"a":5.0, "d":6}}
.array_padding
— Number of spaces to put inside brackets for arrays. default:0
object_padding
— Number of spaces to put inside braces for objects. default:0
padding
— Shorthand to set both array_padding
and object_padding
. default:0
before_comma
— Number of spaces to put before commas (for both arrays and objects). default:0
after_comma
— Number of spaces to put after commas (for both arrays and objects). default:0
around_comma
— Shorthand to set both before_comma
and after_comma
. default:0
before_colon_1
— Number of spaces before a colon when the object is on one line. default:0
after_colon_1
— Number of spaces after a colon when the object is on one line. default:0
before_colon_n
— Number of spaces before a colon when the object is on multiple lines. default:0
after_colon_n
— Number of spaces after a colon when the object is on multiple lines. default:0
before_colon
— Shorthand to set both before_colon_1
and before_colon_n
. default:0
after_colon
— Shorthand to set both after_colon_1
and after_colon_n
. default:0
around_colon
— Shorthand to set both before_colon
and after_colon
. default:0
lua
— (Lua only) Output a Lua table literal instead of JSON? default:false
emptyTablesAreObjects
— (Lua only) Should {}
in Lua become a JSON object ({}
) or JSON array ([]
)? default:false
(array)You may omit the 'value' and/or 'object' parameters in your sort
lambda if desired. For example:
# Ruby sorting examples
obj = {e:3, a:2, c:3, b:2, d:1, f:3}
JSON.neat_generate obj, sort:true # sort by key name
#=> {"a":2,"b":2,"c":3,"d":1,"e":3,"f":3}
JSON.neat_generate obj, sort:->(k){ k } # sort by key name (long way)
#=> {"a":2,"b":2,"c":3,"d":1,"e":3,"f":3}
JSON.neat_generate obj, sort:->(k,v){ [-v,k] } # sort by descending value, then by ascending key
#=> {"c":3,"e":3,"f":3,"a":2,"b":2,"d":1}
JSON.neat_generate obj, sort:->(k,v,h){ h.values.count(v) } # sort by count of keys with same value
#=> {"d":1,"a":2,"b":2,"e":3,"c":3,"f":3}
// JavaScript sorting examples
var obj = {e:3, a:2, c:3, b:2, d:1, f:3};
neatJSON( obj, {sort:true} ); // sort by key name
// {"a":2,"b":2,"c":3,"d":1,"e":3,"f":3}
neatJSON( obj, { sort:function(k){ return k }} ); // sort by key name (long way)
// {"a":2,"b":2,"c":3,"d":1,"e":3,"f":3}
neatJSON( obj, { sort:function(k,v){ return -v }} ); // sort by descending value
// {"e":3,"c":3,"f":3,"a":2,"b":2,"d":1}
var countByValue = {};
for (var k in obj) countByValue[obj[k]] = (countByValue[obj[k]]||0) + 1;
neatJSON( obj, { sort:function(k,v){ return countByValue[v] } } ); // sort by count of same value
// {"d":1,"a":2,"b":2,"e":3,"c":3,"f":3}
Note that the JavaScript and Lua versions of NeatJSON do not provide a mechanism for cascading sort in the same manner as Ruby.
NeatJSON is copyright ©2015–2022 by Gavin Kistner and is released under the MIT License. See the LICENSE.txt file for more details.
For bugs or feature requests please open issues on GitHub. For other communication you can email the author directly.
to_json
for their representation.v0.10.5 — November 17, 2022
#
get an invalid escape added (Ruby only)v0.10.4 — November 17, 2022
v0.10.2 — August 31, 2022
trim_trailing_zeros
.v0.10.1 — August 29, 2022
force_floats_in
was combined with wrapping.v0.10 — August 29, 2022
force_floats
and force_floats_in
to support serialization for non-standard parsers that differentiate between integers and floats.trim_trailing_zeros
option to convert the decimals
output from e.g. 5.40000
to 5.4
.v0.9 — July 29, 2019
9e9999
and -9e9999
"NaN"
v0.8.4 — May 3, 2018
v0.8.3 — February 20, 2017
v0.8.2 — December 16th, 2016
v0.8.1 — April 22nd, 2016
v0.8 — April 21st, 2016
sort
to take a lambda for customized sorting of object key/values.v0.7.2 — April 14th, 2016
Object
constructor (e.g. location
).v0.7.1 — April 6th, 2016
v0.7 — March 26th, 2016
indentLast
/indent_last
feature.v0.6.2 — February 8th, 2016
v0.6.1 — October 12th, 2015
v0.6 — April 26th, 2015
before_colon_1
and before_colon_n
to distinguish between single-line and multi-line objects.v0.5 — April 19th, 2015
decimals
option.neatJSON()
JavaScript available to Node.js as well as web browsers.v0.4 — April 18th, 2015
v0.3.2 — April 16th, 2015
v0.3.1 — April 16th, 2015
v0.3 — April 16th, 2015
short:true
and wrapping array values inside objects.v0.2 — April 16th, 2015
short:true
and wrapping values inside objects.v0.1 — April 15th, 2015
FAQs
Unknown package
We found that neatjson demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.